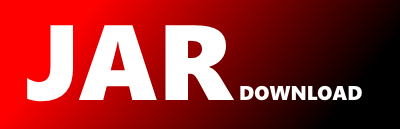
net.sf.jabb.dstream.StreamDataSupplierWithIdAndEnqueuedTimeRange Maven / Gradle / Ivy
/**
*
*/
package net.sf.jabb.dstream;
import java.time.Instant;
import java.util.function.Function;
import net.sf.jabb.dstream.ex.DataStreamInfrastructureException;
/**
* Data structure for a StreamDataSupplier, an ID, a from enqueued time, and a to enqueued time.
* The the from and to enqueued time can be inclusive or exclusive, depending on the upper level application.
* @author James Hu
*
* @param type of the message object
*
*/
public class StreamDataSupplierWithIdAndEnqueuedTimeRange extends SimpleStreamDataSupplierWithId implements StreamDataSupplierWithIdAndRange{
protected Instant fromEnqueuedTime;
protected Instant toEnqueuedTime;
public StreamDataSupplierWithIdAndEnqueuedTimeRange(){
super();
}
public StreamDataSupplierWithIdAndEnqueuedTimeRange(String id, StreamDataSupplier supplier){
super(id, supplier);
}
public StreamDataSupplierWithIdAndEnqueuedTimeRange(String id, StreamDataSupplier supplier, Instant fromEnqueuedTime, Instant toEnqueuedTime){
super(id, supplier);
this.fromEnqueuedTime = fromEnqueuedTime;
this.toEnqueuedTime = toEnqueuedTime;
}
@Override
public ReceiveStatus receiveInRange(Function receiver, String startPosition, String endPosition) throws DataStreamInfrastructureException {
if (startPosition == null || startPosition.length() == 0){
if (endPosition == null || endPosition.length() == 0){
return supplier.receive(receiver, fromEnqueuedTime, toEnqueuedTime);
}else{
// TODO: out of range checking for endPosition
return supplier.receive(receiver, fromEnqueuedTime, endPosition);
}
}else{
if (endPosition == null || endPosition.length() == 0){
// TODO: out of range checking for startPosition
return supplier.receive(receiver, startPosition, toEnqueuedTime);
}else{
// TODO: out of range checking for startPosition and endPosition
return supplier.receive(receiver, startPosition, endPosition);
}
}
}
@Override
public Instant getFrom(){
return fromEnqueuedTime;
}
@Override
public Instant getTo(){
return toEnqueuedTime;
}
/**
* Get the from enqueued time
* @return the from enqueued time, can be null
*/
public Instant getFromEnqueuedTime() {
return fromEnqueuedTime;
}
/**
* Set the from enqueued time
* @param fromEnqueuedTime the from enqueued time, can be null
*/
public void setFromPosition(Instant fromEnqueuedTime) {
this.fromEnqueuedTime = fromEnqueuedTime;
}
/**
* Get the to enqueued time
* @return the to enqueued time, can be null
*/
public Instant getToPosition() {
return toEnqueuedTime;
}
/**
* Set the to enqueued time
* @param toEnqueuedTime the to enqueued time, can be null
*/
public void setToPosition(Instant toEnqueuedTime) {
this.toEnqueuedTime = toEnqueuedTime;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy