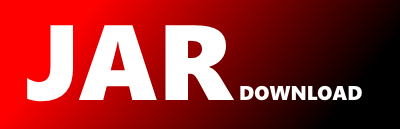
net.sf.javagimmicks.collections.composite.CompositeIterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gimmicks Show documentation
Show all versions of gimmicks Show documentation
Utility classes, APIs and tools for Java
package net.sf.javagimmicks.collections.composite;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import java.util.NoSuchElementException;
import net.sf.javagimmicks.collections.transformer.TransformerUtils;
import net.sf.javagimmicks.util.Function;
class CompositeIterator implements Iterator
{
protected final Iterator extends Iterator> _iterators;
protected Iterator _currentIterator;
protected Iterator _nextIterator;
CompositeIterator(List extends Iterator> iterators)
{
_iterators = iterators.iterator();
findNextIterator();
}
static > CompositeIterator fromCollectionList(List collections)
{
final Function> iteratorExtractor = new Function>()
{
public Iterator apply(C source)
{
return source.iterator();
}
};
final List> iteratorList = new ArrayList>(TransformerUtils.decorate(collections, iteratorExtractor));
return new CompositeIterator(iteratorList);
}
public boolean hasNext()
{
if(_currentIterator != null && _currentIterator.hasNext())
{
return true;
}
findNextIterator();
return _nextIterator != null && _nextIterator.hasNext();
}
public E next()
{
if(!hasNext())
{
throw new NoSuchElementException();
}
return moveNext();
}
public void remove()
{
if(_currentIterator == null)
{
throw new IllegalStateException();
}
_currentIterator.remove();
}
protected void findNextIterator()
{
if(_nextIterator != null)
{
return;
}
while(_iterators.hasNext())
{
_nextIterator = _iterators.next();
if(_nextIterator.hasNext())
{
break;
}
}
}
protected E moveNext()
{
if(_nextIterator != null)
{
_currentIterator = _nextIterator;
_nextIterator = null;
}
return _currentIterator.next();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy