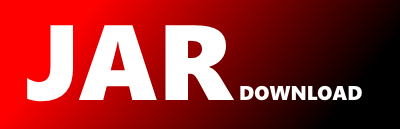
net.sf.javagimmicks.collections.builder.ListBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gimmicks Show documentation
Show all versions of gimmicks Show documentation
Utility classes, APIs and tools for Java
package net.sf.javagimmicks.collections.builder;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.LinkedList;
import java.util.List;
/**
* A builder class for building {@link List}s using a fluent API with one
* chained call.
*
* @param
* the type of elements the resulting {@link List} can carry
* @param
* the type of the produced {@link List}
*/
public class ListBuilder> extends CollectionBuilder
{
/**
* Wraps a new {@link ListBuilder} around the given {@link List}
*
* @param internalList
* the {@link List} to wrap a new {@link ListBuilder} around
* @param
* the type of elements the resulting {@link List} can carry
* @param
* the type of the produced {@link List}
* @return the resulting {@link ListBuilder}
*/
public static > ListBuilder create(final T internalList)
{
return new ListBuilder(internalList);
}
/**
* Creates a new {@link ListBuilder} for building a new {@link ArrayList}
*
* @param
* the type of elements the resulting {@link ArrayList} can carry
* @return the resulting {@link ListBuilder}
*/
public static ListBuilder> createArrayList()
{
return create(new ArrayList());
}
/**
* Creates a new {@link ListBuilder} for building a new {@link ArrayList}
* with the given initial capacity
*
* @param initialCapcity
* the initial capacity to use for the wrapped {@link ArrayList}
* @param
* the type of elements the resulting {@link ArrayList} can carry
* @return the resulting {@link ListBuilder}
*/
public static ListBuilder> createArrayList(final int initialCapcity)
{
return create(new ArrayList(initialCapcity));
}
/**
* Creates a new {@link ListBuilder} for building a new {@link LinkedList}
*
* @param
* the type of elements the resulting {@link LinkedList} can carry
* @return the resulting {@link ListBuilder}
*/
public static ListBuilder> createLinkedList()
{
return create(new LinkedList());
}
/**
* Creates a new {@link ListBuilder} around a given internal {@link List}
*
* @param internalList
* the {@link List} to wrap around
*/
public ListBuilder(final T internalList)
{
super(internalList);
}
/**
* Calls {@link List#add(int, Object)} on the underlying {@link List} and
* returns itself
*
* @param index
* the index where to add the new element in the {@link List}
* @param e
* the element to add
* @return the {@link ListBuilder} itself
* @see List#add(int, Object)
*/
public ListBuilder add(final int index, final E e)
{
_internalCollection.add(index, e);
return this;
}
/**
* Calls {@link List#addAll(int, Collection)} on the underlying {@link List}
* and returns itself
*
* @param index
* the index where to add the new elements in the {@link List}
* @param c
* the {@link Collection} whose elements should be added
* @return the {@link ListBuilder} itself
* @see List#addAll(int, Collection)
*/
public ListBuilder addAll(final int index, final Collection extends E> c)
{
_internalCollection.addAll(index, c);
return this;
}
/**
* Adds any number of elements given as a variable argument list to the
* underlying {@link List} and returns itself
*
* @param index
* the index where to add the new elements in the {@link List}
* @param elements
* the list of elements to add
* @return the {@link ListBuilder} itself
* @see #addAll(int, Collection)
*/
public ListBuilder addAll(final int index, final E... elements)
{
return addAll(Arrays.asList(elements));
}
/**
* Calls {@link List#remove(int)} on the underlying {@link List} and returns
* itself
*
* @param index
* the index where to remove an element
* @return the {@link ListBuilder} itself
* @see List#remove(int)
*/
public ListBuilder remove(final int index)
{
_internalCollection.remove(index);
return this;
}
/**
* Calls {@link List#set(int, Object)} on the underlying {@link List} and
* returns itself
*
* @param index
* the index where to set the new element
* @param e
* the element to set in the underlying {@link List}
* @return the {@link ListBuilder} itself
* @see List#set(int, Object)
*/
public ListBuilder set(final int index, final E e)
{
_internalCollection.set(index, e);
return this;
}
/**
* Calls {@link List#add(Object)} on the underlying {@link List} and returns
* itself
*
* @param e
* the element to add
* @return the {@link ListBuilder} itself
* @see List#add(Object)
*/
@Override
public ListBuilder add(final E e)
{
return (ListBuilder) super.add(e);
}
/**
* Calls {@link List#addAll(Collection)} on the underlying {@link List} and
* returns itself
*
* @param c
* the {@link Collection} whose elements should be added
* @return the {@link ListBuilder} itself
* @see List#addAll(Collection)
*/
@Override
public ListBuilder addAll(final Collection extends E> c)
{
return (ListBuilder) super.addAll(c);
}
/**
* Adds any number of elements given as a variable argument list to the
* underlying {@link List} and returns itself
*
* @param elements
* the list of elements to add
* @return the {@link ListBuilder} itself
* @see #addAll(Collection)
*/
@Override
public ListBuilder addAll(final E... elements)
{
return (ListBuilder) super.addAll(elements);
}
/**
* Calls {@link List#clear()} on the underlying {@link List} and returns
* itself
*
* @return the {@link ListBuilder} itself
* @see List#clear()
*/
@Override
public ListBuilder clear()
{
return (ListBuilder) super.clear();
}
/**
* Calls {@link List#remove(Object)} on the underlying {@link List} and
* returns itself
*
* @param o
* the element to remove from the underlying {@link List}
* @return the {@link ListBuilder} itself
* @see List#remove(Object)
*/
@Override
public ListBuilder remove(final Object o)
{
return (ListBuilder) super.remove(o);
}
/**
* Calls {@link List#removeAll(Collection)} on the underlying {@link List}
* and returns itself
*
* @param c
* the {@link Collection} of elements to remove from the underlying
* {@link List}
* @return the {@link ListBuilder} itself
* @see List#removeAll(Collection)
*/
@Override
public ListBuilder removeAll(final Collection> c)
{
return (ListBuilder) super.removeAll(c);
}
/**
* Removes any number of elements given as a variable argument list from the
* underlying {@link List} and returns itself
*
* @param elements
* the list of elements to remove
* @return the {@link ListBuilder} itself
* @see #removeAll(Collection)
*/
@Override
public ListBuilder removeAll(final E... elements)
{
return (ListBuilder) super.removeAll(elements);
}
/**
* Calls {@link List#retainAll(Collection)} on the underlying {@link List}
* and returns itself
*
* @param c
* the {@link Collection} of elements to retain in the underlying
* {@link List}
* @return the {@link ListBuilder} itself
* @see List#retainAll(Collection)
*/
@Override
public ListBuilder retainAll(final Collection> c)
{
return (ListBuilder) super.retainAll(c);
}
/**
* Retains any number of elements given as a variable argument list in the
* underlying {@link List} and returns itself
*
* @param elements
* the list of elements to retain
* @return the {@link ListBuilder} itself
* @see #retainAll(Collection)
*/
@Override
public ListBuilder retainAll(final E... elements)
{
return (ListBuilder) super.retainAll(elements);
}
/**
* Returns the underlying {@link List}
*
* @return the underlying {@link List}
*/
public T toList()
{
return toCollection();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy