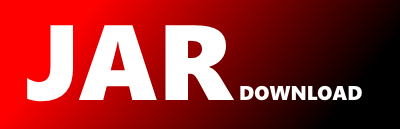
net.sf.itcb.common.client.aop.ProviderInterceptor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of itcb-common-client Show documentation
Show all versions of itcb-common-client Show documentation
This module is the common client module for calling webservices (server module). It defines all generic treatements that allows calling webservices
package net.sf.itcb.common.client.aop;
import java.io.IOException;
import javax.annotation.Resource;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.JAXBException;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.namespace.QName;
import javax.xml.transform.Source;
import javax.xml.transform.TransformerException;
import net.sf.itcb.common.client.exceptions.ClientItcbException;
import net.sf.itcb.common.client.exceptions.ClientItcbExceptionMappingErrors;
import net.sf.itcb.common.client.marshaller.ItcbJaxb2Marshaller;
import org.aopalliance.intercept.MethodInterceptor;
import org.aopalliance.intercept.MethodInvocation;
import org.springframework.beans.factory.annotation.Configurable;
import org.springframework.context.i18n.LocaleContextHolder;
import org.springframework.ws.client.core.SourceExtractor;
import org.springframework.ws.client.core.support.WebServiceGatewaySupport;
import org.springframework.ws.soap.client.SoapFaultClientException;
import org.springframework.xml.transform.StringResult;
import org.springframework.xml.transform.StringSource;
/**
* This interceptor allows to use generic client in order to call a remote provider webservice.
* It can manage each interface which respects those rules :
*
* - Only one parameter *Request generated by JAXB from an element or from a complexType defined in a WSDL. This object contains all information needed by the service
* - A return object *Response generated by JAXB from an element or from a complexType defined in a WSDL
*
* If it doesn't match, a {@link net.sf.itcb.common.client.exceptions.ClientItcbException} is thrown.
* If requests and responses are built from a complexType, so no {@link XmlRootElement} is defined in JAXB classes.
* For this context, you have two choices :
*
* - Choice 1 : to define the {@link QName} that has to be used for requests.
* - Choice 2 : to let this class generate the {@link QName} using the class as localPart.
*
* This {@link QName} is used for main xml tag in SOAP body.
* This {@link QName} has to match the declaration in your provider wsdl.
* For example
*
* - When the wsdl part name doesn't match to the name of the wsdl message, you have to use Option 1 :
*
{@code
*
*
*
*
*
*
*
* }
* When the wsdl part name matches to the name of the wsdl message, you should use Option 2 :
* {@code
*
*
*
*
*
*
*
* }
*
*
* You have to use {@link ItcbJaxb2Marshaller} in order this class can retrieve the original marshaller and unmarshaller which allow to unmarshall with a specified returned type
*
* @author Pierre Le Roux
*/
@Configurable(preConstruction=true)
@SuppressWarnings({ "unchecked", "rawtypes" })
public class ProviderInterceptor extends WebServiceGatewaySupport implements MethodInterceptor {
@Resource(name="common-clientResources")
protected org.springframework.context.MessageSource messageSource;
protected QName xmlRootElementTag;
public void setXmlRootElementTag(QName xmlRootElementTag) {
this.xmlRootElementTag=xmlRootElementTag;
}
public Object invoke(MethodInvocation arg0) throws Throwable {
if(arg0.getArguments().length != 1) {
throw new ClientItcbException(ClientItcbExceptionMappingErrors.COMMON_CLIENT_NOT_CORRECT_INTERFACE, messageSource.getMessage("common-client.exception.not_correct_interface", new Object[] {arg0.getMethod()}, LocaleContextHolder.getLocale()));
}
try {
StringResult stringResult= new StringResult();
Object requestParameters= arg0.getArguments()[0];
Class> requestParametersClass = requestParameters.getClass();
if(requestParametersClass.isAnnotationPresent(XmlRootElement.class)) {
((ItcbJaxb2Marshaller)getWebServiceTemplate().getMarshaller()).getMarshaller().marshal(requestParameters, stringResult);
}
else {
if(xmlRootElementTag!= null) {
((ItcbJaxb2Marshaller)getWebServiceTemplate().getMarshaller()).getMarshaller().marshal(new JAXBElement(xmlRootElementTag, requestParametersClass, requestParameters), stringResult);
}
else {
((ItcbJaxb2Marshaller)getWebServiceTemplate().getMarshaller()).getMarshaller().marshal(new JAXBElement(new QName(requestParametersClass.getSimpleName()), requestParametersClass, requestParameters), stringResult);
}
}
return getWebServiceTemplate().sendSourceAndReceive(new StringSource(stringResult.getWriter().toString()), new ItcbResponseExtractor(arg0.getMethod().getReturnType()));
}
catch (SoapFaultClientException sfce) {
logger.error(sfce.getFaultCode(), sfce);
throw new ClientItcbException(ClientItcbExceptionMappingErrors.COMMON_CLIENT_TECHNICAL_ERROR, messageSource.getMessage("common-client.exception.technical_error", new Object[] {arg0.getMethod(), getWebServiceTemplate().getDestinationProvider().getDestination(), sfce.getMessage()}, LocaleContextHolder.getLocale()));
}
catch(java.lang.IllegalArgumentException iae) {
logger.error(iae.getMessage(), iae);
throw new ClientItcbException(ClientItcbExceptionMappingErrors.COMMON_CLIENT_TECHNICAL_ERROR, messageSource.getMessage("common-client.exception.technical_error", new Object[] {arg0.getMethod(), getWebServiceTemplate().getDestinationProvider().getDestination(), iae.getMessage()}, LocaleContextHolder.getLocale()));
}
}
/**
* This class is an extractor for the response
* It allows to unmarshall the response using a specified returned type which is the expected type for the method intercepted by the MethodInterceptor
* @author Pierre Le Roux
* @param
*/
public class ItcbResponseExtractor implements SourceExtractor{
private Class clazz;
public ItcbResponseExtractor(Class clazz) {
super();
this.clazz=clazz;
}
@Override
public T extractData(Source src) throws IOException,
TransformerException {
try {
Object object = ((ItcbJaxb2Marshaller)getWebServiceTemplate().getUnmarshaller()).getUnmarshaller().unmarshal(src, clazz);
if(object instanceof JAXBElement) {
return (T)((JAXBElement)object).getValue();
}
else {
return (T)object;
}
} catch (JAXBException e) {
throw new TransformerException(e);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy