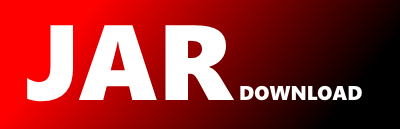
net.sf.javagimmicks.swing.model.ListTreeModelBuilder Maven / Gradle / Ivy
package net.sf.javagimmicks.swing.model;
public class ListTreeModelBuilder
{
protected final ListTreeModel _model;
protected ListTreeNode _currentNode;
protected ListTreeNode _lastChild;
public ListTreeModelBuilder(boolean noChildrenMeansLeaf)
{
_model = new ListTreeModel(noChildrenMeansLeaf);
}
public ListTreeModelBuilder()
{
_model = new ListTreeModel();
}
public ListTreeModelBuilder child(E value)
{
if(_currentNode == null && _lastChild == null)
{
_lastChild = _model.createRoot(value);
}
else if(_currentNode == null)
{
throw new IllegalStateException("Can only add one child on the root level!");
}
else
{
_lastChild = _currentNode.addChild(value);
}
return this;
}
public ListTreeModelBuilder children()
{
if(_lastChild == null)
{
throw new IllegalStateException("No node created yet!");
}
_currentNode = _lastChild;
_lastChild = null;
return this;
}
public ListTreeModelBuilder parent()
{
if(_currentNode == null)
{
throw new IllegalStateException("There is no parent on the root level!");
}
_lastChild = _currentNode;
_currentNode = _currentNode.getParent();
return this;
}
public ListTreeModel buildModel()
{
return _model;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy