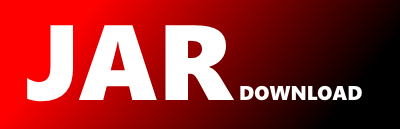
net.sf.javagimmicks.collections.composite.CompositeUtils Maven / Gradle / Ivy
Show all versions of gimmicks Show documentation
package net.sf.javagimmicks.collections.composite;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Enumeration;
import java.util.Iterator;
import java.util.List;
import java.util.ListIterator;
/**
* The central entry point into the composite API. Provides numerous creation
* methods for different collection types.
*
* Each method takes a number of collections of one type and returns a new one
* of the same type that serves as a composite for the given ones.
*/
@SuppressWarnings("unchecked")
public class CompositeUtils
{
private CompositeUtils()
{}
/**
* Creates a composite {@link Enumeration} around any number of other ones
* (provided as {@link Collection} of {@link Enumeration}s)
*
* @param enumerations
* the {@link Enumeration}s to wrap a composite one around
* @param
* the type of elements of treated {@link Enumeration}s
* @return a composite {@link Enumeration} wrapped around the given ones
*/
public static Enumeration enumeration(final Collection> enumerations)
{
return new CompositeEnumeration(new ArrayList>(enumerations));
}
/**
* Creates a composite {@link Enumeration} around any number of other ones
* (provided as variable argument list of {@link Enumeration}s)
*
* @param enumerations
* the {@link Enumeration}s to wrap a composite one around
* @param
* the type of elements of treated {@link Enumeration}s
* @return a composite {@link Enumeration} wrapped around the given ones
*/
public static Enumeration enumeration(final Enumeration... enumerations)
{
return enumeration(Arrays.asList(enumerations));
}
/**
* Creates a composite {@link Enumeration} around two given ones
*
* @param e1
* the first {@link Enumeration} to build a composite one from
* @param e2
* the first {@link Enumeration} to build a composite one from
* @param
* the type of elements of treated {@link Enumeration}s
* @return a composite {@link Enumeration} wrapped around the given ones
*/
public static Enumeration enumeration(final Enumeration e1, final Enumeration e2)
{
return new CompositeEnumeration(Arrays.asList(e1, e2));
}
/**
* Creates a composite {@link Iterator} around any number of other ones
* (provided as {@link Collection} of {@link Iterator}s)
*
* @param iterators
* the {@link Iterator}s to wrap a composite one around
* @param
* the type of elements of treated {@link Iterator}s
* @param
* the {@link Collection} type containing the {@link Iterator}s
* @return a composite {@link Iterator} wrapped around the given ones
*/
public static > Iterator iterator(final Collection iterators)
{
return new CompositeIterator(new ArrayList(iterators));
}
/**
* Creates a composite {@link Iterator} around any number of other ones
* (provided as variable argument list of {@link Iterator}s)
*
* @param iterators
* the {@link Iterator}s to wrap a composite one around
* @param
* the type of elements of treated {@link Iterator}s
* @return a composite {@link Iterator} wrapped around the given ones
*/
public static Iterator iterator(final Iterator... iterators)
{
return iterator(Arrays.asList(iterators));
}
/**
* Creates a composite {@link Iterator} around two given ones
*
* @param i1
* the first {@link Iterator} to build a composite one from
* @param i2
* the first {@link Iterator} to build a composite one from
* @param
* the type of elements of treated {@link Iterator}s
* @return a composite {@link Iterator} wrapped around the given ones
*/
public static Iterator iterator(final Iterator i1, final Iterator i2)
{
return new CompositeIterator(Arrays.asList(i1, i2));
}
/**
* Creates a composite {@link Collection} around any number of other ones
* (provided as {@link Collection} of {@link Collection}s)
*
* @param collections
* the {@link Collection}s to wrap a composite one around
* @param
* the type of elements of treated {@link Collection}s
* @param
* the {@link Collection} type containing the {@link Collection}s
* @return a composite {@link Collection} wrapped around the given ones
*/
public static > Collection collection(final Collection collections)
{
return new CompositeCollection(new ArrayList(collections));
}
/**
* Creates a composite {@link Collection} around any number of other ones
* (provided as variable argument list of {@link Collection}s)
*
* @param collections
* the {@link Collection}s to wrap a composite one around
* @param
* the type of elements of treated {@link Collection}s
* @return a composite {@link Collection} wrapped around the given ones
*/
public static Collection collection(final Collection... collections)
{
return collection(Arrays.asList(collections));
}
/**
* Creates a composite {@link Collection} around two given ones
*
* @param c1
* the first {@link Collection} to build a composite one from
* @param c2
* the first {@link Collection} to build a composite one from
* @param
* the type of elements of treated {@link Collection}s
* @return a composite {@link Collection} wrapped around the given ones
*/
public static Collection collection(final Collection c1, final Collection c2)
{
return new CompositeCollection(Arrays.asList(c1, c2));
}
/**
* Creates a composite {@link ListIterator} around any number of other ones
* (provided as {@link List} of {@link ListIterator}s)
*
* @param listIterators
* the {@link ListIterator}s to wrap a composite one around
* @param
* the type of elements of treated {@link ListIterator}s
* @param
* the {@link Collection} type containing the {@link ListIterator}s
* @return a composite {@link ListIterator} wrapped around the given ones
*/
public static > ListIterator listIterator(final List listIterators)
{
return new CompositeListIterator(new ArrayList(listIterators));
}
/**
* Creates a composite {@link ListIterator} around any number of other ones
* (provided as variable argument list of {@link ListIterator}s)
*
* @param listIterators
* the {@link ListIterator}s to wrap a composite one around
* @param
* the type of elements of treated {@link ListIterator}s
* @return a composite {@link ListIterator} wrapped around the given ones
*/
public static ListIterator listIterator(final ListIterator... listIterators)
{
return listIterator(Arrays.asList(listIterators));
}
/**
* Creates a composite {@link ListIterator} around two given ones
*
* @param it1
* the first {@link ListIterator} to build a composite one from
* @param it2
* the first {@link ListIterator} to build a composite one from
* @param
* the type of elements of treated {@link ListIterator}s
* @return a composite {@link ListIterator} wrapped around the given ones
*/
public static ListIterator listIterator(final ListIterator it1, final ListIterator it2)
{
return new CompositeListIterator(Arrays.asList(it1, it2));
}
/**
* Creates a composite {@link List} around any number of other ones (provided
* as {@link List} of {@link List}s)
*
* @param lists
* the {@link List}s to wrap a composite one around
* @param
* the type of elements of treated {@link List}s
* @param
* the {@link Collection} type containing the {@link List}s
* @return a composite {@link List} wrapped around the given ones
*/
public static > List list(final List lists)
{
return new CompositeList(new ArrayList(lists));
}
/**
* Creates a composite {@link List} around any number of other ones (provided
* as variable argument list of {@link List}s)
*
* @param lists
* the {@link List}s to wrap a composite one around
* @param
* the type of elements of treated {@link List}s
* @return a composite {@link List} wrapped around the given ones
*/
public static List list(final List... lists)
{
return list(Arrays.asList(lists));
}
/**
* Creates a composite {@link List} around two given ones
*
* @param l1
* the first {@link List} to build a composite one from
* @param l2
* the first {@link List} to build a composite one from
* @param
* the type of elements of treated {@link List}s
* @return a composite {@link List} wrapped around the given ones
*/
public static List list(final List l1, final List l2)
{
return new CompositeList(Arrays.asList(l1, l2));
}
}