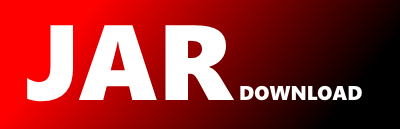
net.sf.javagimmicks.math.MathExt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gimmicks Show documentation
Show all versions of gimmicks Show documentation
Utility classes, APIs and tools for Java
package net.sf.javagimmicks.math;
import java.math.BigInteger;
import net.sf.javagimmicks.math.sequence.FactorialSequence;
/**
* Provides some extension to {@link Math} by providing additional utility
*/
public class MathExt
{
private MathExt()
{}
/**
* Calculates the factorial for a given
* number.
*
* @param base
* the base number of which to calculate the factorial
* @return the resulting factorial
*/
public static BigInteger factorial(final BigInteger base)
{
return FactorialSequence.getValue(base);
}
/**
* Calculates the factorial for a given
* number.
*
* @param base
* the base number of which to calculate the factorial
* @return the resulting factorial
*/
public static BigInteger factorial(final long base)
{
return factorial(BigInteger.valueOf(base));
}
/**
* Calculates the binomial
* coefficient for a given n and k number.
*
* @param n
* the n part of the binomial coefficient
* @param k
* the k part of the binomial coefficient
* @return the resulting binomial coefficient
*/
public static BigInteger binomial(final BigInteger n, final BigInteger k)
{
if (n == null || k == null)
{
throw new IllegalArgumentException("At least one argument was null!");
}
if (n.compareTo(k) < 0)
{
throw new IllegalArgumentException("k was greater than n!");
}
final BigInteger nMinusK = n.subtract(k);
if (nMinusK.compareTo(k) > 0)
{
return binomial(n, nMinusK);
}
BigInteger result = BigInteger.ONE;
for (BigInteger i = k.add(BigInteger.ONE); i.compareTo(n) <= 0; i = i.add(BigInteger.ONE))
{
result = result.multiply(i);
}
result = result.divide(factorial(nMinusK));
return result;
}
/**
* Calculates the binomial
* coefficient for a given n and k number.
*
* @param n
* the n part of the binomial coefficient
* @param k
* the k part of the binomial coefficient
* @return the resulting binomial coefficient
*/
public static BigInteger binomial(final long n, final long k)
{
return binomial(BigInteger.valueOf(n), BigInteger.valueOf(k));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy