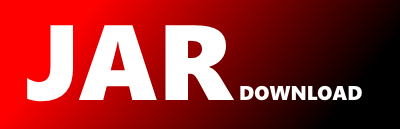
org.jgraph.graph.GraphModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ingeniasjgraphmod Show documentation
Show all versions of ingeniasjgraphmod Show documentation
A modified version of some JGraph files
The newest version!
/*
* @(#)GraphModel.java 1.0 03-JUL-04
*
* Copyright (c) 2001-2004 Gaudenz Alder
*
*/
package org.jgraph.graph;
import java.util.Iterator;
import java.util.Map;
import javax.swing.event.UndoableEditListener;
import javax.swing.undo.UndoableEdit;
import org.jgraph.event.GraphModelListener;
/**
* The interface that defines a suitable data model for a JGraph.
*
* @version 1.0 1/1/02
* @author Gaudenz Alder
*/
public interface GraphModel {
//
// Roots
//
/**
* Returns the number of roots in the model. Returns 0 if the model is
* empty.
*
* @return the number of roots in the model
*/
int getRootCount();
/**
* Returns the root at index index in the model. This should not
* return null if index is a valid index for the model (that is
* index >= 0 && index < getRootCount()).
*
* @return the root of at index index
*/
Object getRootAt(int index);
/**
* Returns the index of root
in the model. If root is
* null
, returns -1.
*
* @param root
* a root in the model, obtained from this data source
* @return the index of the root in the model, or -1 if the parent is
* null
*/
int getIndexOfRoot(Object root);
/**
* Returns true
if node
or one of its
* ancestors is in the model.
*
* @return true
if node
is in the model
*/
boolean contains(Object node);
/**
* Returns a AttributeMap
that represents the properties for
* the specified cell.
*
* @return properties of node
as a Map
*/
AttributeMap getAttributes(Object node);
/**
* Returns the user object for the specified cell.
*
* @return userobject of node
*/
Object getValue(Object node);
//
// Graph Structure
//
/**
* Returns the source of edge
. edge must be an
* object previously obtained from this data source.
*
* @return Object
that represents the source of edge
*/
Object getSource(Object edge);
/**
* Returns the target of edge
. edge must be an
* object previously obtained from this data source.
*
* @return Object
that represents the target of edge
*/
Object getTarget(Object edge);
/**
* Returns true
if port
is a valid source for
* edge
. edge and port must be objects
* previously obtained from this data source.
*
* @return true
if port
is a valid source for
* edge
.
*/
boolean acceptsSource(Object edge, Object port);
/**
* Returns true
if port
is a valid target for
* edge
. edge and port must be objects
* previously obtained from this data source.
*
* @return true
if port
is a valid target for
* edge
.
*/
boolean acceptsTarget(Object edge, Object port);
/**
* Returns an iterator of the edges connected to port
.
* port must be a object previously obtained from this data source.
* This method never returns null.
*
* @param port
* a port in the graph, obtained from this data source
* @return Iterator
that represents the connected edges
*/
Iterator edges(Object port);
/**
* Returns true
if edge
is a valid edge.
*
* @return true
if edge
is a valid edge.
*/
boolean isEdge(Object edge);
/**
* Returns true
if port
is a valid port,
* possibly supporting edge connection.
*
* @return true
if port
is a valid port.
*/
boolean isPort(Object port);
//
// Group structure
//
/**
* Returns the parent of child in the model. child must be a
* node previously obtained from this data source. This returns null if
* child is a root in the model.
*
* @param child
* a node in the graph, obtained from this data source
* @return the parent of child
*/
Object getParent(Object child);
/**
* Returns the index of child in parent. If either the parent or child is
* null
, returns -1.
*
* @param parent
* a note in the tree, obtained from this data source
* @param child
* the node we are interested in
* @return the index of the child in the parent, or -1 if either the parent
* or the child is null
*/
int getIndexOfChild(Object parent, Object child);
/**
* Returns the child of parent at index index in the
* parent's child array. parent must be a node previously obtained
* from this data source. This should not return null if index is a
* valid index for parent (that is index >= 0 && index
* < getChildCount( parent )).
*
* @param parent
* a node in the tree, obtained from this data source
* @return the child of parent at index index
*/
Object getChild(Object parent, int index);
/**
* Returns the number of children of parent . Returns 0 if the node
* is a leaf or if it has no children. parent must be a node
* previously obtained from this data source.
*
* @param parent
* a node in the tree, obtained from this data source
* @return the number of children of the node parent
*/
int getChildCount(Object parent);
/**
* Returns whether the specified node is a leaf node. The way the test is
* performed depends on the askAllowsChildren
setting.
*
* @param node
* the node to check
* @return true if the node is a leaf node
*/
boolean isLeaf(Object node);
//
// Change Support
//
/**
* Inserts the cells
and connections into the model, and
* passes attributes
to the views. Notifies the model- and
* undo listeners of the change.
*/
void insert(Object[] roots, Map attributes, ConnectionSet cs, ParentMap pm,
UndoableEdit[] e);
/**
* Removes cells
from the model. Notifies the model- and undo
* listeners of the change.
*/
void remove(Object[] roots);
/**
* Applies the propertyMap
and the connection changes to the
* model. The initial edits
that triggered the call are
* considered to be part of this transaction. Notifies the model- and undo
* listeners of the change. Note: If only
* edits
is non-null, the edits are directly passed to the
* UndoableEditListeners.
*/
void edit(Map attributes, ConnectionSet cs, ParentMap pm, UndoableEdit[] e);
/**
* Indicates the start of one level of an executable change
*/
public void beginUpdate();
/**
* Indicates the end of the current level of an executable change
*/
public void endUpdate();
/**
* Executes the specified executable change on this graph model
* @param change the change to be executed
*/
public void execute(ExecutableChange change);
/**
* Returns a map of (cell, clone)-pairs for all cells
and
* their children. Special care should be taken to replace references
* between cells.
*/
Map cloneCells(Object[] cells);
/**
* Messaged when the value of the cell has changed, eg from within the edit
* method.
*/
Object valueForCellChanged(Object cell, Object newValue);
//
// Layering
//
/**
* Sends cells
to back.
*/
void toBack(Object[] cells);
/**
* Brings cells
to front.
*/
void toFront(Object[] cells);
//
// Listeners
//
/**
* Adds a listener for the GraphModelEvent posted after the model changes.
*/
void addGraphModelListener(GraphModelListener l);
/**
* Removes a listener previously added with addGraphModelListener() .
*/
void removeGraphModelListener(GraphModelListener l);
/**
* Adds an undo listener for notification of any changes. Undo/Redo
* operations performed on the UndoableEdit
will cause the
* appropriate ModelEvent to be fired to keep the view(s) in sync with the
* model.
*/
void addUndoableEditListener(UndoableEditListener listener);
/**
* Removes an undo listener.
*/
void removeUndoableEditListener(UndoableEditListener listener);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy