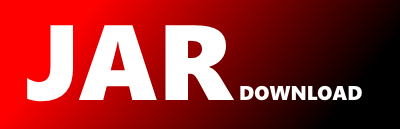
jason.bb.BeliefBase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jason Show documentation
Show all versions of jason Show documentation
Jason is a fully-fledged interpreter for an extended version of AgentSpeak, a BDI agent-oriented logic programming language.
//----------------------------------------------------------------------------
// Copyright (C) 2003 Rafael H. Bordini, Jomi F. Hubner, et al.
//
// This library is free software; you can redistribute it and/or
// modify it under the terms of the GNU Lesser General Public
// License as published by the Free Software Foundation; either
// version 2.1 of the License, or (at your option) any later version.
//
// This library is distributed in the hope that it will be useful,
// but WITHOUT ANY WARRANTY; without even the implied warranty of
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
// Lesser General Public License for more details.
//
// You should have received a copy of the GNU Lesser General Public
// License along with this library; if not, write to the Free Software
// Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
//
// To contact the authors:
// http://www.inf.ufrgs.br/~bordini
// http://www.das.ufsc.br/~jomi
//
//----------------------------------------------------------------------------
package jason.bb;
import java.util.Iterator;
import java.util.Set;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import jason.asSemantics.Agent;
import jason.asSemantics.Unifier;
import jason.asSyntax.Atom;
import jason.asSyntax.Literal;
import jason.asSyntax.Pred;
import jason.asSyntax.PredicateIndicator;
import jason.asSyntax.Term;
/**
* Common interface for all kinds of Jason Belief bases, even those
* customised by the user.
*/
public abstract class BeliefBase implements Iterable, Cloneable {
public static final Term ASelf = new Atom("self");
public static final Term APercept = new Atom("percept");
/** represents the structure 'source(percept)' */
public static final Term TPercept = Pred.createSource(APercept);
/** represents the structure 'source(self)' */
public static final Term TSelf = Pred.createSource(ASelf);
/**
* Called before the MAS execution with the agent that uses this
* BB and the args informed in .mas2j project.
* Example in .mas2j:
* agent BeliefBaseClass(1,bla);
* the init args will be ["1", "bla"].
*/
public void init(Agent ag, String[] args) {}
/** Called just before the end of MAS execution */
public void stop() {}
/** removes all beliefs from BB */
public void clear() {}
public Set getNameSpaces() { return null; }
/** Adds a belief in the end of the BB, returns true if succeed.
* The annots of l may be changed to reflect what was changed in the BB,
* for example, if l is p[a,b] in a BB with p[a], l will be changed to
* p[b] to produce the event +p[b], since only the annotation b is changed
* in the BB. */
public boolean add(Literal l) { return false; }
/** Adds a belief in the BB at index position, returns true if succeed */
public boolean add(int index, Literal l) { return false; }
/** Returns an iterator for all beliefs. */
public abstract Iterator iterator();
/**
* Returns an iterator for all literals in the default namespace of the BB that match the functor/arity
* of the parameter.
*/
public abstract Iterator getCandidateBeliefs(PredicateIndicator pi);
/**
* Returns an iterator for all literals relevant for l's predicate
* indicator, if l is a var, returns all beliefs.
*
* The unifier u may contain values for variables in l.
*
* Example, if BB={a(10),a(20),a(2,1),b(f)}, then
* getCandidateBeliefs(a(5), {})
= {{a(10),a(20)}.
* if BB={a(10),a(20)}, then getCandidateBeliefs(X)
=
* {{a(10),a(20)}. The getCandidateBeliefs(a(X), {X -> 5})
* should also return {{a(10),a(20)}.
*/
public Iterator getCandidateBeliefs(Literal l, Unifier u) { return null; }
/**
* Returns the literal l as it is in BB, this method does not
* consider annotations in the search.
Example, if
* BB={a(10)[a,b]}, contains(a(10)[d])
returns
* a(10)[a,b].
*/
public Literal contains(Literal l) { return null; }
/** Returns the number of beliefs in BB */
public int size() { return 0; }
/** Returns all beliefs that have "percept" as source */
public Iterator getPercepts() { return null; }
/** Removes a literal from BB, returns true if succeed */
public boolean remove(Literal l) { return false; }
/** Removes all believes with some functor/arity in the default namespace */
public boolean abolish(PredicateIndicator pi) { return abolish(Literal.DefaultNS, pi); }
public boolean abolish(Atom namespace, PredicateIndicator pi) { return false; }
/** Gets the BB as XML */
public Element getAsDOM(Document document) { return null; }
public abstract BeliefBase clone();
Object lock = new Object();
/** Gets a lock for the BB */
public Object getLock() {
return lock;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy