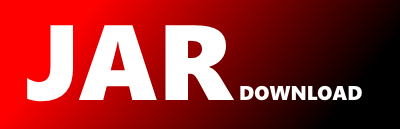
net.sf.javagimmicks.collections.transformer.NavigableKeySet Maven / Gradle / Ivy
package net.sf.javagimmicks.collections.transformer;
import java.util.AbstractSet;
import java.util.Comparator;
import java.util.Iterator;
import java.util.NavigableMap;
import java.util.NavigableSet;
import java.util.SortedSet;
import java.util.Map.Entry;
public class NavigableKeySet extends AbstractSet implements NavigableSet
{
protected final NavigableMap _parent;
public NavigableKeySet(NavigableMap parent)
{
_parent = parent;
}
public Comparator super K> comparator()
{
return _parent.comparator();
}
public K first()
{
return _parent.firstKey();
}
public K last()
{
return _parent.lastKey();
}
@Override
public Iterator iterator()
{
Iterator> entryIterator = _parent.entrySet().iterator();
return TransformerUtils.decorate(entryIterator, new KeyTransformer());
}
@Override
public int size()
{
return _parent.size();
}
public K ceiling(K e)
{
return _parent.ceilingKey(e);
}
public Iterator descendingIterator()
{
return descendingSet().iterator();
}
public NavigableSet descendingSet()
{
return _parent.descendingKeySet();
}
public K floor(K e)
{
return _parent.floorKey(e);
}
public K higher(K e)
{
return _parent.higherKey(e);
}
public K lower(K e)
{
return _parent.lowerKey(e);
}
public K pollFirst()
{
Entry firstEntry = _parent.pollFirstEntry();
return firstEntry != null ? firstEntry.getKey() : null;
}
public K pollLast()
{
Entry lastEntry = _parent.pollLastEntry();
return lastEntry != null ? lastEntry.getKey() : null;
}
public NavigableSet headSet(K toElement, boolean inclusive)
{
return new NavigableKeySet(_parent.headMap(toElement, inclusive));
}
public SortedSet headSet(K toElement)
{
return headSet(toElement, false);
}
public NavigableSet subSet(K fromElement, boolean fromInclusive, K toElement, boolean toInclusive)
{
return new NavigableKeySet(_parent.subMap(fromElement, fromInclusive, toElement, toInclusive));
}
public SortedSet subSet(K fromElement, K toElement)
{
return subSet(fromElement, true, toElement, false);
}
public NavigableSet tailSet(K fromElement, boolean inclusive)
{
return new NavigableKeySet(_parent.tailMap(fromElement, inclusive));
}
public SortedSet tailSet(K fromElement)
{
return tailSet(fromElement, true);
}
protected static class KeyTransformer implements Transformer, K>
{
public K transform(Entry source)
{
return source.getKey();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy