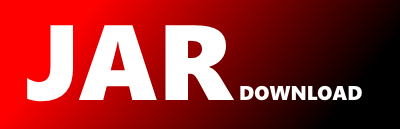
net.sf.javagimmicks.concurrent.locks.DefaultLockRegistry Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gimmicks Show documentation
Show all versions of gimmicks Show documentation
Utility classes, APIs and tools for Java
package net.sf.javagimmicks.concurrent.locks;
import java.io.Serializable;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.TreeMap;
import net.sf.javagimmicks.util.Supplier;
class DefaultLockRegistry implements LockRegistry, Serializable
{
private static final long serialVersionUID = -785304313135882910L;
private static final Supplier> HASHSET_SHARED_COLLECTION_SUPPLIER = new HashSetSharedCollectionSupplier();
public static DefaultLockRegistry createHashBasedInstance()
{
return new DefaultLockRegistry(new HashMap(), new HashMap>(),
HASHSET_SHARED_COLLECTION_SUPPLIER);
}
public static DefaultLockRegistry createTreeBasedInstance()
{
return new DefaultLockRegistry(new TreeMap(), new TreeMap>(),
HASHSET_SHARED_COLLECTION_SUPPLIER);
}
protected final Map _exRegistry;
protected final Map> _shRegistry;
protected final Supplier> _shCollectionSupplier;
public DefaultLockRegistry(final Map exRegistry, final Map> shRegistry,
final Supplier> sharedCollectionFactory)
{
_exRegistry = exRegistry;
_shRegistry = shRegistry;
_shCollectionSupplier = sharedCollectionFactory;
}
@Override
public boolean isSharedFree(final Collection resources)
{
for (final K resource : resources)
{
if (_shRegistry.containsKey(resource))
{
return false;
}
}
return true;
}
@Override
public void registerShared(final Collection resources)
{
final Thread currentThread = Thread.currentThread();
for (final K resource : resources)
{
Collection threadsForResource = _shRegistry.get(resource);
if (threadsForResource == null)
{
threadsForResource = _shCollectionSupplier.get();
_shRegistry.put(resource, threadsForResource);
}
threadsForResource.add(currentThread);
}
}
@Override
public void unregisterShared(final Collection resources)
{
final Thread currentThread = Thread.currentThread();
for (final K resource : resources)
{
final Collection threadsForResource = _shRegistry.get(resource);
if (threadsForResource == null)
{
continue;
}
threadsForResource.remove(currentThread);
if (threadsForResource.isEmpty())
{
_shRegistry.remove(resource);
}
}
}
@Override
public boolean isExclusiveFree(final Collection resources)
{
for (final K resource : resources)
{
if (_exRegistry.containsKey(resource))
{
return false;
}
}
return true;
}
@Override
public void registerExclusive(final Collection resources)
{
final Thread currentThread = Thread.currentThread();
for (final K resource : resources)
{
_exRegistry.put(resource, currentThread);
}
}
@Override
public void unregisterExclusive(final Collection resources)
{
for (final K resource : resources)
{
_exRegistry.remove(resource);
}
}
private static final class HashSetSharedCollectionSupplier implements Supplier>, Serializable
{
private static final long serialVersionUID = 3613642441786733902L;
@Override
public Collection get()
{
return new HashSet();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy