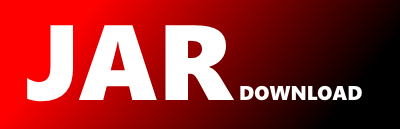
it.discovery.jasperreports.jasper2word.J2WAbstractPrintElementVisitorContext Maven / Gradle / Ivy
The newest version!
package it.discovery.jasperreports.jasper2word;
import it.discovery.jasperreports.jasper2word.J2WGridPageLayout.HeaderFooterPageInfo;
import net.sf.jasperreports.engine.JasperPrint;
import java.util.Map;
import java.util.TreeMap;
/**
* Abstract class for a print element visitor context. This class contains common information valid for every visitor context,
* like the page layout, the last line position written in the document, ecc...
* Subclass can add other information useful in the exporter process.
* @param The class representing the docx output document
* @author discovery
* @date 24/08/15
*/
abstract class J2WAbstractPrintElementVisitorContext {
/** The output document */
private D document;
/** Global exporter configuration */
private J2WReportConfiguration configuration;
/** Store, for each document part, the last line position */
private final Map lastY;
/** Store the last paragraph right index */
private Integer lastParagraphX;
/** Store the last paragraph width */
private Integer lastParagraphWidth;
/** The current page layout */
private J2WGridPageLayout layout;
/** The current report to export */
private JasperPrint report;
/** The last element appended to the document */
private LastDocxElement lastDocxElement;
/** The last header/footer document settings */
private HeaderFooterPageInfo lastPageInfo;
/**
* Constructor.
*/
protected J2WAbstractPrintElementVisitorContext() {
this.lastY = new TreeMap<>();
}
/**
* Return the data object representing the docx document.
* @return The docx document.
*/
public D getDocument() {
return document;
}
/**
* Set the data object representing the docx document.
* @param document The docx document.
*/
public void setDocument(D document) {
this.document = document;
}
/**
* Return the global exporter configuration.
* @return The global exporter configuration.
*/
public J2WReportConfiguration getConfiguration() {
return configuration;
}
/**
* Set the global exporter configuration.
* @param configuration The global exporter configuration.
*/
public void setConfiguration(J2WReportConfiguration configuration) {
this.configuration = configuration;
}
/**
* Return the previous value for the header/footer settings.
* @return The previous value for the header/footer settings.
*/
public HeaderFooterPageInfo getLastPageInfo() {
return lastPageInfo;
}
/**
* Set the value of the last used header/footer settings.
* @param lastPageInfo The last used header/footer settings.
*/
public void setLastPageInfo(HeaderFooterPageInfo lastPageInfo) {
this.lastPageInfo = lastPageInfo;
}
/**
* Return the last line consumed in the document, relative to the document {@code part}.
* @param part The document part.
* @return The last line in the document, that is the last bottom position reached in the document.
*/
public int getLastY(DocxDocumentPart part) {
Integer y = this.lastY.get(part);
return y == null? 0 : y;
}
/**
* Set the last line position reached in the document, relative to the document {@code part}.
* @param part The document part.
* @param lastY The last line position reached in the document.
*/
public void setLastY(DocxDocumentPart part, int lastY) {
this.lastY.put(part, lastY);
}
/**
* Clear all the stored last line positions in the document.
*/
public void resetLastY() {
this.lastY.clear();
}
/**
* Return the right margin of the last paragraph printed in output.
* @return The last right margin.
*/
public Integer getLastParagraphX() {
return lastParagraphX;
}
/**
* Set the right margin of the last paragraph printed in output.
* @param lastParagraphX The last right margin.
*/
public void setLastParagraphX(Integer lastParagraphX) {
this.lastParagraphX = lastParagraphX;
}
/**
* Return the width of the last paragraph printed in output.
* @return The last paragraph width.
*/
public Integer getLastParagraphWidth() {
return lastParagraphWidth;
}
/**
* Set the width of the last paragraph printed in output.
* @param lastParagraphWidth The last paragraph width.
*/
public void setLastParagraphWidth(Integer lastParagraphWidth) {
this.lastParagraphWidth = lastParagraphWidth;
}
/**
* Return the current page layout.
* @return The current page layout.
*/
public J2WGridPageLayout getLayout() {
return layout;
}
/**
* Set the current page layout.
* @param layout The current page layout.
*/
public void setLayout(J2WGridPageLayout layout) {
this.layout = layout;
}
/**
* Return the current report to export.
* @return The current report to export.
*/
public JasperPrint getReport() {
return report;
}
/**
* Set the current report to export.
* @param report The current report to export.
*/
public void setReport(JasperPrint report) {
this.report = report;
}
/**
* Return the last printed document element.
* @return The last printed document element.
*/
public LastDocxElement getLastDocxElement() {
return lastDocxElement;
}
/**
* Set the last printed document element.
* @param lastDocxElement The last printed document element.
*/
public void setLastDocxElement(LastDocxElement lastDocxElement) {
this.lastDocxElement = lastDocxElement;
}
/**
* Reset the context status.
*/
public abstract void resetPageStatus();
/**
* Kind of element that can be append in a docx document.
*/
public enum LastDocxElement {
/** Paragraph element (with text) */
TEXT_PARAGRAPH,
/** A table */
TABLE
}
/**
* Document parts.
*/
public enum DocxDocumentPart {
/** The header of the document */
HEADER,
/** The footer of the document */
FOOTER,
/** The body of the document */
BODY
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy