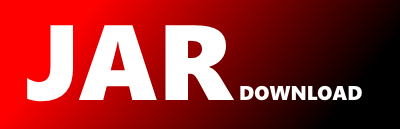
net.sf.javagimmicks.collections8.composite.CompositeEnumeration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gimmicks Show documentation
Show all versions of gimmicks Show documentation
Utility classes, APIs and tools for Java
package net.sf.javagimmicks.collections8.composite;
import java.util.Enumeration;
import java.util.Iterator;
import java.util.List;
import java.util.NoSuchElementException;
class CompositeEnumeration implements Enumeration
{
protected final Iterator> _enumerations;
protected Enumeration _currentEnumeration;
protected Enumeration _nextEnumeration;
CompositeEnumeration(final List> enumerations)
{
_enumerations = enumerations.iterator();
findNextEnumeration();
}
@Override
public boolean hasMoreElements()
{
if (_currentEnumeration != null && _currentEnumeration.hasMoreElements())
{
return true;
}
findNextEnumeration();
return _nextEnumeration != null && _nextEnumeration.hasMoreElements();
}
@Override
public E nextElement()
{
if (!hasMoreElements())
{
throw new NoSuchElementException();
}
return moveNext();
}
protected void findNextEnumeration()
{
if (_nextEnumeration != null)
{
return;
}
while (_enumerations.hasNext())
{
_nextEnumeration = _enumerations.next();
if (_nextEnumeration.hasMoreElements())
{
break;
}
}
}
protected E moveNext()
{
if (_nextEnumeration != null)
{
_currentEnumeration = _nextEnumeration;
_nextEnumeration = null;
}
return _currentEnumeration.nextElement();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy