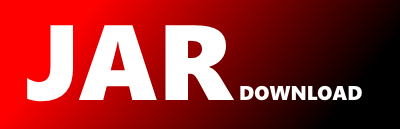
net.sf.javagimmicks.collections.diff.Difference Maven / Gradle / Ivy
package net.sf.javagimmicks.collections.diff;
import java.util.List;
/**
* Represents one single difference (unit) between two {@link List}s.
*
* Note that the comparison of two {@link List}s will usually result in
* multiple differences, which this API encapsulates with a
* {@link DifferenceList}.
*
* One single difference always consists of a delete range of elements
* and/or an add range of elements. These ranges are represented
* as {@link Range} instances.
*
* Examples
*
* - When comparing [1, 2, 3, 4] to [1, X, Y, Z, 4] there is a delete
* {@link Range} [2, 3] and an add {@link Range} [X, Y, Z]
* - When comparing [1, 2, 3, 4] to [1, 4] there is a delete {@link Range}
* [2, 3] but no add {@link Range}
* - When comparing [1, 4] to [1, 2, 3, 4] there is no delete
* {@link Range} but an add {@link Range} [2, 3]
*
*
* @param
* the type of elements the two compared {@link List}s have
* @see DifferenceList
*/
public interface Difference
{
/**
* The pseudo end index ({@value #NONE}) if there is no delete or add range.
*/
int NONE = -1;
/**
* Returns the delete {@link Range} of this {@link Difference}. This
* will never return {@code null} event if there is no such {@link Range} -
* instead {@link Range#exists()} will result to {@code false}.
*
* @return the delete {@link Range}
*/
Range deleteRange();
/**
* Returns the add {@link Range} of this {@link Difference}. This will
* never return {@code null} event if there is no such {@link Range} -
* instead {@link Range#exists()} will result to {@code false}.
*
* @return the add {@link Range}
*/
Range addRange();
/**
* Produces an inverted version of this {@link Difference} which means that
* delete range and add range are exchanged.
*
* @return an inverted version of this {@link Difference}
*/
Difference invert();
/**
* Encapsulates a range (of type delete or add) that a
* {@link Difference} will contain.
*
* Note: a {@link Range} is defined to be read-only, so implementors
* should ensure this.
*
* @param
* the type of elements the surrounding {@link Difference} operates
* on.
*/
interface Range extends List
{
/**
* Returns the index of the first element of the range or 0 if there is
* none ({@link #exists()} resolves to {@code false}).
*
* @return the first index of the range
* @see #exists()
*/
int getStartIndex();
/**
* Returns the index of the last element of the range or {@link #NONE} if
* there is none ({@link #exists()} resolves to {@code false}).
*
* @return the last index of the range
* @see #exists()
*/
int getEndIndex();
/**
* Returns if the range exists in the surrounding {@link Difference}
*
* @return if the range exists in the surrounding {@link Difference}
*/
boolean exists();
}
}