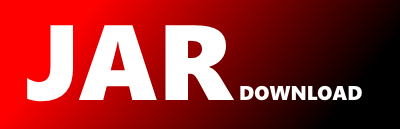
net.sf.jabb.taskq.ScheduledTaskQueues Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jabb-core-java8 Show documentation
Show all versions of jabb-core-java8 Show documentation
Additions to jabb-core that require Java 8
/**
*
*/
package net.sf.jabb.taskq;
import java.io.Serializable;
import java.time.Duration;
import java.time.Instant;
import java.util.List;
import net.sf.jabb.taskq.ex.NoSuchTaskException;
import net.sf.jabb.taskq.ex.NotOwningTaskException;
import net.sf.jabb.taskq.ex.TaskQueueStorageInfrastructureException;
/**
* Queues of tasks that need to be executed at specified times and may have a predecessor.
*
* - processorId: identifier of the task processor. Each thread must have a unique ID of its own.
*
- nextVisibleTime: the task will not be dequeue-able before this time.
* - predecessorId: the task will not be dequeue-able if another task with that ID exists in any queue.
* - timeout: the period that a task remains in the queue but invisible after it is dequeued by a processor.
* - ID of the task: They are generated by the service and are guaranteed to be unique across queues provided by the same service.
*
*
* @author James Hu
*
*/
public interface ScheduledTaskQueues {
/**
* Put a task into the queue.
* @param queue id/name of the queue
* @param detail the detail
* @param expectedExecutionTime the time that the task needs to be executed
* @param predecessorId ID of the predecessor task. This task will not be visible until the predecessor task has been deleted.
* @return ID of the just enqueued task
* @throws TaskQueueStorageInfrastructureException if an exception in the underlying infrastructure happened
*/
String put(String queue, Serializable detail, Instant expectedExecutionTime, String predecessorId) throws TaskQueueStorageInfrastructureException;
/**
* Put a task into the queue.
* @param queue id/name of the queue
* @param detail the detail
* @param expectedExecutionDelay the duration after which the task needs to be executed
* @param predecessorId ID of the predecessor task. This task will not be visible until the predecessor task has been deleted.
* @return ID of the just enqueued task
* @throws TaskQueueStorageInfrastructureException if an exception in the underlying infrastructure happened
*/
default String put(String queue, Serializable detail, Duration expectedExecutionDelay, String predecessorId) throws TaskQueueStorageInfrastructureException{
return put(queue, detail, Instant.now().plus(expectedExecutionDelay), predecessorId);
}
/**
* Put a task into the queue. The task has no predecessor thus its predecessorId will be set to null.
* @param queue id/name of the queue
* @param detail the detail
* @param expectedExecutionTime the time that the task needs to be executed
* @return ID of the just enqueued task
* @throws TaskQueueStorageInfrastructureException if an exception in the underlying infrastructure happened
*/
default String put(String queue, Serializable detail, Instant expectedExecutionTime) throws TaskQueueStorageInfrastructureException{
return put(queue, detail, expectedExecutionTime, null);
}
/**
* Put a task into the queue, and let the expected execution time of the task to be now.
* @param queue id/name of the queue
* @param detail the detail
* @param expectedExecutionDelay the delay after which the task is expected to be executed
* @return ID of the just enqueued task
* @throws TaskQueueStorageInfrastructureException if an exception in the underlying infrastructure happened
*/
default String put(String queue, Serializable detail, Duration expectedExecutionDelay) throws TaskQueueStorageInfrastructureException{
return put(queue, detail, Instant.now().plus(expectedExecutionDelay), null);
}
/**
* Put a task into the queue, and let the expected execution time of the task to be now.
* @param queue id/name of the queue
* @param detail the detail
* @return ID of the just enqueued task
* @throws TaskQueueStorageInfrastructureException if an exception in the underlying infrastructure happened
*/
default String put(String queue, Serializable detail) throws TaskQueueStorageInfrastructureException{
return put(queue, detail, Instant.now(), null);
}
/**
* Put a task into the queue, and let the expected execution time of the task to be now.
* @param queue id/name of the queue
* @param detail the detail
* @param predecessorId ID of the predecessor task. This task will not be visible until the predecessor task has been deleted.
* @return ID of the just enqueued task
* @throws TaskQueueStorageInfrastructureException if an exception in the underlying infrastructure happened
*/
default String put(String queue, Serializable detail, String predecessorId) throws TaskQueueStorageInfrastructureException{
return put(queue, detail, Instant.now(), predecessorId);
}
/**
* Get tasks from the queue.
* @param queue The queue in which tasks will be retrieved
* @param expectedExecutionTime All returned tasks must have an expected execution time no later than this parameter
* @param maxNumOfTasks the maximum number of tasks to be returned
* @param processorId The ID of the processor. This ID will be used to update the task meta data
* @param timeout The time after which the execution of the task will time out and the task will be visible/dequeue-able again.
* @return A list of tasks sorted by (nextVisibleTime, enqueuedTime)
* @throws TaskQueueStorageInfrastructureException if an exception in the underlying infrastructure happened
*/
List get(String queue, Instant expectedExecutionTime, int maxNumOfTasks, String processorId, Instant timeout) throws TaskQueueStorageInfrastructureException;
// get by: where queue = :queues and nextVisibleTime <= :nextVisibleTime and not exists (where id = :predecessorId) order by nextVisibleTime, enqueuedTime limit :limit
// also updated by setting: processorId = :processorId, nextVisibleTime = :timeout
/**
* Get tasks from the queue.
* @param queue The queue in which tasks will be retrieved
* @param expectedExecutionTime All returned tasks must have an expected execution time no later than this parameter
* @param maxNumOfTasks the maximum number of tasks to be returned
* @param processorId The ID of the processor. This ID will be used to update the task meta data
* @param timeoutDuration The duration for this task to be kept invisible in the queue
* @return A list of tasks sorted by (queuePriority, nextVisibleTime, enqueuedTime)
* @throws TaskQueueStorageInfrastructureException if an exception in the underlying infrastructure happened
*/
default List get(String queue, Instant expectedExecutionTime, int maxNumOfTasks, String processorId, Duration timeoutDuration) throws TaskQueueStorageInfrastructureException{
return get(queue, expectedExecutionTime, maxNumOfTasks, processorId, Instant.now().plus(timeoutDuration));
}
/**
* Get tasks from the queue.
* @param queue The queue in which tasks will be retrieved
* @param maxNumOfTasks the maximum number of tasks to be returned
* @param processorId The ID of the processor. This ID will be used to update the task meta data
* @param timeoutDuration The duration for this task to be kept invisible in the queue
* @return A list of tasks sorted by (queuePriority, nextVisibleTime, enqueuedTime)
* @throws TaskQueueStorageInfrastructureException if an exception in the underlying infrastructure happened
*/
default List get(String queue, int maxNumOfTasks, String processorId, Duration timeoutDuration) throws TaskQueueStorageInfrastructureException{
return get(queue, Instant.now(), maxNumOfTasks, processorId, timeoutDuration);
}
/**
* Finish the task, so that it will be removed from the queue.
* @param id ID of the task
* @param processorId ID of the processor
* @throws NotOwningTaskException if the task is not currently owned by the processor
* @throws NoSuchTaskException if the task cannot be found
* @throws TaskQueueStorageInfrastructureException if an exception in the underlying infrastructure happened
*/
void finish(String id, String processorId) throws NotOwningTaskException, NoSuchTaskException, TaskQueueStorageInfrastructureException;
// The effect is deleting: where id = :id and processorId = :processorId and nextVisibleTime > now()
/**
* Finish the task, so that it will be removed from the queue.
* @param task the task
* @param processorId ID of the processor
* @throws NotOwningTaskException if the task is not currently owned by the processor
* @throws NoSuchTaskException if the task cannot be found
* @throws TaskQueueStorageInfrastructureException if an exception in the underlying infrastructure happened
*/
default void finish(ReadOnlyScheduledTask task, String processorId) throws NotOwningTaskException, NoSuchTaskException, TaskQueueStorageInfrastructureException{
finish(task.getTaskId(), processorId);
}
/**
* Abort the task, so that it will be visible in the queue again.
* @param id ID of the task
* @param processorId ID of the processor
* @throws NotOwningTaskException if the task is not currently owned by the processor
* @throws NoSuchTaskException if the task cannot be found
* @throws TaskQueueStorageInfrastructureException if an exception in the underlying infrastructure happened
*/
void abort(String id, String processorId) throws NotOwningTaskException, NoSuchTaskException, TaskQueueStorageInfrastructureException;
// The effect is updating: set nextVisibleTime = now() where id = :id and processorId = :processorId
/**
* Abort the task, so that it will be visible in the queue again.
* @param task the task
* @param processorId ID of the processor
* @throws NotOwningTaskException if the task is not currently owned by the processor
* @throws NoSuchTaskException if the task cannot be found
* @throws TaskQueueStorageInfrastructureException if an exception in the underlying infrastructure happened
*/
default void abort(ReadOnlyScheduledTask task, String processorId) throws NotOwningTaskException, NoSuchTaskException, TaskQueueStorageInfrastructureException{
abort(task.getTaskId(), processorId);
}
/**
* Update the timeout.
* @param id ID of the task
* @param processorId ID of the processor
* @param newTimeout the new time out
* @throws NotOwningTaskException if the task is not currently owned by the processor
* @throws NoSuchTaskException if the task cannot be found
* @throws TaskQueueStorageInfrastructureException if an exception in the underlying infrastructure happened
*/
void renewTimeout(String id, String processorId, Instant newTimeout) throws NotOwningTaskException, NoSuchTaskException, TaskQueueStorageInfrastructureException;
// The effect is updating: set nextVisibleTime = :newTimeout where id = :id and processorId = :processorId
/**
* Update the timeout.
* The effect is updating {@code (nextVisibleTime = NOW() + leasePeriod where id = :id and lastConsumer = :consumerId) }
* @param id ID of the task
* @param processorId ID of the processor
* @param newTimeoutDuration the new duration after which the task execution will time out
* @throws NotOwningTaskException if the task is not currently owned by the processor
* @throws NoSuchTaskException if the task cannot be found
* @throws TaskQueueStorageInfrastructureException if an exception in the underlying infrastructure happened
*/
default void renewTimeout(String id, String processorId, Duration newTimeoutDuration) throws NotOwningTaskException, NoSuchTaskException, TaskQueueStorageInfrastructureException{
renewTimeout(id, processorId, Instant.now().plus(newTimeoutDuration));
}
/**
* Update the timeout.
* @param task the task
* @param processorId ID of the processor
* @param newTimeout the new time out
* @throws NotOwningTaskException if the task is not currently owned by the processor
* @throws NoSuchTaskException if the task cannot be found
* @throws TaskQueueStorageInfrastructureException if an exception in the underlying infrastructure happened
*/
default void renewTimeout(ReadOnlyScheduledTask task, String processorId, Instant newTimeout) throws NotOwningTaskException, NoSuchTaskException, TaskQueueStorageInfrastructureException{
renewTimeout(task.getTaskId(), processorId, newTimeout);
}
/**
* Update the timeout.
* @param task the task
* @param processorId ID of the processor
* @param newTimeoutDuration the new duration after which the task execution will time out
* @throws NotOwningTaskException if the task is not currently owned by the processor
* @throws NoSuchTaskException if the task cannot be found
* @throws TaskQueueStorageInfrastructureException if an exception in the underlying infrastructure happened
*/
default void renewTimeout(ReadOnlyScheduledTask task, String processorId, Duration newTimeoutDuration) throws NotOwningTaskException, NoSuchTaskException, TaskQueueStorageInfrastructureException{
renewTimeout(task.getTaskId(), processorId, newTimeoutDuration);
}
/**
* Clear all tasks in a specific queue
* @param queue the queue in which all tasks will be deleted
* @throws TaskQueueStorageInfrastructureException if an exception in the underlying infrastructure happened
*/
void clear(String queue) throws TaskQueueStorageInfrastructureException;
/**
* Clear all tasks in all queues
* @throws TaskQueueStorageInfrastructureException if an exception in the underlying infrastructure happened
*/
void clearAll() throws TaskQueueStorageInfrastructureException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy