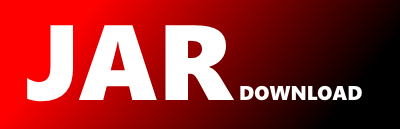
it.discovery.jasperreports.jasper2word.EConvertMisure Maven / Gradle / Ivy
The newest version!
package it.discovery.jasperreports.jasper2word;
import java.math.BigInteger;
/**
* Subset of units of measure. This class contains most used units in word documents and their conversions.
* @author discovery
* @date 24/08/15
*/
public enum EConvertMisure {
/** Equals to 1/2 of point. Used in font size. */
HALF_POINTS,
/** Equals to 1/20 of point. Used in most document parts (ex. margin, spacing, indent ...) */
th20_POINTS,
/** One point. Every jasper report elements is measured in points. Word document table borders too. */
POINTS,
/** Equals to 1/12700 of point. Used to measure document's images. */
EMU,
/** Equals to 1/8 of point. Used to measure borders */
EIGHTS_POINTS
;
/**
* Convert {@code value} from {@code this} type to {@code to} type.
* @param to Destination type for the conversion.
* @param value The value to be converted.
* @return The value in the new unit of measure.
* @see #convertIntoBigInteger(EConvertMisure, double)
*/
public double convertInto(EConvertMisure to, double value) {
switch (this) {
case HALF_POINTS: {
switch (to) {
case HALF_POINTS:
return value;
case th20_POINTS:
return (value / 2D) / 20D;
case POINTS:
return value / 2D;
case EMU:
return Units.toEMU(value / 2D);
case EIGHTS_POINTS:
return (value / 2D) * 8D;
}
}
break;
case th20_POINTS: {
switch (to) {
case HALF_POINTS:
return (value / 20D) * 2D;
case th20_POINTS:
return value;
case POINTS:
return value / 20D;
case EMU:
return Units.toEMU(value / 20D);
case EIGHTS_POINTS:
return (value / 20D) * 8D;
}
}
break;
case POINTS: {
switch (to) {
case HALF_POINTS:
return value * 2D;
case th20_POINTS:
return value * 20D;
case POINTS:
return value;
case EMU:
return Units.toEMU(value);
case EIGHTS_POINTS:
return value * 8D;
}
}
break;
case EMU: {
switch (to) {
case HALF_POINTS:
return Units.toPoints((long) value) * 2D;
case th20_POINTS:
return Units.toPoints((long) value) * 20D;
case POINTS:
return Units.toPoints((long) value);
case EMU:
return value;
case EIGHTS_POINTS:
return Units.toPoints((long) value) * 8D;
}
}
break;
case EIGHTS_POINTS: {
switch (to) {
case HALF_POINTS:
return (value / 8D) * 2D;
case th20_POINTS:
return (value / 8D) * 20D;
case POINTS:
return (value / 8D);
case EMU:
return Units.toEMU(value / 8D);
case EIGHTS_POINTS:
return value;
}
}
}
throw new IllegalArgumentException("Operation not supported");
}
/**
* Same of {@link #convertInto(EConvertMisure, double) convertInto}, but the output type is BigInteger, most used in
* the data structures that map xml word data object.
* @param to Destination type for the conversion.
* @param value The value to be converted.
* @return The value in the new unit of measure.
* @see #convertInto(EConvertMisure, double)
*/
public BigInteger convertIntoBigInteger(EConvertMisure to, double value) {
return BigInteger.valueOf((long) this.convertInto(to, value));
}
/**
* Return a line spacing value according the {@code size} value and the {@code factor}.
* @param size The single line spacing; if {@code null} is assumed as 12.
* @param factor The line spacing factor (es. single = 1, one and half = 1.5, double = 2, etc...)
* @return The line spacing in {@link #th20_POINTS twentieth of points}.
*/
public static BigInteger toLineSpacing(Double size, double factor) {
if (size == null)
size = 12D;
return POINTS.convertIntoBigInteger(th20_POINTS, size * factor);
}
/**
* Utility class for point-to-emu and vice versa conversions.
*/
private static class Units {
/** Emu to point factor. */
public static final int EMU_PER_POINT = 12700;
/**
* Convert points to emu.
* @param points Points to be converted,
* @return The value in emu.
*/
public static int toEMU(double points) {
return (int) Math.round(EMU_PER_POINT * points);
}
/**
* Convert emu to points.
* @param emu The emu to be converted.
* @return The value in points.
*/
public static double toPoints(long emu) {
return (double) emu / EMU_PER_POINT;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy