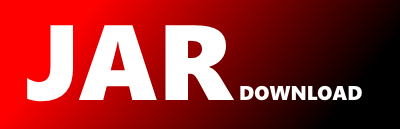
net.sf.ij_plugins.imageio.Validate Maven / Gradle / Ivy
Show all versions of ijp_imageio Show documentation
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package net.sf.ij_plugins.imageio;
/**
* A few methods from org.apache.commons.lang3.Validate, duplicated to reduce runtime dependencies.
*/
interface Validate {
/**
* Validate that the specified argument array is neither {@code null}
* nor a length of zero (no elements); otherwise throwing an exception
* with the specified message.
*
* Validate.notEmpty(myArray, "The array must not be empty");
*
* @param the array type
* @param array the array to check, validated not null by this method
* @param message the {@link String#format(String, Object...)} exception message if invalid, not null
* @param values the optional values for the formatted exception message, null array not recommended
* @return the validated array (never {@code null} method for chaining)
* @throws NullPointerException if the array is {@code null}
* @throws IllegalArgumentException if the array is empty
*/
static T[] notEmpty(final T[] array, final String message, final Object... values) {
if (array == null) {
throw new NullPointerException(String.format(message, values));
}
if (array.length == 0) {
throw new IllegalArgumentException(String.format(message, values));
}
return array;
}
/**
* Validate that the specified argument is not {@code null};
* otherwise throwing an exception.
*
* Validate.notNull(myObject, "The object must not be null");
*
* The message of the exception is "The validated object is
* null".
*
* @param the object type
* @param object the object to check
* @return the validated object (never {@code null} for method chaining)
* @throws NullPointerException if the object is {@code null}
* @see #notNull(Object, String, Object...)
*/
static T notNull(final T object) {
return notNull(object, "The validated object is null");
}
/**
* Validate that the specified argument is not {@code null};
* otherwise throwing an exception with the specified message.
*
* Validate.notNull(myObject, "The object must not be null");
*
* @param the object type
* @param object the object to check
* @param message the {@link String#format(String, Object...)} exception message if invalid, not null
* @param values the optional values for the formatted exception message
* @return the validated object (never {@code null} for method chaining)
* @throws NullPointerException if the object is {@code null}
* @see #notNull(Object)
*/
static T notNull(final T object, final String message, final Object... values) {
if (object == null) {
throw new NullPointerException(String.format(message, values));
}
return object;
}
/**
* Validate that the argument condition is {@code true}; otherwise
* throwing an exception with the specified message. This method is useful when
* validating according to an arbitrary boolean expression, such as validating a
* primitive number or using your own custom validation expression.
*
* Validate.isTrue(i >= min && i <= max, "The value must be between %d and %d", min, max);
* Validate.isTrue(myObject.isOk(), "The object is not okay");
*
* @param expression the boolean expression to check
* @param message the {@link String#format(String, Object...)} exception message if invalid, not null
* @param values the optional values for the formatted exception message, null array not recommended
* @throws IllegalArgumentException if expression is {@code false}
*/
static void isTrue(final boolean expression, final String message, final Object... values) {
if (!expression) {
throw new IllegalArgumentException(String.format(message, values));
}
}
}