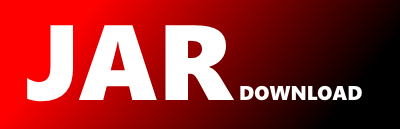
ingenias.editor.persistence.RelationshipSave Maven / Gradle / Ivy
/**
* Copyright (C) 2010 Jorge J. Gomez-Sanz over original code from Ruben Fuentes
*
*
*
* This file is part of the INGENME tool. INGENME is an open source meta-editor
* which produces customized editors for user-defined modeling languages
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation version 3 of the License
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
* You should have received a copy of the GNU General Public License
* along with this program. If not, see
**/
package ingenias.editor.persistence;
import java.lang.reflect.*;
import javax.swing.tree.*;
import org.apache.xerces.parsers.DOMParser;
import org.xml.sax.InputSource;
import java.awt.Color;
import java.awt.Point;
import java.awt.Rectangle;
import java.io.OutputStreamWriter;
import java.io.*;
import javax.swing.tree.DefaultMutableTreeNode;
import java.util.*;
import java.util.Map;
import java.util.Hashtable;
import java.util.ArrayList;
import javax.xml.parsers.*;
import org.jgraph.JGraph;
import org.jgraph.graph.*;
import org.w3c.dom.*;
import org.w3c.dom.Document;
import org.w3c.dom.DOMImplementation;/**
* Copyright (C) 2010 Jorge J. Gomez-Sanz over original code from Ruben Fuentes
*
* Modifications over original code from jgraph.sourceforge.net
*
* This file is part of the INGENME tool. INGENME is an open source meta-editor
* which produces customized editors for user-defined modeling languages
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation version 3 of the License
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
* You should have received a copy of the GNU General Public License
* along with this program. If not, see
**/
import ingenias.editor.entities.*;
import ingenias.exception.*;
import ingenias.editor.cell.*;
import ingenias.editor.*;
public class RelationshipSave extends RelationshipSaveAbs{
protected void saveRole(ingenias.editor.entities.RoleEntity en,
OutputStreamWriter os) throws
IOException {
// os.write("\n");
Enumeration enumeration = null;
if (en.getClass().equals(ingenias.editor.entities.ContainssourceRole.class)){
ingenias.editor.entities.ContainssourceRole nen=(ingenias.editor.entities.ContainssourceRole)en;
}
if (en.getClass().equals(ingenias.editor.entities.ContainstargetRole.class)){
ingenias.editor.entities.ContainstargetRole nen=(ingenias.editor.entities.ContainstargetRole)en;
}
if (en.getClass().equals(ingenias.editor.entities.IsDocumentedBysourceRole.class)){
ingenias.editor.entities.IsDocumentedBysourceRole nen=(ingenias.editor.entities.IsDocumentedBysourceRole)en;
}
if (en.getClass().equals(ingenias.editor.entities.IsDocumentedBytargetRole.class)){
ingenias.editor.entities.IsDocumentedBytargetRole nen=(ingenias.editor.entities.IsDocumentedBytargetRole)en;
}
if (en.getClass().equals(ingenias.editor.entities.PropsOrderedAssourceRole.class)){
ingenias.editor.entities.PropsOrderedAssourceRole nen=(ingenias.editor.entities.PropsOrderedAssourceRole)en;
}
if (en.getClass().equals(ingenias.editor.entities.PropsOrderedAstargetRole.class)){
ingenias.editor.entities.PropsOrderedAstargetRole nen=(ingenias.editor.entities.PropsOrderedAstargetRole)en;
}
if (en.getClass().equals(ingenias.editor.entities.VisualizedAssourceRole.class)){
ingenias.editor.entities.VisualizedAssourceRole nen=(ingenias.editor.entities.VisualizedAssourceRole)en;
}
if (en.getClass().equals(ingenias.editor.entities.VisualizedAstargetRole.class)){
ingenias.editor.entities.VisualizedAstargetRole nen=(ingenias.editor.entities.VisualizedAstargetRole)en;
}
if (en.getClass().equals(ingenias.editor.entities.HassourceRole.class)){
ingenias.editor.entities.HassourceRole nen=(ingenias.editor.entities.HassourceRole)en;
}
if (en.getClass().equals(ingenias.editor.entities.HasMOtargetRole.class)){
ingenias.editor.entities.HasMOtargetRole nen=(ingenias.editor.entities.HasMOtargetRole)en;
}
if (en.getClass().equals(ingenias.editor.entities.AssociationEndsourceRole.class)){
ingenias.editor.entities.AssociationEndsourceRole nen=(ingenias.editor.entities.AssociationEndsourceRole)en;
}
if (en.getClass().equals(ingenias.editor.entities.AssociationEndtargetRole.class)){
ingenias.editor.entities.AssociationEndtargetRole nen=(ingenias.editor.entities.AssociationEndtargetRole)en;
}
if (en.getClass().equals(ingenias.editor.entities.PlayedBysourceRole.class)){
ingenias.editor.entities.PlayedBysourceRole nen=(ingenias.editor.entities.PlayedBysourceRole)en;
}
if (en.getClass().equals(ingenias.editor.entities.PlayedBytargetRole.class)){
ingenias.editor.entities.PlayedBytargetRole nen=(ingenias.editor.entities.PlayedBytargetRole)en;
}
if (en.getClass().equals(ingenias.editor.entities.BinaryRelsourceRole.class)){
ingenias.editor.entities.BinaryRelsourceRole nen=(ingenias.editor.entities.BinaryRelsourceRole)en;
}
if (en.getClass().equals(ingenias.editor.entities.BinaryReltargetRole.class)){
ingenias.editor.entities.BinaryReltargetRole nen=(ingenias.editor.entities.BinaryReltargetRole)en;
}
if (en.getClass().equals(ingenias.editor.entities.InheritsRsourceRole.class)){
ingenias.editor.entities.InheritsRsourceRole nen=(ingenias.editor.entities.InheritsRsourceRole)en;
}
if (en.getClass().equals(ingenias.editor.entities.InheritsRtargetRole.class)){
ingenias.editor.entities.InheritsRtargetRole nen=(ingenias.editor.entities.InheritsRtargetRole)en;
}
if (en.getClass().equals(ingenias.editor.entities.HasCommentsourceRole.class)){
ingenias.editor.entities.HasCommentsourceRole nen=(ingenias.editor.entities.HasCommentsourceRole)en;
}
if (en.getClass().equals(ingenias.editor.entities.HasCommenttargetRole.class)){
ingenias.editor.entities.HasCommenttargetRole nen=(ingenias.editor.entities.HasCommenttargetRole)en;
}
if (en.getClass().equals(ingenias.editor.entities.InheritsOsourceRole.class)){
ingenias.editor.entities.InheritsOsourceRole nen=(ingenias.editor.entities.InheritsOsourceRole)en;
}
if (en.getClass().equals(ingenias.editor.entities.InheritsOtargetRole.class)){
ingenias.editor.entities.InheritsOtargetRole nen=(ingenias.editor.entities.InheritsOtargetRole)en;
}
if (en.getClass().equals(ingenias.editor.entities.HassourceRole.class)){
ingenias.editor.entities.HassourceRole nen=(ingenias.editor.entities.HassourceRole)en;
}
if (en.getClass().equals(ingenias.editor.entities.HastargetRole.class)){
ingenias.editor.entities.HastargetRole nen=(ingenias.editor.entities.HastargetRole)en;
}
Hashtable ht = new Hashtable();
en.toMap(ht);
ObjectSave.saveMap(ht, os);
// os.write(" \n");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy