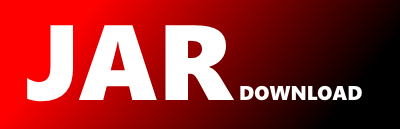
net.sf.javagimmicks.collections8.event.AbstractEventNavigableMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gimmicks Show documentation
Show all versions of gimmicks Show documentation
Utility classes, APIs and tools for Java
package net.sf.javagimmicks.collections8.event;
import java.util.NavigableMap;
import java.util.NavigableSet;
import net.sf.javagimmicks.collections8.decorators.AbstractEntryDecorator;
/**
* A base {@link NavigableMap} wrapper that reports changes to internal callback
* methods - these must be overwritten by concrete implementations in order to
* react in any way to the changes.
*
* Methods that must be overwritten:
*
* - {@link #fireEntryAdded(Object, Object)}
* - {@link #fireEntryUpdated(Object, Object, Object)}
* - {@link #fireEntryRemoved(Object, Object)}
*
*/
public abstract class AbstractEventNavigableMap extends AbstractEventSortedMap implements
NavigableMap
{
private static final long serialVersionUID = 7570207692375842675L;
/**
* Wraps a new instance around a given {@link NavigableMap}
*
* @param decorated
* the {@link NavigableMap} to wrap
*/
public AbstractEventNavigableMap(final NavigableMap decorated)
{
super(decorated);
}
@Override
public NavigableMap getDecorated()
{
return (NavigableMap) super.getDecorated();
}
@Override
public Entry ceilingEntry(final K key)
{
return new EventEntry(this, getDecorated().ceilingEntry(key));
}
@Override
public K ceilingKey(final K key)
{
return getDecorated().ceilingKey(key);
}
@Override
public Entry firstEntry()
{
return new EventEntry(this, getDecorated().firstEntry());
}
@Override
public Entry floorEntry(final K key)
{
return new EventEntry(this, getDecorated().floorEntry(key));
}
@Override
public K floorKey(final K key)
{
return getDecorated().floorKey(key);
}
@Override
public Entry higherEntry(final K key)
{
return new EventEntry(this, getDecorated().higherEntry(key));
}
@Override
public K higherKey(final K key)
{
return getDecorated().higherKey(key);
}
@Override
public Entry lastEntry()
{
return new EventEntry(this, getDecorated().lastEntry());
}
@Override
public Entry lowerEntry(final K key)
{
return new EventEntry(this, getDecorated().lowerEntry(key));
}
@Override
public K lowerKey(final K key)
{
return getDecorated().lowerKey(key);
}
@Override
public Entry pollFirstEntry()
{
final Entry firstEntry = getDecorated().pollFirstEntry();
if (firstEntry != null)
{
fireEntryRemoved(firstEntry.getKey(), firstEntry.getValue());
}
return firstEntry;
}
@Override
public Entry pollLastEntry()
{
final Entry lastEntry = getDecorated().pollLastEntry();
if (lastEntry != null)
{
fireEntryRemoved(lastEntry.getKey(), lastEntry.getValue());
}
return lastEntry;
}
@Override
public NavigableSet descendingKeySet()
{
return descendingMap().navigableKeySet();
}
@Override
public NavigableSet navigableKeySet()
{
return new NavigableMapKeySetDecorator(this);
}
@Override
public NavigableMap descendingMap()
{
return new EventSubNavigableMap(this, getDecorated().descendingMap());
}
@Override
public NavigableMap headMap(final K toKey, final boolean inclusive)
{
return new EventSubNavigableMap(this, getDecorated().headMap(toKey, inclusive));
}
@Override
public NavigableMap subMap(final K fromKey, final boolean fromInclusive, final K toKey,
final boolean toInclusive)
{
return new EventSubNavigableMap(this, getDecorated().subMap(fromKey, fromInclusive, toKey, toInclusive));
}
@Override
public NavigableMap tailMap(final K fromKey, final boolean inclusive)
{
return new EventSubNavigableMap(this, getDecorated().tailMap(fromKey, inclusive));
}
protected static class EventSubNavigableMap extends AbstractEventNavigableMap
{
private static final long serialVersionUID = 8445308257944385932L;
protected final AbstractEventNavigableMap _parent;
protected EventSubNavigableMap(final AbstractEventNavigableMap parent, final NavigableMap decorated)
{
super(decorated);
_parent = parent;
}
@Override
protected void fireEntryAdded(final K key, final V value)
{
_parent.fireEntryAdded(key, value);
}
@Override
protected void fireEntryRemoved(final K key, final V value)
{
_parent.fireEntryRemoved(key, value);
}
@Override
protected void fireEntryUpdated(final K key, final V oldValue, final V newValue)
{
_parent.fireEntryUpdated(key, oldValue, newValue);
}
}
protected static class EventEntry extends AbstractEntryDecorator
{
private static final long serialVersionUID = 5721131374089977796L;
protected final AbstractEventNavigableMap _parent;
public EventEntry(final AbstractEventNavigableMap parent, final Entry decorated)
{
super(decorated);
_parent = parent;
}
@Override
public V setValue(final V value)
{
final V oldValue = super.setValue(value);
_parent.fireEntryUpdated(getKey(), oldValue, value);
return oldValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy