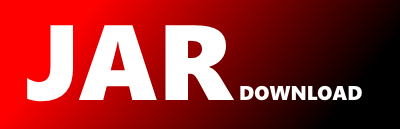
de.mrapp.parser.feed.common.source.UrlSource Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of javafeedparsercommon Show documentation
Show all versions of javafeedparsercommon Show documentation
Contains common classes of projects, which allow to parse feeds
The newest version!
/*
* JavaFeedParserCommon Copyright 2013-2014 Michael Rapp
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package de.mrapp.parser.feed.common.source;
import static de.mrapp.parser.feed.common.util.Condition.ensureNotNull;
import java.io.IOException;
import java.net.URL;
import org.jdom2.Document;
import org.jdom2.JDOMException;
import org.jdom2.input.SAXBuilder;
import de.mrapp.parser.feed.common.builder.InvalidSourceException;
/**
* A source, which allows to read the XML structure of a feed from an
* {@link URL}.
*
* @author Michael Rapp
*
* @since 1.0.0
*/
public class UrlSource implements Source {
/**
* The URL, which allows to read the feed's content.
*/
private URL url;
/**
* Creates a new source, which allows to read the XML structure of a feed
* from an {@link URL}.
*
* @param url
* The URL, which allows to read the feed's content, as an
* instance of the class {@link URL}. The URL may not be null
*/
public UrlSource(final URL url) {
setUrl(url);
}
/**
* Returns the {@link URL}, which allows to read the feed's content.
*
* @return The URL, which allows to read the feed's content, as an instance
* of the class {@link URL}. The URL may not be null
*/
public final URL getUrl() {
return url;
}
/**
* Sets the {@link URL}, which allows to read the feed's content.
*
* @param url
* The URL, which should be set, as an instance of the class
* {@link URL}. The URL may not be null
*/
public final void setUrl(final URL url) {
ensureNotNull(url, "The URL may not be null");
this.url = url;
}
@Override
public final Document getDocument() throws InvalidSourceException {
try {
return new SAXBuilder().build(url);
} catch (JDOMException e) {
throw new InvalidSourceException(e.getMessage(), e);
} catch (IOException e) {
throw new InvalidSourceException(e.getMessage(), e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy