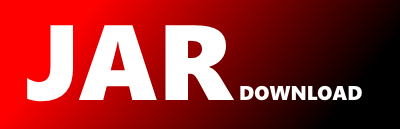
net.sf.javagimmicks.collections.event.AbstractEventNavigableMap Maven / Gradle / Ivy
package net.sf.javagimmicks.collections.event;
import java.util.NavigableMap;
import java.util.NavigableSet;
import net.sf.javagimmicks.collections.transformer.NavigableKeySet;
public abstract class AbstractEventNavigableMap extends AbstractEventSortedMap implements NavigableMap
{
private static final long serialVersionUID = 7570207692375842675L;
public AbstractEventNavigableMap(NavigableMap decorated)
{
super(decorated);
}
@Override
public NavigableMap getDecorated()
{
return (NavigableMap) super.getDecorated();
}
public Entry ceilingEntry(K key)
{
return getDecorated().ceilingEntry(key);
}
public K ceilingKey(K key)
{
return getDecorated().ceilingKey(key);
}
public Entry firstEntry()
{
return getDecorated().firstEntry();
}
public Entry floorEntry(K key)
{
return getDecorated().floorEntry(key);
}
public K floorKey(K key)
{
return getDecorated().floorKey(key);
}
public Entry higherEntry(K key)
{
return getDecorated().higherEntry(key);
}
public K higherKey(K key)
{
return getDecorated().higherKey(key);
}
public Entry lastEntry()
{
return getDecorated().lastEntry();
}
public Entry lowerEntry(K key)
{
return getDecorated().lowerEntry(key);
}
public K lowerKey(K key)
{
return getDecorated().lowerKey(key);
}
public Entry pollFirstEntry()
{
Entry firstEntry = getDecorated().pollFirstEntry();
if(firstEntry != null)
{
fireEntryRemoved(firstEntry.getKey(), firstEntry.getValue());
}
return firstEntry;
}
public Entry pollLastEntry()
{
Entry lastEntry = getDecorated().pollLastEntry();
if(lastEntry != null)
{
fireEntryRemoved(lastEntry.getKey(), lastEntry.getValue());
}
return lastEntry;
}
public NavigableSet descendingKeySet()
{
return descendingMap().navigableKeySet();
}
public NavigableSet navigableKeySet()
{
return new NavigableKeySet(this);
}
public NavigableMap descendingMap()
{
return new EventSubNavigableMap(this, getDecorated().descendingMap());
}
public NavigableMap headMap(K toKey, boolean inclusive)
{
return new EventSubNavigableMap(this, getDecorated().headMap(toKey, inclusive));
}
public NavigableMap subMap(K fromKey, boolean fromInclusive, K toKey, boolean toInclusive)
{
return new EventSubNavigableMap(this, getDecorated().subMap(fromKey, fromInclusive, toKey, toInclusive));
}
public NavigableMap tailMap(K fromKey, boolean inclusive)
{
return new EventSubNavigableMap(this, getDecorated().tailMap(fromKey, inclusive));
}
protected static class EventSubNavigableMap extends AbstractEventNavigableMap
{
private static final long serialVersionUID = 8445308257944385932L;
protected final AbstractEventNavigableMap _parent;
protected EventSubNavigableMap(AbstractEventNavigableMap parent, NavigableMap decorated)
{
super(decorated);
_parent = parent;
}
@Override
protected void fireEntryAdded(K key, V value)
{
_parent.fireEntryAdded(key, value);
}
@Override
protected void fireEntryRemoved(K key, V value)
{
_parent.fireEntryRemoved(key, value);
}
@Override
protected void fireEntryUpdated(K key, V oldValue, V newValue)
{
_parent.fireEntryUpdated(key, oldValue, newValue);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy