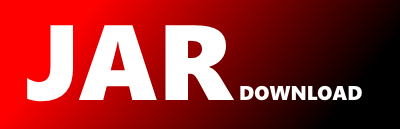
net.sf.itcb.common.client.aop.ClientInterceptor Maven / Gradle / Ivy
package net.sf.itcb.common.client.aop;
import net.sf.itcb.common.business.core.ItcbApplicationContextHolder;
import net.sf.itcb.common.client.exceptions.ClientItcbException;
import net.sf.itcb.common.client.exceptions.ClientItcbExceptionMappingErrors;
import net.sf.itcb.common.client.security.provider.SecurementCredentialsProvider;
import org.aopalliance.intercept.MethodInterceptor;
import org.aopalliance.intercept.MethodInvocation;
import org.springframework.context.MessageSource;
import org.springframework.context.i18n.LocaleContextHolder;
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.ws.client.core.support.WebServiceGatewaySupport;
import org.springframework.ws.soap.client.SoapFaultClientException;
import org.springframework.ws.soap.security.wss4j.Wss4jSecurityInterceptor;
/**
* This interceptor allows to use generic client in order to call a spring webservice.
* It can manage each interface which respects those rules :
*
* - Only one parameter *Request generated by JAXB using an XSD file. This object contains all information needed by the service
* - A return object *Response generated by JAXB using an XSD file
*
* If it doesn't match, a {@link net.sf.itcb.common.client.exceptions.ClientItcbException} is thrown with {@link net.sf.itcb.common.client.exceptions.ClientExceptionMappingErrors#}.
*
* If a {@link org.springframework.ws.soap.security.wss4j.Wss4jSecurityInterceptor} interceptor is declared in the webServiceTemplate property,
* then the securementCredentialsProvider (@see net.sf.itcb.common.client.security.provider.SecurementCredentialsProvider.SecurementCredentialsProvider} property has to be defined too.
* If not, a {@link net.sf.itcb.common.client.exceptions.ClientItcbException.ClientItcbException} is thrown with {@link net.sf.itcb.common.client.exceptions.ClientExceptionMappingErrors#COMMON_CLIENT_NOT_SET_SECUREMENT_PROVIDER}.
*
* @author Pierre Le Roux
*/
public class ClientInterceptor extends WebServiceGatewaySupport implements MethodInterceptor {
protected MessageSource messageSource = ItcbApplicationContextHolder.getContext().getBean("common-clientResources", MessageSource.class);
private SecurementCredentialsProvider securementCredentialsProvider;
public void setSecurementCredentialsProvider(SecurementCredentialsProvider securementCredentialsProvider) {
this.securementCredentialsProvider= securementCredentialsProvider;
}
public Object invoke(MethodInvocation arg0) throws Throwable {
for (int i = 0; i < getWebServiceTemplate().getInterceptors().length; i++) {
if(getWebServiceTemplate().getInterceptors()[i] instanceof Wss4jSecurityInterceptor) {
if(securementCredentialsProvider == null) {
throw new ClientItcbException(ClientItcbExceptionMappingErrors.COMMON_CLIENT_NOT_SET_SECUREMENT_PROVIDER, messageSource.getMessage("common-client.exception.not_set_securement_provider", new Object[] {arg0.getMethod()}, LocaleContextHolder.getLocale()));
}
UserDetails user = securementCredentialsProvider.getUserCredentials();
Wss4jSecurityInterceptor securityInterceptor = (Wss4jSecurityInterceptor)getWebServiceTemplate().getInterceptors()[i];
if(user!= null) {
securityInterceptor.setSecurementUsername(user.getUsername());
securityInterceptor.setSecurementPassword(user.getPassword());
}
}
};
if(arg0.getArguments().length != 1) {
throw new ClientItcbException(ClientItcbExceptionMappingErrors.COMMON_CLIENT_NOT_CORRECT_INTERFACE, messageSource.getMessage("common-client.exception.not_correct_interface", new Object[] {arg0.getMethod()}, LocaleContextHolder.getLocale()));
}
try {
return getWebServiceTemplate().marshalSendAndReceive(arg0.getArguments()[0]);
}
catch (SoapFaultClientException sfce) {
logger.error(sfce.getFaultCode(), sfce);
throw new ClientItcbException(ClientItcbExceptionMappingErrors.COMMON_CLIENT_TECHNICAL_ERROR, messageSource.getMessage("common-client.exception.technical_error", new Object[] {arg0.getMethod(), getWebServiceTemplate().getDestinationProvider().getDestination(), sfce.getMessage()}, LocaleContextHolder.getLocale()));
}
catch(java.lang.IllegalArgumentException iae) {
logger.error(iae.getMessage(), iae);
throw new ClientItcbException(ClientItcbExceptionMappingErrors.COMMON_CLIENT_TECHNICAL_ERROR, messageSource.getMessage("common-client.exception.technical_error", new Object[] {arg0.getMethod(), getWebServiceTemplate().getDestinationProvider().getDestination(), iae.getMessage()}, LocaleContextHolder.getLocale()));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy