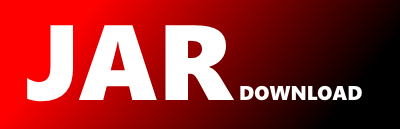
de.mrapp.parser.feed.common.datastructure.Pair Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of javafeedparsercommon Show documentation
Show all versions of javafeedparsercommon Show documentation
Contains common classes of projects, which allow to parse feeds
The newest version!
/*
* JavaFeedParserCommon Copyright 2013-2014 Michael Rapp
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package de.mrapp.parser.feed.common.datastructure;
import static de.mrapp.parser.feed.common.util.Condition.ensureNotNull;
import java.io.Serializable;
/**
* A data structure, which contains a pair of values of any generic type, which
* is extended from the interface {@link Serializable}.
*
*
* @param
* The type of the first value
* @param
* The type of the second value
*
* @author Michael Rapp
*
* @since 1.0.0
*/
public class Pair
implements Serializable {
/**
* The constant serial version UID.
*/
private static final long serialVersionUID = 1L;
/**
* The first value.
*/
private FirstType first;
/**
* The seconds value.
*/
private SecondType second;
/**
* Creates a new data structure, which contains a pair of values.
*
* @param first
* The first value. The value may not be null
* @param second
* The second value. The value may not be null
*/
public Pair(final FirstType first, final SecondType second) {
setFirst(first);
setSecond(second);
}
/**
* Returns the first value.
*
* @return The first value as an instance of the type FirstType. The value
* may not be null
*/
public final FirstType getFirst() {
return first;
}
/**
* Sets the first value.
*
* @param first
* The value, which should be set, as an instance of the type
* FirstType. The value may not be null
*/
public final void setFirst(final FirstType first) {
ensureNotNull(first, "The value may not be null");
this.first = first;
}
/**
* Returns the second value.
*
* @return The second value as an instance of the type SecondType. The value
* may not be null
*/
public final SecondType getSecond() {
return second;
}
/**
* Sets the second value.
*
* @param second
* The value, which should be set, as an instance of the type
* SecondType. The value may not be null
*/
public final void setSecond(final SecondType second) {
ensureNotNull(second, "The value may not be null");
this.second = second;
}
/**
* Creates and returns a new data structure, which contains a pair of
* values.
*
* @param
* The type of the first value
* @param
* The type of the second value
* @param first
* The first value. The value may not be null
* @param second
* The second value. The value may not be null
* @return The pair of values, which has been created
*/
public static Pair create(
final FirstType first, final SecondType second) {
return new Pair(first, second);
}
@Override
public final String toString() {
return "Pair [first=" + first + ", second=" + second + "]";
}
@Override
public final int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + first.hashCode();
result = prime * result + second.hashCode();
return result;
}
@Override
public final boolean equals(final Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (getClass() != obj.getClass())
return false;
Pair, ?> other = (Pair, ?>) obj;
if (!first.equals(other.first))
return false;
if (!second.equals(other.second))
return false;
return true;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy