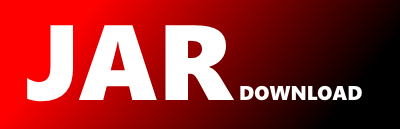
net.sf.javagimmicks.collections8.transformer.BidiTransformingNavigableSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gimmicks Show documentation
Show all versions of gimmicks Show documentation
Utility classes, APIs and tools for Java
package net.sf.javagimmicks.collections8.transformer;
import java.util.Iterator;
import java.util.NavigableSet;
import net.sf.javagimmicks.transform8.BidiFunction;
class BidiTransformingNavigableSet
extends BidiTransformingSortedSet
implements NavigableSet
{
BidiTransformingNavigableSet(NavigableSet set, BidiFunction transformer)
{
super(set, transformer);
}
public T ceiling(T e)
{
F ceiling = getNavigableSet().ceiling(transformBack(e));
return ceiling != null ? transform(ceiling) : null;
}
public Iterator descendingIterator()
{
return TransformerUtils.decorate(
getNavigableSet().descendingIterator(),
getTransformerFunction());
}
public NavigableSet descendingSet()
{
return TransformerUtils.decorate(
getNavigableSet().descendingSet(),
getTransformerBidiFunction());
}
public T floor(T e)
{
F floor = getNavigableSet().floor(transformBack(e));
return floor != null ? transform(floor) : null;
}
public NavigableSet headSet(T toElement, boolean inclusive)
{
return TransformerUtils.decorate(
getNavigableSet().headSet(transformBack(toElement), inclusive),
getTransformerBidiFunction());
}
public T higher(T e)
{
F higher = getNavigableSet().higher(transformBack(e));
return higher != null ? transform(higher) : null;
}
public T lower(T e)
{
F lower = getNavigableSet().lower(transformBack(e));
return lower != null ? transform(lower) : null;
}
public T pollFirst()
{
F first = getNavigableSet().pollFirst();
return first != null ? transform(first) : null;
}
public T pollLast()
{
F last = getNavigableSet().pollLast();
return last != null ? transform(last) : null;
}
public NavigableSet subSet(T fromElement, boolean fromInclusive,
T toElement, boolean toInclusive)
{
return TransformerUtils.decorate(
getNavigableSet().subSet(
transformBack(fromElement), fromInclusive,
transformBack(toElement), toInclusive),
getTransformerBidiFunction());
}
public NavigableSet tailSet(T fromElement, boolean inclusive)
{
return TransformerUtils.decorate(
getNavigableSet().tailSet(transformBack(fromElement), inclusive),
getTransformerBidiFunction());
}
protected NavigableSet getNavigableSet()
{
return (NavigableSet) _internalSet;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy