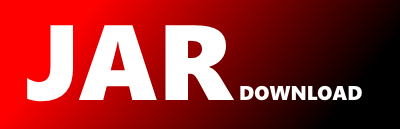
jason.stdlib.substring Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jason Show documentation
Show all versions of jason Show documentation
Jason is a fully-fledged interpreter for an extended version of AgentSpeak, a BDI agent-oriented logic programming language.
package jason.stdlib;
import jason.asSemantics.DefaultInternalAction;
import jason.asSemantics.InternalAction;
import jason.asSemantics.TransitionSystem;
import jason.asSemantics.Unifier;
import jason.asSyntax.NumberTermImpl;
import jason.asSyntax.StringTerm;
import jason.asSyntax.Term;
import java.util.Iterator;
/**
Internal action: .substring
.
Description: checks if a string is sub-string of another
string. The arguments can be other kinds of terms, in which case
the toString() of the term is used. If "position" is a
free variable, the internal action backtracks all possible values
for the positions where the sub-string occurs in the string.
Parameters:
- + substring (any term).
- + string (any term).
- +/- position (optional -- integer): the position of
the string where the sub-string occurs.
Examples:
-
.substring("b","aaa")
: false.
-
.substring("b","aaa",X)
: false.
-
.substring("a","bbacc")
: true.
-
.substring("a","abbacca",X)
: true and X
unifies with 0, 3, and 6.
-
.substring("a","bbacc",0)
: false.
-
.substring(a(10),b(t1,a(10)),X)
: true and X
unifies with 5.
-
.substring(a(10),b("t1,a(10)"),X)
: true and X
unifies with 6.
@see jason.stdlib.concat
@see jason.stdlib.delete
@see jason.stdlib.length
@see jason.stdlib.reverse
*/
public class substring extends DefaultInternalAction {
private static InternalAction singleton = null;
public static InternalAction create() {
if (singleton == null)
singleton = new substring();
return singleton;
}
@Override public int getMinArgs() { return 2; }
@Override public int getMaxArgs() { return 3; }
@Override
public Object execute(TransitionSystem ts, final Unifier un, final Term[] args) throws Exception {
checkArguments(args);
final String s0;
if (args[0].isString())
s0 = ((StringTerm)args[0]).getString();
else
s0 = args[0].toString();
final String s1;
if (args[1].isString())
s1 = ((StringTerm)args[1]).getString();
else
s1 = args[1].toString();
if (args.length == 2) {
// no backtracking utilisation
return s1.indexOf(s0) >= 0;
} else {
// backtrack version: unifies in the third argument all possible positions of s0 in s1
return new Iterator() {
Unifier c = null; // the current response (which is an unifier)
int pos = 0; // current position in s1
public boolean hasNext() {
if (c == null) // the first call of hasNext should find the first response
find();
return c != null;
}
public Unifier next() {
if (c == null) find();
Unifier b = c;
find(); // find next response
return b;
}
void find() {
if (pos < s1.length()) {
pos = s1.indexOf(s0,pos);
if (pos >= 0) {
c = (Unifier)un.clone();
c.unifiesNoUndo(args[2], new NumberTermImpl(pos));
pos++;
return;
}
pos = s1.length(); // to stop searching
}
c = null; // no member is found,
}
public void remove() {}
};
//return un.unifies(args[2], new NumberTermImpl(pos));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy