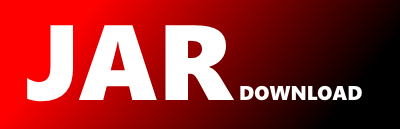
net.sf.ij_plugins.imageio.plugins.ImageIOOpenPlugin Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ijp_imageio Show documentation
Show all versions of ijp_imageio Show documentation
ijp-ImageIO enable reading and writing images using Java ImageIO codecs. The core ImageIO formats: JPEG, PNG, BMP, WBMP, and GIF. IJP-ImageIO is also using JAI codes adding support for TIFF, JPEG200, PNM, and PCX. TIFF supports reading and writing using various compression schemes: LZW, JPEG, ZIP, and Deflate. For more detailed information see IJP-ImageIO home page: https://github.com/ij-plugins/ijp-imageio/wiki.
/*
* Image/J Plugins
* Copyright (C) 2002-2016 Jarek Sacha
* Author's email: jpsacha at gmail.com
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*
* Latest release available at http://sourceforge.net/projects/ij-plugins/
*/
package net.sf.ij_plugins.imageio.plugins;
import ij.IJ;
import ij.ImagePlus;
import ij.ImageStack;
import ij.io.OpenDialog;
import ij.plugin.PlugIn;
import net.sf.ij_plugins.imageio.IJImageIO;
import net.sf.ij_plugins.imageio.impl.ImageFileChooserFactory;
import net.sf.ij_plugins.imageio.impl.OpenImageFileChooser;
import javax.swing.*;
import java.io.File;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
/**
* Opens file chooser dialog and reads images using {@link IJImageIO}.
*
* @author Jarek Sacha
*/
public class ImageIOOpenPlugin implements PlugIn {
// FIXME: keep in sync with current ImageJ directory
private static final String TITLE = "Image IO Open";
/**
* Argument passed to run
method to use open dialog with an image preview.
*/
public static final String ARG_IMAGE_PREVIEW = "preview";
private static OpenImageFileChooser jaiChooser;
/**
* Main processing method for the ImageIOOpenPlugin object. Type of the file dialog is
* determined by value of arg
. If it is equal "{@value #ARG_IMAGE_PREVIEW}" then
* file chooser with image preview will be used. By default standard Image/J's open dialog is
* used.
*
* @param arg Can be user to specify type of the open dialog.
*/
public void run(final String arg) {
IJ.showStatus("Starting \"" + TITLE + "\" plugins...");
final FilesAndPageIndex fpi = ARG_IMAGE_PREVIEW.equalsIgnoreCase(arg)
? selectFilesWithPreview()
: selectFile();
if (fpi.files.length < 1) {
return;
}
final boolean combineIntoStack = fpi.files.length > 1
&& IJ.showMessageWithCancel(TITLE,
"" + fpi.files.length + " files selected.\n"
+ "Should the images be combined into a stack?");
if (combineIntoStack) {
// Open images
// open(files, pageIndex, combineIntoStack);
final List imageList = new ArrayList<>();
for (final File file : fpi.files) {
final ImagePlus[] images = open(file, fpi.pageIndex);
imageList.addAll(Arrays.asList(images));
}
if (imageList.size() == 1) {
imageList.get(0).show();
} else if (imageList.size() > 1) {
// Attempt to combine
final ImagePlus stackImage = combineImages(imageList);
// Show
if (stackImage != null) {
stackImage.show();
} else {
if (imageList.size() > 1) {
IJ.showMessage(TITLE, "Unable to combine images into a stack.\n" +
"Loading each separately.");
}
for (final ImagePlus anImageList : imageList) {
anImageList.show();
}
}
}
} else {
for (final File file : fpi.files) {
final ImagePlus[] images = open(file, fpi.pageIndex);
for (final ImagePlus imp : images) {
imp.show();
}
}
}
IJ.showStatus("");
}
private FilesAndPageIndex selectFilesWithPreview() {
if (jaiChooser == null) {
jaiChooser = ImageFileChooserFactory.createJAIOpenChooser();
final String dirName = OpenDialog.getDefaultDirectory();
final File dirFile = new File(dirName != null ? dirName : ".");
jaiChooser.setCurrentDirectory(dirFile);
jaiChooser.setMultiSelectionEnabled(true);
}
final String dirName = OpenDialog.getDefaultDirectory();
final File dirFile = new File(dirName != null ? dirName : ".");
jaiChooser.setCurrentDirectory(dirFile);
if (jaiChooser.showOpenDialog(IJ.getInstance()) == JFileChooser.APPROVE_OPTION) {
OpenDialog.setDefaultDirectory(jaiChooser.getSelectedFile().getParentFile().getAbsolutePath());
return new FilesAndPageIndex(jaiChooser.getSelectedFiles(), jaiChooser.getPageIndex());
} else {
return new FilesAndPageIndex(new File[0], null);
}
}
/*
*
*/
private FilesAndPageIndex selectFile() {
final OpenDialog openDialog = new OpenDialog(TITLE, null);
if (openDialog.getFileName() == null) {
return new FilesAndPageIndex(new File[0], null);
}
final File[] files = new File[1];
files[0] = new File(openDialog.getDirectory(), openDialog.getFileName());
return new FilesAndPageIndex(files, null);
}
private ImagePlus[] open(final File file, int[] pageIndex) {
IJ.showStatus("Opening: " + file.getName());
try {
return IJImageIO.read(file, true, pageIndex);
} catch (final Exception ex) {
ex.printStackTrace();
String message = "Error opening file: " + file.getName() + ".\n\n";
message += (ex.getMessage() == null) ? ex.toString() : ex.getMessage();
IJ.showMessage(TITLE, message);
return new ImagePlus[0];
}
}
/**
* Attempts to combine images on the list into a stack. If successful return the combined image,
* otherwise return null. Images cannot be combined if they are of different types, different
* sizes, or have more then single slice.
*
* @param imageList List of images to combine into a stack.
* @return Combined image if successful, otherwise null.
*/
private static ImagePlus combineImages(final List imageList) {
// TODO: in unable to combine throw exception with error message, do not return null.
if (imageList == null || imageList.size() < 1) {
return null;
}
if (imageList.size() == 1) {
return imageList.get(0);
}
final ImagePlus firstImage = imageList.get(0);
if (firstImage.getStackSize() != 1) {
return null;
}
final int fileType = firstImage.getFileInfo().fileType;
final int w = firstImage.getWidth();
final int h = firstImage.getHeight();
final ImageStack stack = firstImage.getStack();
for (int i = 1; i < imageList.size(); ++i) {
final ImagePlus im = imageList.get(i);
if (im.getStackSize() != 1) {
return null;
}
if (fileType == im.getFileInfo().fileType
&& w == im.getWidth() && h == im.getHeight()) {
stack.addSlice(im.getTitle(), im.getProcessor().getPixels());
} else {
return null;
}
}
firstImage.setStack(firstImage.getTitle(), stack);
return firstImage;
}
private static class FilesAndPageIndex {
final File[] files;
final int[] pageIndex;
public FilesAndPageIndex(File[] files, int[] pageIndex) {
this.files = files;
this.pageIndex = pageIndex;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy