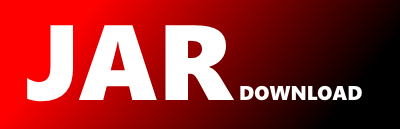
net.sf.javagimmicks.collections8.RingIterator Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gimmicks Show documentation
Show all versions of gimmicks Show documentation
Utility classes, APIs and tools for Java
package net.sf.javagimmicks.collections8;
import java.util.Iterator;
import java.util.NoSuchElementException;
/**
* An implementation of {@link Iterator} that wraps around a given
* {@link RingCursor}.
*
* @param
* the type of elements this {@link RingIterator} operates on
*/
public class RingIterator implements Iterator
{
private final RingCursor _ringCursor;
private final int _size;
private int _counter = 0;
private boolean _removeCalled = true;
/**
* Constructs a new instance around the given {@link RingCursor}.
*
* @param ringCursor
* the {@link RingCursor} over which's elements should be iterated
*/
public RingIterator(final RingCursor ringCursor)
{
_ringCursor = ringCursor;
_ringCursor.previous();
_size = ringCursor.size();
}
@Override
public boolean hasNext()
{
return _counter != _size;
}
@Override
public E next()
{
if (!hasNext())
{
throw new NoSuchElementException();
}
_removeCalled = false;
++_counter;
return _ringCursor.next();
}
@Override
public void remove()
{
if (_removeCalled)
{
throw new IllegalStateException(
"next() has not yet been called since last remove() call or creation of this iterator!");
}
_removeCalled = true;
_ringCursor.remove();
if (!_ringCursor.isEmpty())
{
_ringCursor.previous();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy