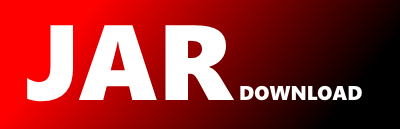
net.sf.javagimmicks.collections8.transformer.package-info Maven / Gradle / Ivy
Show all versions of gimmicks Show documentation
/**
* This package spans up the JavaGimmicks
* transformation API which allows to decorate
* collections with transforming views that apply
* a specified transformation logic on access to all
* elements.
*
* Let's use the terms
* internal format and external format for the
* two element formats (original and view).
*
* For example, you can create a {@code List} which is
* actually internally backed by a {@code List}. You
* wont't be aware of this fact and will think to work with
* a {@code List}. You can add, remove, get or set
* elements and of course iterate over them. The instance
* you are working on will automatically take care of transforming
* between the respective element types {@link java.lang.String} and
* {@link java.lang.Integer} and the results of all operations will be
* immediately visible in the internal {@link java.util.List}.
*
* Additionally, you could also think of a {@code List}
* which internally works with another {@code List}
* but keeps the elements in the reverse character order (for
* what reasons ever you like).
*
* Of course, in order to be able to work with transforming
* instances, you need to provide to them the transformation rules.
* You can do so by writing a class that implements the
* {@link java.util.function.Function} or
* {@link net.sf.javagimmicks.transform8.BidiFunction} interface
* which are both contained in this API.
*
* When providing a {@link java.util.function.Function},
* you will only be able to perform operations on the transforming instance that
* do not need to convert from the external format to the internal
* format. For example, you will be able to iterate over a
* {@link java.util.List}, get elements, remove them or ask if they are
* contained inside. But you will not be able to set or add elements
* (neither on the {@link java.util.List} nor on any of its
* {@link java.util.ListIterator}s. For more details, see below.
*
* When providing a {@link net.sf.javagimmicks.transform8.BidiFunction},
* all operations will be available and some operations will even be faster.
*
*
Decorator methods in {@link net.sf.javagimmicks.collections8.transformer.TransformerUtils}
* This class provides a large number of methods {@code decorate()}
* (and {@code decorateKeyBased()} and {@code decorateValueBased()}
* for {@link java.util.Map}s) which can be used to create transformed instances
* of a number of (generic) base classes by providing an instance of this
* base class and one of {@link java.util.function.Function} or
* {@link net.sf.javagimmicks.transform8.BidiFunction}.
*
* The resulting instance will implement the same interface (but
* eventually with different type parameters) and additionally
* {@link net.sf.javagimmicks.transform8.Transforming} or
* {@link net.sf.javagimmicks.transform8.BidiTransforming}
* (both depending on the provided transformation rule instance).
* Furthermore, this instance
* is completely backed on the the provided one and will contain
* no data itself. Any changes on it will affect the internal one and
* vice versa.
*
*
*
Operations on a transformed {@link java.util.function.Consumer}
*
*
*
* Operation
* Function
*
*
*
*
* accept()
* supported
*
*
*
*
* andThen()
* supported
*
*
*
*
* Operations on a transformed {@link java.util.Comparator}
*
*
*
* Operation
* Function
*
*
*
*
* compare()
* supported
*
*
*
*
* Operations on a transformed {@link java.util.Iterator}
*
*
*
* Operation
* Function
*
*
*
*
* hasNext()
* supported
*
*
* next()
* supported
*
*
* remove()
* supported
*
*
*
*
*
* Operations on a transformed {@link java.util.Spliterator}
*
*
*
* Operation
* Function
* BidiFunction
*
*
*
*
* tryAdvance()
* supported
*
*
* trySplit()
* supported
*
*
* estimatedSize()
* supported
*
*
* characteristics()
* supported
*
*
* hasCharacteristics()
* supported
*
*
* forEachRemaining()
* supported
*
*
* getExactSizeIfKnown()
* supported
*
*
* getComparator()
* not supported
* supported
*
*
*
*
*
* Operations on a transformed {@link java.util.Collection}
*
*
*
* Operation
* Function
* BidiFunction
*
*
*
*
* add()
* not supported
* supported
*
*
* addAll()
* not supported
* supported
*
*
* clear()
* supported
*
*
* contains()
* supported; needs {@code equals()} method
*
*
* containsAll()
* supported; needs {@code equals()} method
*
*
* isEmpty()
* supported
*
*
* iterator()
* supported; See {@code Iterator} operations
*
*
* remove()
* supported; needs {@code equals()} method
*
*
* removeAll()
* supported; needs {@code equals()} method
*
*
* retainAll()
* supported; needs {@code equals()} method
*
*
* size()
* supported
*
*
* toArray()
* supported
*
*
*
*
*
* Operations on a transformed {@link java.util.Set}
*
*
*
* Operation
* Function
* BidiFunction
*
*
*
*
* add()
* not supported
* supported
*
*
* addAll()
* not supported
* supported
*
*
* clear()
* supported
*
*
* contains()
* supported; needs {@code equals()} method
* supported; faster
*
*
* containsAll()
* supported; needs {@code equals()} method
* supported; faster
*
*
* isEmpty()
* supported
*
*
* iterator()
* supported; See {@code Iterator} operations
*
*
* remove()
* supported; needs {@code equals()} method
* supported; faster
*
*
* removeAll()
* supported; needs {@code equals()} method
* supported; faster
*
*
* retainAll()
* supported; needs {@code equals()} method
* supported; faster
*
*
* size()
* supported
*
*
* toArray()
* supported
*
*
*
*
*
* Operations on a transformed {@link java.util.SortedSet}
* Note: the sorting order of a transformed {@link java.util.SortedSet}
* remains that from the wrapped one
*
*
*
* Operation
* Function
* BidiFunction
*
*
*
*
* {@link java.util.Set} operations
* See table for {@code Set} operations
*
*
* comparator()
* not supported
* supported
*
*
* first()
* supported
*
*
* headSet()
* not supported
* supported
*
*
* last()
* supported
*
*
* subSet()
* not supported
* supported
*
*
* tailSet()
* not supported
* supported
*
*
*
*
*
* Operations on a transformed {@link java.util.NavigableSet}
* Note: the sorting order of a transformed {@link java.util.NavigableSet}
* remains that from the wrapped one
*
*
*
* Operation
* Function
* BidiFunction
*
*
*
*
* {@link java.util.Set} operations
* See table for {@code Set} operations
*
*
* {@link java.util.SortedSet} operations
* See table for {@code SortedSet} operations
*
*
* ceiling()
* not supported
* supported
*
*
* descendingIterator()
* supported
*
*
* descendingSet()
* supported
*
*
* floor()
* not supported
* supported
*
*
* headSet()
* not supported
* supported
*
*
* higher()
* not supported
* supported
*
*
* lower()
* not supported
* supported
*
*
* pollFirst()
* supported
*
*
* pollLast()
* supported
*
*
* subSet()
* not supported
* supported
*
*
* tailSet()
* not supported
* supported
*
*
*
*
*
* Operations on a transformed {@link java.util.ListIterator}
*
*
*
* Operation
* Function
* BidiFunction
*
*
*
*
* {@link java.util.Iterator} operations
* See table for {@code Iterator} operations
*
*
* add()
* not supported
* supported
*
*
* hasPrevious()
* supported
*
*
* nextIndex()
* supported
*
*
* previous()
* supported
*
*
* previousIndex()
* supported
*
*
* set()
* not supported
* supported
*
*
*
*
*
* Operations on a transformed {@link java.util.List}
*
*
*
* Operation
* Function
* BidiFunction
*
*
*
*
* {@link java.util.Collection} operations
* See table for {@code Collection} operations
*
*
* add()
* not supported
* supported
*
*
* addAll()
* not supported
* supported
*
*
* get()
* supported
*
*
* indexOf()
* supported; needs {@code equals()} method
*
*
* lastIndexOf()
* supported; needs {@code equals()} method
*
*
* listIterator()
* supported; See {@code ListIterator} operations
*
*
* remove()
* supported
*
*
* set()
* not supported
* supported
*
*
* subList()
* supported (with same behaviour)
*
*
*
*
*
* Operations on a key-based transformed {@link java.util.Map}
*
*
*
* Operation
* Function
* BidiFunction
*
*
*
*
* isEmpty()
* supported
*
*
* size()
* supported
*
*
* put()
* not supported
* supported
*
*
* putAll()
* not supported
* supported
*
*
* get()
* supported; keys need {@code equals()} method
* supported; faster
*
*
* containsKey()
* supported; keys need {@code equals()} method
* supported; faster
*
*
* containsValue()
* supported; values need {@code equals()} method
*
*
* remove()
* supported; keys need {@code equals()} method
* supported; faster
*
*
* clear()
* supported
*
*
* keySet()
* See table for {@code Set} operations
*
*
* values()
* supported
*
*
* entrySet()
* See table for {@code Set} operations
*
*
* Entry.getKey()
* supported
*
*
* Entry.getValue()
* supported
*
*
* Entry.setValue()
* supported
*
*
*
*
*
* Operations on a key-based transformed {@link java.util.SortedMap}
* Note: the sorting order of a transformed {@link java.util.SortedMap}
* remains that from the wrapped one
*
*
*
* Operation
* Function
* BidiFunction
*
*
*
*
* {@link java.util.Map} operations
* See table for {@code Map} operations
*
*
* comparator()
* not supported
* supported
*
*
* firstKey()
* supported
*
*
* headMap()
* not supported
* supported
*
*
* lastKey()
* supported
*
*
* subMap()
* not supported
* supported
*
*
* tailMap()
* not supported
* supported
*
*
*
*
*
* Operations on a key-based transformed {@link java.util.NavigableMap}
* Note: the sorting order of a transformed {@link java.util.NavigableMap}
* remains that from the wrapped one
*
*
*
* Operation
* Function
* BidiFunction
*
*
*
*
* {@link java.util.Map} operations
* See table for {@code Map} operations
*
*
* {@link java.util.SortedMap} operations
* See table for {@code SortedMap} operations
*
*
* ceilingEntry()
* not supported
* supported
*
*
* ceilingKey()
* not supported
* supported
*
*
* descendingKeySet()
* supported; See table for {@code NavigableSet} operations
*
*
* descendingMap()
* supported
*
*
* firstEntry()
* supported
*
*
* floorEntry()
* not supported
* supported
*
*
* floorKey()
* not supported
* supported
*
*
* headMap()
* not supported
* supported
*
*
* higherEntry()
* not supported
* supported
*
*
* higherKey()
* not supported
* supported
*
*
* lastEntry()
* supported
*
*
* lowerEntry()
* not supported
* supported
*
*
* lowerKey()
* not supported
* supported
*
*
* navigableKeySet()
* supported; See table for {@code NavigableSet} operations
*
*
* pollFirstEntry()
* supported
*
*
* pollLastEntry()
* supported
*
*
* subMap()
* not supported
* supported
*
*
* tailMap()
* not supported
* supported
*
*
*
*
*
* Operations on a value-based transformed {@link java.util.Map}
*
*
*
* Operation
* Function
* BidiFunction
*
*
*
*
* isEmpty()
* supported
*
*
* size()
* supported
*
*
* put()
* not supported
* supported
*
*
* putAll()
* not supported
* supported
*
*
* get()
* supported
*
*
* containsKey()
* supported
*
*
* containsValue()
* supported; values need {@code equals()} method
* supported; faster
*
*
* remove()
* supported
*
*
* clear()
* supported
*
*
* keySet()
* supported
*
*
* values()
* See table for {@code Collection} operations
*
*
* entrySet()
* See table for {@code Set} operations
*
*
* Entry.getKey()
* supported
*
*
* Entry.getValue()
* supported
*
*
* Entry.setValue()
* not supported
* supported
*
*
*
*
*
* Operations on a value-based transformed {@link java.util.SortedMap}
*
*
*
* Operation
* Function
* BidiFunction
*
*
*
*
* {@link java.util.Map} operations
* See table for {@code Map} operations
*
*
* comparator()
* supported
*
*
* firstKey()
* supported
*
*
* headMap()
* supported
*
*
* lastKey()
* supported
*
*
* subMap()
* supported
*
*
* tailMap()
* supported
*
*
*
*
*
* Operations on a value-based transformed {@link java.util.NavigableMap}
*
*
*
* Operation
* Function
* BidiFunction
*
*
*
*
* {@link java.util.Map} operations
* See table for {@code Map} operations
*
*
* {@link java.util.SortedMap} operations
* See table for {@code SortedMap} operations
*
*
* ceilingEntry()
* supported
*
*
* ceilingKey()
* supported
*
*
* descendingKeySet()
* supported
*
*
* descendingMap()
* supported
*
*
* firstEntry()
* supported
*
*
* floorEntry()
* supported
*
*
* floorKey()
* supported
*
*
* headMap()
* supported
*
*
* higherEntry()
* supported
*
*
* higherKey()
* supported
*
*
* lastEntry()
* supported
*
*
* lowerEntry()
* supported
*
*
* lowerKey()
* supported
*
*
* navigableKeySet()
* supported
*
*
* pollFirstEntry()
* supported
*
*
* pollLastEntry()
* supported
*
*
* subMap()
* supported
*
*
* tailMap()
* supported
*
*
*
*
*
* Operations on a key- and value-based transformed {@link java.util.Map}
*
* Note: When using a combination of one Function and one BidiFunction
* for keys and values allows you can fall back to the supported operation list
* for the part that only has a Function.
*
* For example a key-transformed and value-bidi-transformed {@link java.util.Map}
* supports the same operations than a purely key-transformed
* {@link java.util.Map}.
*
* Vice-versa example a key-bidi-transformed and value-transformed {@link java.util.Map}
* supports the same operations than a purely value-transformed
* {@link java.util.Map}.
*
*
*
* Operation
* Key- and Value-Function
* Key- and Value-BidiFunction
*
*
*
*
* isEmpty()
* supported
*
*
* size()
* supported
*
*
* put()
* not supported
* supported
*
*
* putAll()
* not supported
* supported
*
*
* get()
* supported; keys need {@code equals()} method
* supported; faster
*
*
* containsKey()
* supported; keys need {@code equals()} method
* supported; faster
*
*
* containsValue()
* supported; values need {@code equals()} method
* supported; faster
*
*
* remove()
* supported; keys need {@code equals()} method
* supported; faster
*
*
* clear()
* supported
*
*
* keySet()
* See table for {@code Set} operations
*
*
* values()
* See table for {@code Collection} operations
*
*
* entrySet()
* See table for {@code Set} operations
*
*
* Entry.getKey()
* supported
*
*
* Entry.getValue()
* supported
*
*
* Entry.setValue()
* not supported
* supported
*
*
*
*
*
* Operations on a key- and value-based transformed {@link java.util.SortedMap}
* Note: the sorting order of a transformed {@link java.util.SortedMap}
* remains that from the wrapped one
*
* Note: When using a combination of one Function and one BidiFunction
* for keys and values allows you can fall back to the supported operation list
* for the part that only has a Function.
*
* For example a key-transformed and value-bidi-transformed {@link java.util.SortedMap}
* supports the same operations than a purely key-transformed
* {@link java.util.SortedMap}.
*
* Vice-versa example a key-bidi-transformed and value-transformed {@link java.util.SortedMap}
* supports the same operations than a purely value-transformed
* {@link java.util.SortedMap}.
*
*
*
* Operation
* Key- and Value-Function
* Key- and Value-BidiFunction
*
*
*
*
* {@link java.util.Map} operations
* See table for {@code Map} operations
*
*
* comparator()
* not supported
* supported
*
*
* firstKey()
* supported
*
*
* headMap()
* not supported
* supported
*
*
* lastKey()
* supported
*
*
* subMap()
* not supported
* supported
*
*
* tailMap()
* not supported
* supported
*
*
*
*
*
* Operations on a key- and value-based transformed {@link java.util.NavigableMap}
* Note: the sorting order of a transformed {@link java.util.NavigableMap}
* remains that from the wrapped one
*
* Note: When using a combination of one Function and one BidiFunction
* for keys and values allows you can fall back to the supported operation list
* for the part that only has a Function.
*
* For example a key-transformed and value-bidi-transformed {@link java.util.NavigableMap}
* supports the same operations than a purely key-transformed
* {@link java.util.NavigableMap}.
*
* Vice-versa example a key-bidi-transformed and value-transformed {@link java.util.NavigableMap}
* supports the same operations than a purely value-transformed
* {@link java.util.NavigableMap}.
*
*
*
* Operation
* Key- and Value-Function
* Key- and Value-BidiFunction
*
*
*
*
* {@link java.util.Map} operations
* See table for {@code Map} operations
*
*
* {@link java.util.SortedMap} operations
* See table for {@code SortedMap} operations
*
*
* ceilingEntry()
* not supported
* supported
*
*
* ceilingKey()
* not supported
* supported
*
*
* descendingKeySet()
* supported; See table for {@code NavigableSet} operations
*
*
* descendingMap()
* supported
*
*
* firstEntry()
* supported
*
*
* floorEntry()
* not supported
* supported
*
*
* floorKey()
* not supported
* supported
*
*
* headMap()
* not supported
* supported
*
*
* higherEntry()
* not supported
* supported
*
*
* higherKey()
* not supported
* supported
*
*
* lastEntry()
* supported
*
*
* lowerEntry()
* not supported
* supported
*
*
* lowerKey()
* not supported
* supported
*
*
* navigableKeySet()
* supported; See table for {@code NavigableSet} operations
*
*
* pollFirstEntry()
* supported
*
*
* pollLastEntry()
* supported
*
*
* subMap()
* not supported
* supported
*
*
* tailMap()
* not supported
* supported
*
*
*
*
*
* Operations on a transformed {@link net.sf.javagimmicks.collections.RingCursor}
*
*
*
* Operation
* Function
* BidiFunction
*
*
*
*
* cursor()
* supported
*
*
* get()
* supported
*
*
* insertAfter()
* not supported
* supported
*
*
* insertBefore()
* not supported
* supported
*
*
* isEmpty()
* supported
*
*
* iterator()
* supported
*
*
* next()
* supported
*
*
* previous()
* supported
*
*
* remove()
* supported
*
*
* set()
* not supported
* supported
*
*
* size()
* supported
*
*
* toList()
* supported
*
*
*
*
*
* Operations on a transformed {@link net.sf.javagimmicks.collections.Ring}
*
*
*
* Operation
* Function
* BidiFunction
*
*
*
*
* {@link java.util.Collection} operations
* See table for {@code Collection} operations
*
*
* cursor()
* supported
*
*
*
*
* @author Michael Scholz
*/
package net.sf.javagimmicks.collections8.transformer;