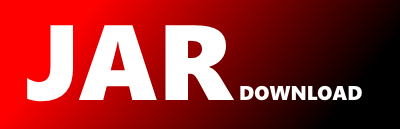
net.sf.javagimmicks.collections8.Lists Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gimmicks Show documentation
Show all versions of gimmicks Show documentation
Utility classes, APIs and tools for Java
package net.sf.javagimmicks.collections8;
import java.util.Collection;
import java.util.List;
import java.util.ListIterator;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
import java.util.function.Predicate;
/**
* Contains some static helper operations for dealing with {@link List}s.
*/
public class Lists
{
private Lists()
{}
/**
* Performs a {@link Collection#forEach(java.util.function.Consumer)}
* operation on a given {@link List} providing the current index as an
* additional parameter - uses a {@link BiConsumer} instead of a
* {@link Consumer} to be able to provide the index.
*
* @param list
* the {@link List} to iterate over
* @param action
* the {@link BiConsumer} that takes the current index and element
* @param
* the type of elements that the {@link List} carries
*/
public static void forEachWithIndex(final List list, final BiConsumer action)
{
for (final ListIterator listIterator = list.listIterator(); listIterator.hasNext();)
{
action.accept(listIterator.nextIndex(), listIterator.next());
}
}
/**
* Performs a filtered
* {@link Collection#forEach(java.util.function.Consumer)} operation on a
* given {@link List} providing the current index as an additional parameter
* - uses a {@link BiConsumer} instead of a {@link Consumer} to be able to
* provide the index.
*
* @param list
* the {@link List} to iterate over
* @param filter
* a {@link Predicate} to filter entries on which the
* {@link BiConsumer} should be called
* @param action
* the {@link BiConsumer} that takes the current index and element
* @param
* the type of elements that the {@link List} carries
*/
public static void forEachWithIndex(final List list, final Predicate filter,
final BiConsumer action)
{
for (final ListIterator listIterator = list.listIterator(); listIterator.hasNext();)
{
final int index = listIterator.nextIndex();
final E element = listIterator.next();
if (filter.test(element))
{
action.accept(index, element);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy