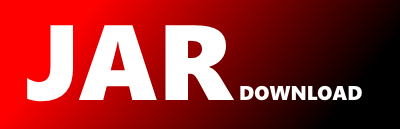
ingenias.editor.DraggableTabbedPane Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ingened Show documentation
Show all versions of ingened Show documentation
Meta-language editor built over INGENME. It is the most basic editor to be used in the rest of projects
package ingenias.editor;
import ingenias.editor.ButtonTabComponent;
import java.awt.AWTException;
import java.awt.BorderLayout;
import java.awt.Color;
import java.awt.Component;
import java.awt.Dimension;
import java.awt.DisplayMode;
import java.awt.Graphics;
import java.awt.GraphicsDevice;
import java.awt.GraphicsEnvironment;
import java.awt.Image;
import java.awt.Point;
import java.awt.Rectangle;
import java.awt.Robot;
import java.awt.Toolkit;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import java.awt.event.MouseMotionAdapter;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.awt.image.BufferedImage;
import javax.swing.Icon;
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JTabbedPane;
//Code partially reused from
// http://stackoverflow.com/questions/60269/how-to-implement-draggable-tab-using-java-swing
// hack to simulate full screen drawing
//http://weblogs.java.net/blog/joshy/archive/2003/12/swing_hack_7_le.html
//drop on new window code is original
public class DraggableTabbedPane extends JTabbedPaneWithCloseIcons {
private static GraphicsDevice device;
private static DisplayMode original_mode;
private static Dimension size;
private static BufferedImage screen;
private boolean dragging = false;
private Image tabImage = null;
private Point currentMouseLocation = null;
private int draggedTabIndex = 0;
private JFrame falseForeground;
private BufferedImage toPaintOver;
private int oldx;
private int oldy;
public DraggableTabbedPane() {
super();
addMouseMotionListener(new MouseMotionAdapter() {
public void mouseDragged(MouseEvent e) {
if(!dragging) {
// Gets the tab index based on the mouse position
int tabNumber = getUI().tabForCoordinate(DraggableTabbedPane.this, e.getX(), e.getY());
if(tabNumber >= 0) {
draggedTabIndex = tabNumber;
Rectangle bounds = getComponentAt(draggedTabIndex).getBounds();
//getUI().getTabBounds(DraggableTabbedPane.this, tabNumber);
// Paint the tabbed pane to a buffer
Image totalImage = new BufferedImage(getWidth(), getHeight(), BufferedImage.TYPE_INT_ARGB);
Graphics totalGraphics = totalImage.getGraphics();
totalGraphics.setClip(bounds);
// Don't be double buffered when painting to a static image.
setDoubleBuffered(false);
paint(totalGraphics);
// Paint just the dragged tab to the buffer
tabImage = new BufferedImage(bounds.width, bounds.height, BufferedImage.TYPE_INT_ARGB);
Graphics graphics = tabImage.getGraphics();
graphics.drawImage(totalImage, 0, 0,
bounds.width, bounds.height, bounds.x, bounds.y,
bounds.x + bounds.width, bounds.y+bounds.height,
DraggableTabbedPane.this);
dragging = true;
/*try {
captureScreen();
} catch (AWTException e1) {
// TODO Auto-generated catch block
e1.printStackTrace();
}*/
repaint();
}
} else {
currentMouseLocation = e.getPoint();
// Need to repaint
repaint();
}
super.mouseDragged(e);
}
});
addMouseListener(new MouseAdapter() {
private Icon draggedIcon;
public void mouseReleased(MouseEvent e) {
if(dragging) {
int tabNumber = getUI().tabForCoordinate(DraggableTabbedPane.this, e.getX(), 10);
final Component comp = getComponentAt(draggedTabIndex);
final String title = getTitleAt(draggedTabIndex);
if (tabNumber>=0)
draggedIcon=getIconAt(tabNumber);
removeTabAt(draggedTabIndex);
if(tabNumber >= 0 &&
e.getY()=0 &&
e.getX()>=0 && e.getX()=0)
setSelectedIndex(tabNumber);
}
}
public void addTabWithCloseIcon(String title, Component component) {
super.addTab(title,component);
this.setTabComponentAt(this.getTabCount()-1, new ButtonTabComponent((JTabbedPane)this,null));
}
public static void main(String[] args) {
JFrame test = new JFrame("Tab test");
test.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
test.setSize(400, 400);
GraphicsEnvironment ge =
GraphicsEnvironment.getLocalGraphicsEnvironment();
GraphicsDevice gd = ge.getDefaultScreenDevice();
//If translucent windows aren't supported, exit.
/* if (!gd.isWindowTranslucencySupported(TRANSLUCENT)) {
System.err.println(
"Translucency is not supported");
System.exit(0);
}*/
DraggableTabbedPane tabs = new DraggableTabbedPane();
tabs.addTab("One", new JButton("One"));
tabs.addTab("Two", new JButton("Two"));
tabs.addTab("Three", new JButton("Three"));
tabs.addTab("Four", new JButton("Four"));
test.add(tabs);
test.setVisible(true);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy