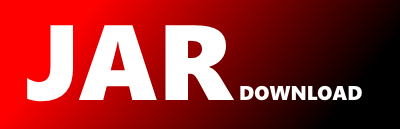
net.sf.javagimmicks.collections8.event.AbstractEventMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gimmicks Show documentation
Show all versions of gimmicks Show documentation
Utility classes, APIs and tools for Java
package net.sf.javagimmicks.collections8.event;
import java.util.Iterator;
import java.util.Map;
import java.util.Set;
import net.sf.javagimmicks.collections8.decorators.AbstractEntryDecorator;
import net.sf.javagimmicks.collections8.decorators.AbstractIteratorDecorator;
import net.sf.javagimmicks.collections8.decorators.AbstractUnmodifiableMapDecorator;
/**
* A base {@link Map} wrapper that reports changes to internal callback methods
* - these must be overwritten by concrete implementations in order to react in
* any way to the changes.
*
* Methods that must be overwritten:
*
* - {@link #fireEntryAdded(Object, Object)}
* - {@link #fireEntryUpdated(Object, Object, Object)}
* - {@link #fireEntryRemoved(Object, Object)}
*
*/
public abstract class AbstractEventMap extends AbstractUnmodifiableMapDecorator
{
private static final long serialVersionUID = -2690296055006665266L;
/**
* Wraps a new instance around a given {@link Map}
*
* @param decorated
* the {@link Map} to wrap
*/
public AbstractEventMap(final Map decorated)
{
super(decorated);
}
@Override
public Set> entrySet()
{
return new EventMapEntrySet(getDecorated().entrySet());
}
@Override
public V put(final K key, final V value)
{
final boolean isUpdate = containsKey(key);
final V oldValue = getDecorated().put(key, value);
if (isUpdate)
{
fireEntryUpdated(key, oldValue, value);
}
else
{
fireEntryAdded(key, value);
}
return oldValue;
}
abstract protected void fireEntryAdded(K key, V value);
abstract protected void fireEntryUpdated(K key, V oldValue, V newValue);
abstract protected void fireEntryRemoved(K key, V value);
protected class EventMapEntrySet extends AbstractEventSet>
{
private static final long serialVersionUID = 4496963842926801525L;
protected EventMapEntrySet(final Set> decorated)
{
super(decorated);
}
@Override
public Iterator> iterator()
{
return new AbstractIteratorDecorator>(super.iterator())
{
@Override
public Entry next()
{
return new AbstractEntryDecorator(super.next())
{
private static final long serialVersionUID = -6377534237333144069L;
@Override
public V setValue(final V value)
{
final V oldValue = super.setValue(value);
fireEntryUpdated(getKey(), oldValue, value);
return oldValue;
}
};
}
};
}
@Override
public boolean add(final Entry e)
{
throw new UnsupportedOperationException("Cannot directly add entries to the EntrySet of an AbstractEventMap!");
}
@Override
protected void fireElementAdded(final Entry element)
{}
@Override
protected void fireElementReadded(final Entry element)
{}
@Override
protected void fireElementRemoved(final Entry element)
{
fireEntryRemoved(element.getKey(), element.getValue());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy