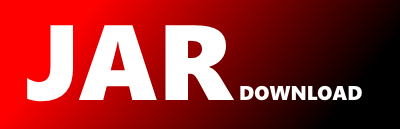
net.sf.javagimmicks.collections8.ArrayRing Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gimmicks Show documentation
Show all versions of gimmicks Show documentation
Utility classes, APIs and tools for Java
package net.sf.javagimmicks.collections8;
import java.util.ArrayList;
import java.util.NoSuchElementException;
/**
* An implementation of {@link Ring} that internally operates on an
* {@link ArrayList}.
*/
public class ArrayRing extends AbstractRing
{
private final ArrayList _backingList;
/**
* Constructs a new instance with a default {@link ArrayList} in the
* background.
*/
public ArrayRing()
{
_backingList = new ArrayList();
}
/**
* Constructs a new instance with a {@link ArrayList} in the background that
* has the given initial capacity.
*
* @param initialCapacity
* the initial capacity that should be reserved on the internal
* {@link ArrayList}
*
* @see ArrayList#ArrayList(int)
*/
public ArrayRing(final int initialCapacity)
{
_backingList = new ArrayList(initialCapacity);
}
@Override
public int size()
{
return _backingList.size();
}
@Override
public RingCursor cursor()
{
return new ArrayRingCursor(this, 0);
}
/**
* Adjusts the underlying {@link ArrayList} to have the minimum given
* capacity.
*
* @param minCapacity
* the new minimal capacity
* @see ArrayList#ensureCapacity(int)
*/
public void ensureCapacity(final int minCapacity)
{
_backingList.ensureCapacity(minCapacity);
}
private static class ArrayRingCursor extends BasicRingCursor>
{
private int _position;
private ArrayRingCursor(final ArrayRing ring, final int position)
{
super(ring);
_position = position;
}
@Override
public E get()
{
checkForModification();
if (_ring._backingList.isEmpty())
{
throw new NoSuchElementException();
}
return _ring._backingList.get(_position);
}
@Override
public void insertAfter(final E value)
{
checkForModification();
if (_ring.isEmpty())
{
_ring._backingList.add(value);
_position = 0;
}
else
{
_ring._backingList.add(_position + 1, value);
}
++_ring._modCount;
++_expectedModCount;
}
@Override
public void insertBefore(final E value)
{
checkForModification();
if (_position == 0)
{
_ring._backingList.add(value);
}
else
{
_ring._backingList.add(_position, value);
++_position;
}
++_ring._modCount;
++_expectedModCount;
}
@Override
public E next()
{
checkForModification();
if (_ring.isEmpty())
{
throw new NoSuchElementException("Ring is empty");
}
++_position;
if (_position == _ring.size())
{
_position = 0;
}
return get();
}
@Override
public E previous()
{
checkForModification();
if (_ring.isEmpty())
{
throw new NoSuchElementException("Ring is empty");
}
--_position;
if (_position == -1)
{
_position += _ring.size();
}
return get();
}
@Override
public E remove()
{
checkForModification();
if (_ring.isEmpty())
{
throw new NoSuchElementException("Ring is empty");
}
final E result = _ring._backingList.remove(_position);
if (_position >= _ring._backingList.size())
{
--_position;
}
++_ring._modCount;
++_expectedModCount;
return result;
}
@Override
public RingCursor cursor()
{
return new ArrayRingCursor(_ring, _position);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy