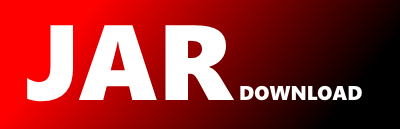
net.sf.javagimmicks.collections.ListComparator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gimmicks Show documentation
Show all versions of gimmicks Show documentation
Utility classes, APIs and tools for Java
package net.sf.javagimmicks.collections;
import java.util.Comparator;
import java.util.Iterator;
import java.util.List;
import net.sf.javagimmicks.util.ComparableComparator;
/**
* A {@link Comparator} implementation able to compare {@link List}s based on a
* given compare logic on the element level.
*
* @param
* the type of elements to compare
*/
@SuppressWarnings("unchecked")
public class ListComparator implements Comparator>
{
private final Comparator _elementComparator;
/**
* A reusable instance able to compare {@link List}s of {@link Comparable}
* elements
*/
@SuppressWarnings("rawtypes")
public static ListComparator extends Comparable>> COMPARABLE_INSTANCE = new ListComparator(
ComparableComparator.INSTANCE);
/**
* Creates a new instance based on a given element {@link Comparator}
*
* @param elementComparator
* the element {@link Comparator} to use for comparing
*/
public ListComparator(final Comparator elementComparator)
{
_elementComparator = elementComparator;
}
public static > ListComparator getComparableInstance()
{
return (ListComparator) COMPARABLE_INSTANCE;
}
@Override
public int compare(final List list1, final List list2)
{
final Iterator iterator1 = list1.iterator();
final Iterator iterator2 = list2.iterator();
while (iterator1.hasNext() && iterator2.hasNext())
{
final E element1 = iterator1.next();
final E element2 = iterator2.next();
final int iCompareResult = _elementComparator.compare(element1, element2);
if (iCompareResult != 0)
{
return iCompareResult;
}
}
if (iterator1.hasNext())
{
return -1;
}
else if (iterator2.hasNext())
{
return 1;
}
else
{
return 0;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy