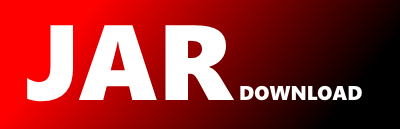
net.sf.jabb.util.ex.ExceptionUncheckUtility Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jabb-core-java8 Show documentation
Show all versions of jabb-core-java8 Show documentation
Additions to jabb-core that require Java 8
package net.sf.jabb.util.ex;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.function.Supplier;
/**
* from http://stackoverflow.com/questions/27644361/how-can-i-throw-checked-exceptions-from-inside-java-8-streams
* @author James Hu
*
*/
public class ExceptionUncheckUtility {
@FunctionalInterface
public interface BiConsumerThrowsExceptions {
void accept(T t, U u) throws Exception;
}
@FunctionalInterface
public interface ConsumerThrowsExceptions {
void accept(T t) throws Exception;
}
@FunctionalInterface
public interface FunctionThrowsExceptions {
R apply(T t) throws Exception;
}
@FunctionalInterface
public interface PredicateThrowsExceptions {
Boolean test(T t) throws Exception;
}
@FunctionalInterface
public interface SupplierThrowsExceptions {
T get() throws Exception;
}
@FunctionalInterface
public interface RunnableThrowsExceptions {
void run() throws Exception;
}
/**
* Usage example:
* .forEach(consumerThrowsUnchecked(name -> System.out.println(Class.forName(name))));
or
* .forEach(consumerThrowsUnchecked(ClassNameUtil::println));
*
* @param argument type of the consumer
* @param consumer the original lambda
* @return the wrapped lambda that throws exceptions in an unchecked manner
*/
public static Consumer consumerThrowsUnchecked(ConsumerThrowsExceptions consumer) {
return t -> {
try {
consumer.accept(t);
} catch (Exception exception) {
throwAsUnchecked(exception);
}
};
}
/**
* Usage example:
* .map(functionThrowsUnchecked(name -> Class.forName(name)))
or
* .map(functionThrowsUnchecked(Class::forName))
*
* @param argument type of the function
* @param return type of the function
* @param function the original lambda
* @return the wrapped lambda that throws exceptions in an unchecked manner
*/
public static Function functionThrowsUnchecked(FunctionThrowsExceptions function) {
return t -> {
try {
return function.apply(t);
} catch (Exception exception) {
throwAsUnchecked(exception);
return null;
}
};
}
/**
* Usage example:
* .map(predicateThrowsUnchecked(t -> Class.isInstance(t))
or
* .map(predicateThrowsUnchecked(Class::isInstance))
*
* @param argument type of the function
* @param function the original lambda
* @return the wrapped lambda that throws exceptions in an unchecked manner
*/
public static Predicate predicateThrowsUnchecked(PredicateThrowsExceptions function) {
return t -> {
try {
return function.test(t);
} catch (Exception exception) {
throwAsUnchecked(exception);
return false;
}
};
}
/**
* Usage example:
* supplierThrowsUnchecked(() -> new StringJoiner(new String(new byte[]{77, 97, 114, 107}, "UTF-8")))
* @param argument type of the function
* @param function the original lambda
* @return the wrapped lambda that throws exceptions in an unchecked manner
*/
public static Supplier supplierThrowsUnchecked(SupplierThrowsExceptions function) {
return () -> {
try {
return function.get();
} catch (Exception exception) {
throwAsUnchecked(exception);
return null;
}
};
}
/**
* Execute the lambda and throw exceptions in an unchecked manner
* Usage example: runThrowingUnchecked(() -> Class.forName("xxx"));
* @param runnable the original lambda
*/
public static void runThrowingUnchecked(RunnableThrowsExceptions runnable) {
try {
runnable.run();
} catch (Exception exception) {
throwAsUnchecked(exception);
}
}
/**
* Execute the lambda and throw exceptions in an unchecked manner
* Usage example: getThrowingUnchecked(() -> Class.forName("xxx"));
* @param return type of the function
* @param supplier the original lambda
* @return the result returned by the lambda
*/
public static R getThrowingUnchecked(SupplierThrowsExceptions supplier) {
try {
return supplier.get();
} catch (Exception exception) {
throwAsUnchecked(exception);
return null;
}
}
/**
* Execute the lambda and throw exceptions in an unchecked manner
* Usage example: applyThrowingUnchecked(Class::forName, "xxx");
* @param argument type of the function
* @param return type of the function
* @param function the original lambda
* @param t argument to the lambda
* @return the result returned by the lambda
*/
public static R applyThrowingUnchecked(FunctionThrowsExceptions function, T t) {
try {
return function.apply(t);
} catch (Exception exception) {
throwAsUnchecked(exception);
return null;
}
}
/**
* Execute the lambda and throw exceptions in an unchecked manner
* Usage example: testThrowingUnchecked(Class::isInstance, "xxx");
* @param argument type of the function
* @param function the original lambda
* @param t argument to the lambda
* @return the result returned by the lambda
*/
public static Boolean testThrowingUnchecked(PredicateThrowsExceptions function, T t) {
try {
return function.test(t);
} catch (Exception exception) {
throwAsUnchecked(exception);
return false;
}
}
/**
* Execute the lambda and throw exceptions in an unchecked manner
* Usage example: acceptThrowingUnchecked(MySystemOut::println, "abc");
* @param argument type of the function
* @param function the original lambda
* @param t argument to the lambda
*/
public static void acceptThrowingUnchecked(ConsumerThrowsExceptions function, T t) {
try {
function.accept(t);
} catch (Exception exception) {
throwAsUnchecked(exception);
}
}
/**
* Throw the exception in an unchecked manner
* @param exception the exception
* @throws E the exception
*/
@SuppressWarnings("unchecked")
private static void throwAsUnchecked(Exception exception) throws E {
throw (E) exception;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy