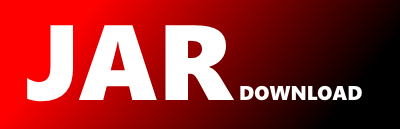
net.sf.javagimmicks.collections.AbstractRingCursor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gimmicks Show documentation
Show all versions of gimmicks Show documentation
Utility classes, APIs and tools for Java
package net.sf.javagimmicks.collections;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.NoSuchElementException;
/**
* An abstract implementation of {@link RingCursor} that provides default
* implementations for some derivable methods.
*
* These are:
*
* - {@link #insertAfter(Iterable)}
* - {@link #insertBefore(Iterable)}
* - {@link #next(int)}
* - {@link #previous(int)}
* - {@link #isEmpty()}
* - {@link #set(Object)}
* - {@link #iterator()}
* - {@link #toList()}
* - {@link #toString()}
*
*/
public abstract class AbstractRingCursor extends AbstractCursor implements RingCursor
{
/**
* A re-implementation of {@link AbstractRingCursor#next(int)} that optimizes
* the internal logic by leveraging advances {@link Ring} features.
*/
@Override
public E next(int count)
{
if (count < 0)
{
return previous(-count);
}
if (count == 0)
{
return get();
}
final int size = size();
count = count % size;
if (count > size / 2)
{
return previous(size - count);
}
else
{
E result = null;
for (int i = 0; i < count; ++i)
{
result = next();
}
return result;
}
}
/**
* A re-implementation of {@link AbstractRingCursor#previous(int)} that
* optimizes the internal logic by leveraging advances {@link Ring} features.
*/
@Override
public E previous(int count)
{
if (count < 0)
{
return next(-count);
}
if (count == 0)
{
return get();
}
final int size = size();
count = count % size;
if (count > size / 2)
{
return next(size - count);
}
else
{
E result = null;
for (int i = 0; i < count; ++i)
{
result = previous();
}
return result;
}
}
@Override
public boolean isEmpty()
{
return size() == 0;
}
@Override
public E set(final E value)
{
if (isEmpty())
{
throw new NoSuchElementException("There is no element to replace");
}
insertAfter(value);
return remove();
}
@Override
public Iterator iterator()
{
return new RingIterator(cursor());
}
@Override
public List toList()
{
final ArrayList result = new ArrayList(size());
for (final E element : this)
{
result.add(element);
}
return result;
}
@Override
public String toString()
{
return toList().toString();
}
protected static class RingIterator implements Iterator
{
private final RingCursor _ringCursor;
private final int _size;
private int _counter = 0;
private boolean _removeCalled = true;
public RingIterator(final RingCursor ringCursor)
{
_ringCursor = ringCursor;
_ringCursor.previous();
_size = ringCursor.size();
}
@Override
public boolean hasNext()
{
return _counter != _size;
}
@Override
public E next()
{
if (!hasNext())
{
throw new NoSuchElementException();
}
_removeCalled = false;
++_counter;
return _ringCursor.next();
}
@Override
public void remove()
{
if (_removeCalled)
{
throw new IllegalStateException(
"next() has not yet been called since last remove() call or creation of this iterator!");
}
_removeCalled = true;
_ringCursor.remove();
if (!_ringCursor.isEmpty())
{
_ringCursor.previous();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy