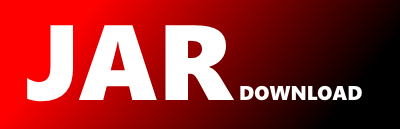
net.sf.javagimmicks.collections8.transformer.TransformerUtils Maven / Gradle / Ivy
Show all versions of gimmicks Show documentation
package net.sf.javagimmicks.collections8.transformer;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Comparator;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.List;
import java.util.ListIterator;
import java.util.Map;
import java.util.NavigableMap;
import java.util.NavigableSet;
import java.util.Set;
import java.util.SortedMap;
import java.util.SortedSet;
import java.util.Spliterator;
import java.util.TreeMap;
import java.util.TreeSet;
import java.util.function.Consumer;
import java.util.function.Function;
import net.sf.javagimmicks.collections8.Ring;
import net.sf.javagimmicks.collections8.RingCursor;
import net.sf.javagimmicks.transform8.BidiFunction;
/**
* This class is the central entry point to the JavaGimmicks transforming
* collections API by providing decorator- and generator methods for many
* transforming types.
*
* A more detailed description of the API can be found at in the package
* description {@link net.sf.javagimmicks.collections8.transformer}.
*
* @see net.sf.javagimmicks.collections8.transformer
* @author Michael Scholz
*/
public class TransformerUtils
{
private TransformerUtils()
{}
/**
* Wraps a new transforming {@link Consumer} using the given {@link Function}
* around a given {@link Consumer}.
*
* @param consumer
* the {@link Consumer} to wrap around
* @param transformer
* the {@link Function} to use for wrapping
* @param
* the "from" or source type
* @param
* the "to" or target type
* @return the transforming wrapped {@link Consumer}
*/
public static Consumer decorate(final Consumer super T> consumer,
final Function transformer)
{
return new TransformingConsumer(consumer, transformer);
}
/**
* Wraps a new transforming {@link Comparator} using the given
* {@link Function} around a given {@link Comparator}.
*
* @param comparator
* the {@link Comparator} to wrap around
* @param transformer
* the {@link Function} to use for wrapping
* @param
* the "from" or source type
* @param
* the "to" or target type
* @return the transforming wrapped {@link Comparator}
*/
public static Comparator super T> decorate(final Comparator super F> comparator,
final Function transformer)
{
return new TransformingComparator(comparator, transformer);
}
/**
* Wraps a new transforming {@link Iterator} using the given {@link Function}
* around a given {@link Iterator}.
*
* For a list of available operations see package description.
*
* @param iterator
* the {@link Iterator} to wrap around
* @param transformer
* the {@link Function} to use for wrapping
* @param
* the "from" or source type
* @param
* the "to" or target type
* @return the transforming wrapped {@link Iterator}
*/
public static Iterator decorate(final Iterator iterator, final Function transformer)
{
return new TransformingIterator(iterator, transformer);
}
/**
* Wraps a new transforming {@link Spliterator} using the given
* {@link Function} around a given {@link Spliterator}.
*
* For a list of available operations see package description.
*
* @param spliterator
* the {@link Spliterator} to wrap around
* @param transformer
* the {@link Function} to use for wrapping
* @param
* the "from" or source type
* @param
* the "to" or target type
* @return the transforming wrapped {@link Spliterator}
*/
public static Spliterator decorate(final Spliterator spliterator, final Function transformer)
{
return new TransformingSpliterator(spliterator, transformer);
}
/**
* Wraps a new transforming {@link Spliterator} using the given
* {@link BidiFunction} around a given {@link Spliterator}.
*
* For a list of available operations see package description.
*
* @param spliterator
* the {@link Spliterator} to wrap around
* @param transformer
* the {@link BidiFunction} to use for wrapping
* @param
* the "from" or source type
* @param
* the "to" or target type
* @return the transforming wrapped {@link Spliterator}
*/
public static Spliterator decorate(final Spliterator spliterator, final BidiFunction transformer)
{
return new TransformingSpliterator(spliterator, transformer);
}
/**
* Wraps a new transforming {@link ListIterator} using the given
* {@link Function} around a given {@link ListIterator}.
*
* For a list of available operations see package description.
*
* @param iterator
* the {@link ListIterator} to wrap around
* @param transformer
* the {@link Function} to use for wrapping
* @param
* the "from" or source type
* @param
* the "to" or target type
* @return the transforming wrapped {@link ListIterator}
*/
public static ListIterator decorate(final ListIterator iterator, final Function transformer)
{
return new TransformingListIterator(iterator, transformer);
}
/**
* Wraps a new transforming {@link ListIterator} using the given
* {@link BidiFunction} around a given {@link ListIterator}.
*
* For a list of available operations see package description.
*
* @param iterator
* the {@link ListIterator} to wrap around
* @param transformer
* the {@link BidiFunction} to use for wrapping
* @param
* the "from" or source type
* @param
* the "to" or target type
* @return the transforming wrapped {@link ListIterator}
*/
public static ListIterator decorate(final ListIterator iterator, final BidiFunction transformer)
{
return new BidiTransformingListIterator(iterator, transformer);
}
/**
* Wraps a new transforming {@link Collection} using the given
* {@link Function} around a given {@link Collection}.
*
* For a list of available operations see package description.
*
* @param collection
* the {@link Collection} to wrap around
* @param transformer
* the {@link Function} to use for wrapping
* @param
* the "from" or source type
* @param
* the "to" or target type
* @return the transforming wrapped {@link Collection}
*/
public static Collection decorate(final Collection collection, final Function transformer)
{
return new TransformingCollection(collection, transformer);
}
/**
* Wraps a new transforming {@link Collection} using the given
* {@link BidiFunction} around a given {@link Collection}.
*
* For a list of available operations see package description.
*
* @param collection
* the {@link Collection} to wrap around
* @param transformer
* the {@link BidiFunction} to use for wrapping
* @param
* the "from" or source type
* @param
* the "to" or target type
* @return the transforming wrapped {@link Collection}
*/
public static Collection decorate(final Collection collection, final BidiFunction transformer)
{
return new BidiTransformingCollection(collection, transformer);
}
/**
* Wraps a new transforming {@link Set} using the given {@link Function}
* around a given {@link Set}.
*
* For a list of available operations see package description.
*
* @param set
* the {@link Set} to wrap around
* @param transformer
* the {@link Function} to use for wrapping
* @param
* the "from" or source type
* @param
* the "to" or target type
* @return the transforming wrapped {@link Set}
*/
public static Set decorate(final Set set, final Function transformer)
{
return new TransformingSet(set, transformer);
}
/**
* Wraps a new transforming {@link Set} using the given {@link BidiFunction}
* around a given {@link Set}.
*
* For a list of available operations see package description.
*
* @param set
* the {@link Set} to wrap around
* @param transformer
* the {@link BidiFunction} to use for wrapping
* @param
* the "from" or source type
* @param
* the "to" or target type
* @return the transforming wrapped {@link Set}
*/
public static Set decorate(final Set set, final BidiFunction transformer)
{
return new BidiTransformingSet(set, transformer);
}
/**
* Wraps a new transforming {@link SortedSet} using the given
* {@link Function} around a given {@link SortedSet}.
*
* For a list of available operations see package description.
*
* @param set
* the {@link SortedSet} to wrap around
* @param transformer
* the {@link Function} to use for wrapping
* @param
* the "from" or source type
* @param
* the "to" or target type
* @return the transforming wrapped {@link SortedSet}
*/
public static SortedSet decorate(final SortedSet set, final Function transformer)
{
return new TransformingSortedSet(set, transformer);
}
/**
* Wraps a new transforming {@link SortedSet} using the given
* {@link BidiFunction} around a given {@link SortedSet}.
*
* For a list of available operations see package description.
*
* @param set
* the {@link SortedSet} to wrap around
* @param transformer
* the {@link BidiFunction} to use for wrapping
* @param
* the "from" or source type
* @param
* the "to" or target type
* @return the transforming wrapped {@link SortedSet}
*/
public static SortedSet decorate(final SortedSet set, final BidiFunction transformer)
{
return new BidiTransformingSortedSet(set, transformer);
}
/**
* Wraps a new transforming {@link NavigableSet} using the given
* {@link Function} around a given {@link NavigableSet}.
*
* For a list of available operations see package description.
*
* @param set
* the {@link NavigableSet} to wrap around
* @param transformer
* the {@link Function} to use for wrapping
* @param
* the "from" or source type
* @param
* the "to" or target type
* @return the transforming wrapped {@link NavigableSet}
*/
public static NavigableSet decorate(final NavigableSet set, final Function transformer)
{
return new TransformingNavigableSet(set, transformer);
}
/**
* Wraps a new transforming {@link NavigableSet} using the given
* {@link BidiFunction} around a given {@link NavigableSet}.
*
* For a list of available operations see package description.
*
* @param set
* the {@link NavigableSet} to wrap around
* @param transformer
* the {@link BidiFunction} to use for wrapping
* @param
* the "from" or source type
* @param
* the "to" or target type
* @return the transforming wrapped {@link NavigableSet}
*/
public static NavigableSet decorate(final NavigableSet set, final BidiFunction transformer)
{
return new BidiTransformingNavigableSet(set, transformer);
}
/**
* Wraps a new transforming {@link List} using the given {@link Function}
* around a given {@link List}.
*
* For a list of available operations see package description.
*
* @param list
* the {@link List} to wrap around
* @param transformer
* the {@link Function} to use for wrapping
* @param
* the "from" or source type
* @param
* the "to" or target type
* @return the transforming wrapped {@link List}
*/
public static List decorate(final List list, final Function transformer)
{
return new TransformingList(list, transformer);
}
/**
* Wraps a new transforming {@link List} using the given {@link BidiFunction}
* around a given {@link List}.
*
* For a list of available operations see package description.
*
* @param list
* the {@link List} to wrap around
* @param transformer
* the {@link BidiFunction} to use for wrapping
* @param
* the "from" or source type
* @param
* the "to" or target type
* @return the transforming wrapped {@link List}
*/
public static List decorate(final List list, final BidiFunction transformer)
{
return new BidiTransformingList(list, transformer);
}
/**
* Wraps a new key-transforming {@link Map} using the given {@link Function}
* around a given {@link Map}.
*
* For a list of available operations see package description.
*
* @param map
* the {@link Map} to wrap around
* @param transformer
* the {@link Function} to use for wrapping
* @param
* the "from" or source key type
* @param
* the "to" or target key type
* @param
* the type of the values
* @return the key-transforming wrapped {@link Map}
*/
public static Map decorateKeyBased(final Map map, final Function transformer)
{
return new KeyTransformingMap(map, transformer);
}
/**
* Wraps a new key-transforming {@link Map} using the given
* {@link BidiFunction} around a given {@link Map}.
*
* For a list of available operations see package description.
*
* @param map
* the {@link Map} to wrap around
* @param transformer
* the {@link BidiFunction} to use for wrapping
* @param
* the "from" or source key type
* @param
* the "to" or target key type
* @param
* the type of the values
* @return the key-transforming wrapped {@link Map}
*/
public static Map decorateKeyBased(final Map map,
final BidiFunction transformer)
{
return new KeyBidiTransformingMap(map, transformer);
}
/**
* Wraps a new key-transforming {@link SortedMap} using the given
* {@link Function} around a given {@link SortedMap}.
*
* For a list of available operations see package description.
*
* @param map
* the {@link SortedMap} to wrap around
* @param transformer
* the {@link Function} to use for wrapping
* @param
* the "from" or source key type
* @param
* the "to" or target key type
* @param
* the type of the values
* @return the key-transforming wrapped {@link SortedMap}
*/
public static SortedMap decorateKeyBased(final SortedMap map,
final Function transformer)
{
return new KeyTransformingSortedMap(map, transformer);
}
/**
* Wraps a new key-transforming {@link SortedMap} using the given
* {@link BidiFunction} around a given {@link SortedMap}.
*
* For a list of available operations see package description.
*
* @param map
* the {@link SortedMap} to wrap around
* @param transformer
* the {@link BidiFunction} to use for wrapping
* @param
* the "from" or source key type
* @param
* the "to" or target key type
* @param
* the type of the values
* @return the key-transforming wrapped {@link SortedMap}
*/
public static SortedMap decorateKeyBased(final SortedMap map,
final BidiFunction transformer)
{
return new KeyBidiTransformingSortedMap(map, transformer);
}
/**
* Wraps a new key-transforming {@link NavigableMap} using the given
* {@link Function} around a given {@link NavigableMap}.
*
* For a list of available operations see package description.
*
* @param map
* the {@link NavigableMap} to wrap around
* @param transformer
* the {@link Function} to use for wrapping
* @param
* the "from" or source key type
* @param
* the "to" or target key type
* @param
* the type of the values
* @return the key-transforming wrapped {@link NavigableMap}
*/
public static NavigableMap decorateKeyBased(final NavigableMap map,
final Function transformer)
{
return new KeyTransformingNavigableMap(map, transformer);
}
/**
* Wraps a new key-transforming {@link NavigableMap} using the given
* {@link BidiFunction} around a given {@link NavigableMap}.
*
* For a list of available operations see package description.
*
* @param map
* the {@link NavigableMap} to wrap around
* @param transformer
* the {@link BidiFunction} to use for wrapping
* @param
* the "from" or source key type
* @param
* the "to" or target key type
* @param
* the type of the values
* @return the key-transforming wrapped {@link NavigableMap}
*/
public static NavigableMap decorateKeyBased(final NavigableMap map,
final BidiFunction transformer)
{
return new KeyBidiTransformingNavigableMap(map, transformer);
}
/**
* Wraps a new value-transforming {@link Map} using the given
* {@link Function} around a given {@link Map}.
*
* For a list of available operations see package description.
*
* @param map
* the {@link Map} to wrap around
* @param transformer
* the {@link Function} to use for wrapping
* @param
* the type of the keys
* @param
* the "from" or source type of the values
* @param
* the "to" or target type of the values
* @return the value-transforming wrapped {@link Map}
*/
public static Map decorateValueBased(final Map map,
final Function transformer)
{
return new ValueTransformingMap(map, transformer);
}
/**
* Wraps a new value-transforming {@link Map} using the given
* {@link BidiFunction} around a given {@link Map}.
*
* For a list of available operations see package description.
*
* @param map
* the {@link Map} to wrap around
* @param transformer
* the {@link BidiFunction} to use for wrapping
* @param
* the type of the keys
* @param
* the "from" or source type of the values
* @param
* the "to" or target type of the values
* @return the value-transforming wrapped {@link Map}
*/
public static Map decorateValueBased(final Map map,
final BidiFunction transformer)
{
return new ValueBidiTransformingMap(map, transformer);
}
/**
* Wraps a new value-transforming {@link SortedMap} using the given
* {@link Function} around a given {@link SortedMap}.
*
* For a list of available operations see package description.
*
* @param map
* the {@link SortedMap} to wrap around
* @param transformer
* the {@link Function} to use for wrapping
* @param
* the type of the keys
* @param
* the "from" or source type of the values
* @param
* the "to" or target type of the values
* @return the value-transforming wrapped {@link SortedMap}
*/
public static SortedMap decorateValueBased(final SortedMap map,
final Function transformer)
{
return new ValueTransformingSortedMap(map, transformer);
}
/**
* Wraps a new value-transforming {@link SortedMap} using the given
* {@link BidiFunction} around a given {@link SortedMap}.
*
* For a list of available operations see package description.
*
* @param map
* the {@link SortedMap} to wrap around
* @param transformer
* the {@link BidiFunction} to use for wrapping
* @param
* the type of the keys
* @param
* the "from" or source type of the values
* @param
* the "to" or target type of the values
* @return the value-transforming wrapped {@link SortedMap}
*/
public static SortedMap decorateValueBased(final SortedMap map,
final BidiFunction transformer)
{
return new ValueBidiTransformingSortedMap(map, transformer);
}
/**
* Wraps a new value-transforming {@link NavigableMap} using the given
* {@link Function} around a given {@link NavigableMap}.
*
* For a list of available operations see package description.
*
* @param map
* the {@link NavigableMap} to wrap around
* @param transformer
* the {@link Function} to use for wrapping
* @param
* the type of the keys
* @param
* the "from" or source type of the values
* @param
* the "to" or target type of the values
* @return the value-transforming wrapped {@link NavigableMap}
*/
public static NavigableMap decorateValueBased(final NavigableMap map,
final Function transformer)
{
return new ValueTransformingNavigableMap(map, transformer);
}
/**
* Wraps a new value-transforming {@link NavigableMap} using the given
* {@link BidiFunction} around a given {@link NavigableMap}.
*
* For a list of available operations see package description.
*
* @param map
* the {@link NavigableMap} to wrap around
* @param transformer
* the {@link BidiFunction} to use for wrapping
* @param
* the type of the keys
* @param
* the "from" or source type of the values
* @param
* the "to" or target type of the values
* @return the value-transforming wrapped {@link NavigableMap}
*/
public static NavigableMap decorateValueBased(final NavigableMap map,
final BidiFunction transformer)
{
return new ValueBidiTransformingNavigableMap(map, transformer);
}
/**
* Wraps a new key- and value-transforming {@link Map} using the given key-
* {@link Function} and value-{@link Function} around a given {@link Map}.
*
* For a list of available operations see package description.
*
* @param map
* the {@link Map} to wrap around
* @param keyFunction
* the key-{@link Function} to used for wrapping
* @param valueFunction
* the key-{@link Function} to used for wrapping
* @param
* the "from" or source key type
* @param
* the "to" or target key type
* @param
* the "from" or source type of the values
* @param
* the "to" or target type of the values
* @return the key-and value-transforming wrapped {@link Map}
*/
public static Map decorate(final Map map, final Function keyFunction,
final Function valueFunction)
{
final Map