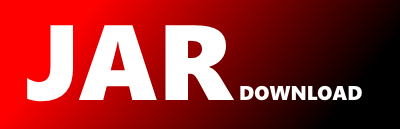
jason.stdlib.nth Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jason Show documentation
Show all versions of jason Show documentation
Jason is a fully-fledged interpreter for an extended version of AgentSpeak, a BDI agent-oriented logic programming language.
The newest version!
package jason.stdlib;
import jason.JasonException;
import jason.asSemantics.DefaultInternalAction;
import jason.asSemantics.InternalAction;
import jason.asSemantics.TransitionSystem;
import jason.asSemantics.Unifier;
import jason.asSyntax.ASSyntax;
import jason.asSyntax.ListTerm;
import jason.asSyntax.NumberTerm;
import jason.asSyntax.Term;
import java.util.Iterator;
/**
Internal action: .nth
.
Description: gets the nth term of a list.
Parameters:
- -/+ index (integer): the position of the term (the first term is at position 0)
- + list (list): the list where to get the term from.
- -/+ term (term): the term at position index in the list.
Examples:
-
.nth(0,[a,b,c],X)
: unifies X
with a
.
-
.nth(2,[a,b,c],X)
: unifies X
with c
.
-
.nth(0,[a,b,c],d)
: false.
-
.nth(0,[a,b,c],a)
: true.
-
.nth(5,[a,b,c],X)
: error.
-
.nth(X,[a,b,c,a,e],a)
: unifies X
with 0
(and 3
if it backtracks).
@see jason.stdlib.concat
@see jason.stdlib.delete
@see jason.stdlib.length
@see jason.stdlib.member
@see jason.stdlib.sort
@see jason.stdlib.max
@see jason.stdlib.min
@see jason.stdlib.reverse
@see jason.stdlib.difference
@see jason.stdlib.intersection
@see jason.stdlib.union
*/
public class nth extends DefaultInternalAction {
private static InternalAction singleton = null;
public static InternalAction create() {
if (singleton == null)
singleton = new nth();
return singleton;
}
@Override public int getMinArgs() {
return 3;
}
@Override public int getMaxArgs() {
return 3;
}
@Override protected void checkArguments(Term[] args) throws JasonException {
super.checkArguments(args); // check number of arguments
if (!args[0].isNumeric() && !args[0].isVar()) {
throw JasonException.createWrongArgument(this,"the first argument should be numeric or a variable -- not '"+args[0]+"'.");
}
if (!args[1].isList()) {
throw JasonException.createWrongArgument(this,"the second argument should be a list and not '"+args[1]+"'.");
}
}
@Override
public Object execute(TransitionSystem ts, final Unifier un, final Term[] args) throws Exception {
checkArguments(args);
ListTerm list = (ListTerm)args[1];
if (args[0].isNumeric()) {
int index = (int)((NumberTerm)args[0]).solve();
if (index < 0 || index >= list.size()) {
throw new JasonException("nth: index "+index+" is out of bounds ("+list.size()+")");
}
return un.unifies(args[2], list.get(index));
}
if (args[0].isVar()) {
final Iterator ilist = list.iterator();
// return all indexes for thirds arg
return new Iterator() {
int index = -1;
Unifier c = null; // the current response (which is an unifier)
public boolean hasNext() {
if (c == null) // the first call of hasNext should find the first response
find();
return c != null;
}
public Unifier next() {
if (c == null) find();
Unifier b = c;
find(); // find next response
return b;
}
void find() {
while (ilist.hasNext()) {
index++;
Term candidate = ilist.next();
c = un.clone();
if (c.unifiesNoUndo( args[2], candidate)) {
c.unifies(args[0], ASSyntax.createNumber(index));
return; // found another response
}
}
c = null; // no more sublists found
}
public void remove() {}
};
}
return false;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy