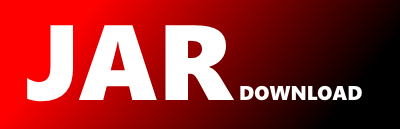
de.mrapp.parser.feed.common.datastructure.AbstractNode Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of javafeedparsercommon Show documentation
Show all versions of javafeedparsercommon Show documentation
Contains common classes of projects, which allow to parse feeds
The newest version!
/*
* JavaFeedParserCommon Copyright 2013-2014 Michael Rapp
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package de.mrapp.parser.feed.common.datastructure;
import static de.mrapp.parser.feed.common.util.Condition.ensureNotEmpty;
import static de.mrapp.parser.feed.common.util.Condition.ensureNotNull;
/**
* An abstract base class for all nodes, which represent an element or attribute
* of a feed's XML structure.
*
* @author Michael Rapp
*
* @since 1.0.0
*/
public abstract class AbstractNode implements Node {
/**
* The constant serial version UID.
*/
private static final long serialVersionUID = 1L;
/**
* The node's name.
*/
private String name;
/**
* The node's namespace.
*/
private Namespace namespace;
/**
* Creates a new node, which represents an element or attribute of a feed's
* XML structure. The node's namespace has no prefix and URI by default.
*
* @param name
* The node's name as a {@link String}. The name may neither be
* null, nor empty
*/
public AbstractNode(final String name) {
setName(name);
setNamespace(new Namespace());
}
@Override
public final String getName() {
return name;
}
/**
* Sets the node's name.
*
* @param name
* The name, which should be set, as a {@link String}. The name
* may neither be null, nor empty
*/
public final void setName(final String name) {
ensureNotNull(name, "The name may not be null");
ensureNotEmpty(name, "The name may not be empty");
this.name = name;
}
@Override
public Namespace getNamespace() {
return namespace;
}
/**
* Sets the node's namespace.
*
* @param namespace
* The namespace, which should be set, as an instance of the
* class {@link Namespace}. The namespace may not be null
*/
public void setNamespace(final Namespace namespace) {
ensureNotNull(namespace, "The namespace may not be null");
this.namespace = namespace;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + name.hashCode();
result = prime * result + namespace.hashCode();
return result;
}
@Override
public boolean equals(final Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
AbstractNode other = (AbstractNode) obj;
if (!name.equals(other.name))
return false;
if (!namespace.equals(other.namespace))
return false;
return true;
}
@Override
public abstract Node clone() throws CloneNotSupportedException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy