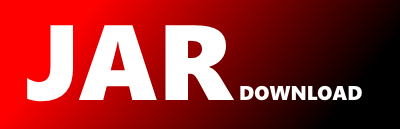
org.jgraph.graph.PortRenderer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ingeniasjgraphmod Show documentation
Show all versions of ingeniasjgraphmod Show documentation
A modified version of some JGraph files
The newest version!
/*
* @(#)PortRenderer.java 1.0 03-JUL-04
*
* Copyright (c) 2001-2004 Gaudenz Alder
*
*/
package org.jgraph.graph;
import java.awt.Color;
import java.awt.Component;
import java.awt.Dimension;
import java.awt.Graphics;
import java.awt.Rectangle;
import java.io.Serializable;
import javax.swing.JComponent;
import javax.swing.UIManager;
import org.jgraph.JGraph;
/**
* This renderer displays entries that implement the CellView interface and
* supports the following attributes:
* GraphConstants.OFFSET GraphConstants.ABSOLUTE convertValueToString
.
*
* @param graph
* the graph that that defines the rendering context.
* @param view
* the cell view that should be rendered.
* @param sel
* whether the object is selected.
* @param focus
* whether the object has the focus.
* @param preview
* whether we are drawing a preview.
* @return the component used to render the value.
*/
public Component getRendererComponent(JGraph graph, CellView view,
boolean sel, boolean focus, boolean preview) {
// Check type
if (view instanceof PortView && graph != null) {
graphBackground = graph.getBackground();
this.view = (PortView) view;
this.hasFocus = focus;
this.selected = sel;
this.preview = preview;
this.xorEnabled = graph.isXorEnabled();
return this;
}
return null;
}
/**
* Paint the renderer. Overrides superclass paint to add specific painting.
* Note: The preview flag is interpreted as "highlight" in this context.
* (This is used to highlight the port if the mouse is over it.)
*/
public void paint(Graphics g) {
Dimension d = getSize();
if (xorEnabled) {
g.setColor(graphBackground);
g.setXORMode(graphBackground);
}
super.paint(g);
if (preview) {
g.fill3DRect(0, 0, d.width, d.height, true);
} else {
g.fillRect(0, 0, d.width, d.height);
}
boolean offset = (GraphConstants.getOffset(view.getAllAttributes()) != null);
g.setColor(getForeground());
if (!offset)
g.fillRect(1, 1, d.width - 2, d.height - 2);
else if (!preview)
g.drawRect(1, 1, d.width - 3, d.height - 3);
}
/**
* Overridden for performance reasons. See the Implementation Note for more information.
*/
public void validate() {
}
/**
* Overridden for performance reasons. See the Implementation Note for more information.
*/
public void revalidate() {
}
/**
* Overridden for performance reasons. See the Implementation Note for more information.
*/
public void repaint(long tm, int x, int y, int width, int height) {
}
/**
* Overridden for performance reasons. See the Implementation Note for more information.
*/
public void repaint(Rectangle r) {
}
/**
* Overridden for performance reasons. See the Implementation Note for more information.
*/
protected void firePropertyChange(String propertyName, Object oldValue,
Object newValue) {
// Strings get interned...
if (propertyName == "text")
super.firePropertyChange(propertyName, oldValue, newValue);
}
/**
* Overridden for performance reasons. See the Implementation Note for more information.
*/
public void firePropertyChange(String propertyName, byte oldValue,
byte newValue) {
}
/**
* Overridden for performance reasons. See the Implementation Note for more information.
*/
public void firePropertyChange(String propertyName, char oldValue,
char newValue) {
}
/**
* Overridden for performance reasons. See the Implementation Note for more information.
*/
public void firePropertyChange(String propertyName, short oldValue,
short newValue) {
}
/**
* Overridden for performance reasons. See the Implementation Note for more information.
*/
public void firePropertyChange(String propertyName, int oldValue,
int newValue) {
}
/**
* Overridden for performance reasons. See the Implementation Note for more information.
*/
public void firePropertyChange(String propertyName, long oldValue,
long newValue) {
}
/**
* Overridden for performance reasons. See the Implementation Note for more information.
*/
public void firePropertyChange(String propertyName, float oldValue,
float newValue) {
}
/**
* Overridden for performance reasons. See the Implementation Note for more information.
*/
public void firePropertyChange(String propertyName, double oldValue,
double newValue) {
}
/**
* Overridden for performance reasons. See the Implementation Note for more information.
*/
public void firePropertyChange(String propertyName, boolean oldValue,
boolean newValue) {
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy