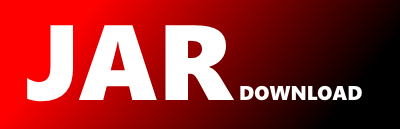
net.sf.javagimmicks.collections.transformer.MappedTransformerUtils Maven / Gradle / Ivy
Show all versions of gimmicks Show documentation
package net.sf.javagimmicks.collections.transformer;
import java.util.Map;
import net.sf.javagimmicks.collections.bidimap.BidiMap;
import net.sf.javagimmicks.transform.BidiFunction;
import net.sf.javagimmicks.transform.Functions;
import net.sf.javagimmicks.util.Function;
/**
* Provides features to build {@link Function}s based on {@link Map}s and
* {@link BidiFunction}s based on {@link BidiMap}s.
*/
public class MappedTransformerUtils
{
private MappedTransformerUtils()
{}
/**
* Creates a new {@link Function} based on a given {@link Map} which
* transforms values by looking them up in the given {@link Map}.
*
* Attention: the resulting {@link Function} will throw an
* {@link IllegalArgumentException} if it should transform a value the has no
* corresponding key within the given {@link Map}.
*
* @param map
* the {@link Map} to wrap into a {@link Function}
* @param
* the "from" or source type
* @param
* the "to" or target type
*
* @return the resulting {@link Function}
*/
public static Function asTransformer(final Map map)
{
return new MapTransformer(map);
}
/**
* Creates a new {@link BidiFunction} based on a given {@link BidiMap}
* which transforms values by looking them up in the given {@link BidiMap}.
*
* Attention: the resulting {@link BidiFunction} will throw an
* {@link IllegalArgumentException} if it should transform a value the has no
* corresponding key (or value) within the given {@link BidiMap}.
*
* @param bidiMap
* the {@link BidiMap} to wrap into a {@link BidiFunction}
* @param
* the "from" or source type
* @param
* the "to" or target type
* @return the resulting {@link BidiFunction}
*/
public static BidiFunction asBidiTransformer(final BidiMap bidiMap)
{
return new BidiMapBidiTransformer(bidiMap);
}
protected static class MapTransformer implements Function
{
protected final Map _baseMap;
protected MapTransformer(final Map map)
{
_baseMap = map;
}
@Override
public T apply(final F source)
{
if (!_baseMap.containsKey(source))
{
throw new IllegalArgumentException("This MapTransformer doesn't contain the given source element '"
+ source + "'!");
}
return _baseMap.get(source);
}
}
protected static class BidiMapBidiTransformer extends MapTransformer implements BidiFunction
{
protected BidiMapBidiTransformer(final BidiMap map)
{
super(map);
}
@Override
public BidiFunction invert()
{
return Functions.invert(this);
}
@Override
public F applyReverse(final T target)
{
if (!_baseMap.containsValue(target))
{
throw new IllegalArgumentException("This BidiMapTransformer doesn't contain the given target element '"
+ target + "'!");
}
return ((BidiMap) _baseMap).getKey(target);
}
}
}