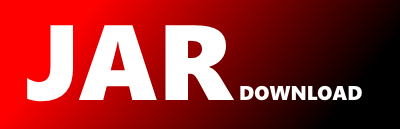
net.sf.javagimmicks.swing.builder.PanelBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gimmicks Show documentation
Show all versions of gimmicks Show documentation
Utility classes, APIs and tools for Java
package net.sf.javagimmicks.swing.builder;
import java.awt.BorderLayout;
import java.awt.FlowLayout;
import java.awt.GridBagLayout;
import java.awt.GridLayout;
import java.awt.LayoutManager;
import javax.swing.Action;
import javax.swing.BoxLayout;
import javax.swing.JButton;
import javax.swing.JComponent;
import javax.swing.JPanel;
/**
* A builder for setting up any {@link JPanel} with a fluent API.
*
* @param
* the type of the parent {@link PanelBuilder} that created this
* {@link PanelBuilder}
*/
public class PanelBuilder> extends ComponentBuilder
{
PanelBuilder(final Parent parentBuilder, final JPanel panel)
{
super(parentBuilder, panel);
}
/**
* Adds a new child {@link JPanel} within the currently built {@link JPanel}
* and returns the {@link PanelBuilder} for it.
*
* @return the {@link PanelBuilder} for the created child {@link JPanel}
*/
public PanelBuilder> panel()
{
return new PanelBuilder>(this, new JPanel());
}
/**
* Adds a new child {@link JPanel} within the currently built {@link JPanel}
* and returns the {@link PanelBuilder} for it.
*
* @param layoutManager
* the {@link LayoutManager} to apply in the created child
* {@link JPanel}
* @return the {@link PanelBuilder} for the created child {@link JPanel}
*/
public PanelBuilder> panel(final LayoutManager layoutManager)
{
return new PanelBuilder>(this, new JPanel(layoutManager));
}
/**
* Applies the given {@link LayoutManager} to the currently built
* {@link JPanel}.
*
* @param layoutManager
* the {@link LayoutManager} to apply
* @return the current builder instance
* @see JPanel#setLayout(LayoutManager)
*/
public PanelBuilder layout(final LayoutManager layoutManager)
{
get().setLayout(layoutManager);
return this;
}
/**
* Applies a new {@link BorderLayout} to the currently built {@link JPanel}.
*
* @return the current builder instance
* @see JPanel#setLayout(LayoutManager)
* @see BorderLayout#BorderLayout()
*/
public PanelBuilder borderLayout()
{
return layout(new BorderLayout());
}
/**
* Applies a new {@link BorderLayout} to the currently built {@link JPanel}.
*
* @param hgap
* the horizontal gap for the new {@link BorderLayout}
* @param vgap
* the vertical gap for the new {@link BorderLayout}
* @return the current builder instance
* @see JPanel#setLayout(LayoutManager)
* @see BorderLayout#BorderLayout(int, int)
*/
public PanelBuilder borderLayout(final int hgap, final int vgap)
{
return layout(new BorderLayout(hgap, vgap));
}
/**
* Applies a new {@link BoxLayout} to the currently built {@link JPanel}.
*
* @param axis
* the axis settings to apply on the new {@link BoxLayout}
* @return the current builder instance
* @see JPanel#setLayout(LayoutManager)
* @see BoxLayout#BoxLayout(java.awt.Container, int)
*/
public PanelBuilder boxLayout(final int axis)
{
return layout(new BoxLayout(get(), axis));
}
/**
* Applies a new {@link BoxLayout} in {@link BoxLayout#X_AXIS} mode to the
* currently built {@link JPanel}.
*
* @return the current builder instance
* @see JPanel#setLayout(LayoutManager)
* @see BoxLayout#BoxLayout(java.awt.Container, int)
* @see BoxLayout#X_AXIS
*/
public PanelBuilder boxLayoutX()
{
return layout(new BoxLayout(get(), BoxLayout.X_AXIS));
}
/**
* Applies a new {@link BoxLayout} in {@link BoxLayout#Y_AXIS} mode to the
* currently built {@link JPanel}.
*
* @return the current builder instance
* @see JPanel#setLayout(LayoutManager)
* @see BoxLayout#BoxLayout(java.awt.Container, int)
* @see BoxLayout#Y_AXIS
*/
public PanelBuilder boxLayoutY()
{
return layout(new BoxLayout(get(), BoxLayout.Y_AXIS));
}
/**
* Applies a new {@link BoxLayout} in {@link BoxLayout#LINE_AXIS} mode to the
* currently built {@link JPanel}.
*
* @return the current builder instance
* @see JPanel#setLayout(LayoutManager)
* @see BoxLayout#BoxLayout(java.awt.Container, int)
* @see BoxLayout#LINE_AXIS
*/
public PanelBuilder boxLayoutLine()
{
return layout(new BoxLayout(get(), BoxLayout.LINE_AXIS));
}
/**
* Applies a new {@link BoxLayout} in {@link BoxLayout#PAGE_AXIS} mode to the
* currently built {@link JPanel}.
*
* @return the current builder instance
* @see JPanel#setLayout(LayoutManager)
* @see BoxLayout#BoxLayout(java.awt.Container, int)
* @see BoxLayout#PAGE_AXIS
*/
public PanelBuilder boxLayoutPage()
{
return layout(new BoxLayout(get(), BoxLayout.PAGE_AXIS));
}
/**
* Applies a new {@link FlowLayout} to the currently built {@link JPanel}.
*
* @return the current builder instance
* @see JPanel#setLayout(LayoutManager)
* @see FlowLayout#FlowLayout()
*/
public PanelBuilder flowLayout()
{
return layout(new FlowLayout());
}
/**
* Applies a new {@link FlowLayout} to the currently built {@link JPanel}.
*
* @param align
* the alignment for the new {@link FlowLayout}
* @return the current builder instance
* @see JPanel#setLayout(LayoutManager)
* @see FlowLayout#FlowLayout(int)
*/
public PanelBuilder flowLayout(final int align)
{
return layout(new FlowLayout(align));
}
/**
* Applies a new {@link FlowLayout} to the currently built {@link JPanel}.
*
* @param align
* the alignment for the new {@link FlowLayout}
* @param hgap
* the horizontal gap for the new {@link FlowLayout}
* @param vgap
* the vertical gap for the new {@link FlowLayout}
* @return the current builder instance
* @see JPanel#setLayout(LayoutManager)
* @see FlowLayout#FlowLayout(int, int, int)
*/
public PanelBuilder flowLayout(final int align, final int hgap, final int vgap)
{
return layout(new FlowLayout(align, hgap, vgap));
}
/**
* Applies a new {@link GridBagLayout} to the currently built {@link JPanel}.
*
* @return the current builder instance
* @see JPanel#setLayout(LayoutManager)
* @see GridBagLayout#GridBagLayout()
*/
public PanelBuilder gridBagLayout()
{
return layout(new GridBagLayout());
}
/**
* Applies a new {@link GridLayout} to the currently built {@link JPanel}.
*
* @return the current builder instance
* @see JPanel#setLayout(LayoutManager)
* @see GridLayout#GridLayout()
*/
public PanelBuilder gridLayout()
{
return layout(new GridLayout());
}
/**
* Applies a new {@link GridLayout} to the currently built {@link JPanel}.
*
* @param rows
* the number of rows for the new {@link GridLayout}
* @param cols
* the number of columns for the new {@link GridLayout}
* @return the current builder instance
* @see JPanel#setLayout(LayoutManager)
* @see GridLayout#GridLayout(int, int)
*/
public PanelBuilder gridLayout(final int rows, final int cols)
{
return layout(new GridLayout(rows, cols));
}
/**
* Applies a new {@link GridLayout} to the currently built {@link JPanel}.
*
* @param rows
* the number of rows for the new {@link GridLayout}
* @param cols
* the number of columns for the new {@link GridLayout}
* @param hgap
* the horizontal gap for the new {@link GridLayout}
* @param vgap
* the vertical gap for the new {@link GridLayout}
* @return the current builder instance
* @see JPanel#setLayout(LayoutManager)
* @see GridLayout#GridLayout(int, int)
*/
public PanelBuilder gridLayout(final int rows, final int cols, final int hgap, final int vgap)
{
return layout(new GridLayout(rows, cols, hgap, vgap));
}
/**
* Adds a new given {@link JComponent} to the currently built {@link JPanel}
* and returns a {@link ComponentBuilder} for it.
*
* @param component
* the {@link JComponent} to add
* @param
* the concrete type of the {@link JComponent} to add
* @return the {@link ComponentBuilder} for the added {@link JComponent}
* @see JPanel#add(java.awt.Component)
*/
public ComponentBuilder> component(
final Component component)
{
get().add(component);
return new ComponentBuilder>(this, component);
}
/**
* Adds a new given {@link JComponent} to the currently built {@link JPanel}
* and returns a {@link ComponentBuilder} for it.
*
* @param component
* the {@link JComponent} to add
* @param constraints
* the constraints to apply upon adding
* @param
* the concrete type of the {@link JComponent} to add
* @return the {@link ComponentBuilder} for the added {@link JComponent}
* @see JPanel#add(java.awt.Component, Object)
*/
public ComponentBuilder> component(
final Component component,
final Object constraints)
{
get().add(component, constraints);
return new ComponentBuilder>(this, component);
}
/**
* Adds a new given {@link JComponent} to the currently built {@link JPanel}
* and returns a {@link ComponentBuilder} for it.
*
* @param component
* the {@link JComponent} to add
* @param constraints
* the constraints to apply upon adding
* @param index
* the index where to add the new {@link JComponent} within the
* current {@link JPanel}
* @param
* the concrete type of the {@link JComponent} to add
* @return the {@link ComponentBuilder} for the added {@link JComponent}
* @see JPanel#add(java.awt.Component, Object, int)
*/
public ComponentBuilder> component(
final Component component,
final Object constraints, final int index)
{
get().add(component, constraints, index);
return new ComponentBuilder>(this, component);
}
/**
* Adds a new given {@link JComponent} to the currently built {@link JPanel}
* and returns a {@link ComponentBuilder} for it.
*
* @param component
* the {@link JComponent} to add
* @param index
* the index where to add the new {@link JComponent} within the
* current {@link JPanel}
* @param
* the concrete type of the {@link JComponent} to add
* @return the {@link ComponentBuilder} for the added {@link JComponent}
* @see JPanel#add(java.awt.Component, int)
*/
public ComponentBuilder> component(
final Component component, final int index)
{
get().add(component, index);
return new ComponentBuilder>(this, component);
}
/**
* Add a new {@link JButton} to the currently built {@link JPanel} and
* returns a {@link ButtonBuilder} for it.
*
* @param text
* the label that the new {@link JButton} should have
* @return the {@link ButtonBuilder} for the added {@link JButton}
* @see JPanel#add(java.awt.Component)
* @see JButton#JButton(String)
*/
public ButtonBuilder> button(final String text)
{
final JButton button = new JButton(text);
get().add(button);
return new ButtonBuilder>(this, button);
}
/**
* Add a new {@link JButton} to the currently built {@link JPanel} and
* returns a {@link ButtonBuilder} for it.
*
* @param action
* the {@link Action} to apply for the new {@link JButton}
* @return the {@link ButtonBuilder} for the added {@link JButton}
* @see JPanel#add(java.awt.Component)
* @see JButton#JButton(Action)
*/
public ButtonBuilder> button(final Action action)
{
final JButton button = new JButton(action);
get().add(button);
return new ButtonBuilder>(this, button);
}
/**
* Add a new {@link JButton} to the currently built {@link JPanel} and
* returns a {@link ButtonBuilder} for it.
*
* @param text
* the label that the new {@link JButton} should have
* @param constraints
* the constraints to apply upon adding
* @return the {@link ButtonBuilder} for the added {@link JButton}
* @see JPanel#add(java.awt.Component, Object)
* @see JButton#JButton(String)
*/
public ButtonBuilder> button(final String text, final Object constraints)
{
final JButton button = new JButton(text);
get().add(button, constraints);
return new ButtonBuilder>(this, button);
}
/**
* Add a new {@link JButton} to the currently built {@link JPanel} and
* returns a {@link ButtonBuilder} for it.
*
* @param action
* the {@link Action} to apply for the new {@link JButton}
* @param constraints
* the constraints to apply upon adding
* @return the {@link ButtonBuilder} for the added {@link JButton}
* @see JPanel#add(java.awt.Component, Object)
* @see JButton#JButton(Action)
*/
public ButtonBuilder> button(final Action action, final Object constraints)
{
final JButton button = new JButton(action);
get().add(button, constraints);
return new ButtonBuilder>(this, button);
}
/**
* Add a new {@link JButton} to the currently built {@link JPanel} and
* returns a {@link ButtonBuilder} for it.
*
* @param text
* the label that the new {@link JButton} should have
* @param constraints
* the constraints to apply upon adding
* @param index
* the index where to add the new {@link JButton} within the
* current {@link JPanel}
* @return the {@link ButtonBuilder} for the added {@link JButton}
* @see JPanel#add(java.awt.Component, Object, int)
* @see JButton#JButton(String)
*/
public ButtonBuilder> button(final String text, final Object constraints, final int index)
{
final JButton button = new JButton(text);
get().add(button, constraints, index);
return new ButtonBuilder>(this, button);
}
/**
* Add a new {@link JButton} to the currently built {@link JPanel} and
* returns a {@link ButtonBuilder} for it.
*
* @param action
* the {@link Action} to apply for the new {@link JButton}
* @param constraints
* the constraints to apply upon adding
* @param index
* the index where to add the new {@link JButton} within the
* current {@link JPanel}
* @return the {@link ButtonBuilder} for the added {@link JButton}
* @see JPanel#add(java.awt.Component, Object, int)
* @see JButton#JButton(Action)
*/
public ButtonBuilder> button(final Action action, final Object constraints, final int index)
{
final JButton button = new JButton(action);
get().add(button, constraints, index);
return new ButtonBuilder>(this, button);
}
/**
* Add a new {@link JButton} to the currently built {@link JPanel} and
* returns a {@link ButtonBuilder} for it.
*
* @param text
* the label that the new {@link JButton} should have
* @param index
* the index where to add the new {@link JButton} within the
* current {@link JPanel}
* @return the {@link ButtonBuilder} for the added {@link JButton}
* @see JPanel#add(java.awt.Component, int)
* @see JButton#JButton(String)
*/
public ButtonBuilder> button(final String text, final int index)
{
final JButton button = new JButton(text);
get().add(button, index);
return new ButtonBuilder>(this, button);
}
/**
* Add a new {@link JButton} to the currently built {@link JPanel} and
* returns a {@link ButtonBuilder} for it.
*
* @param action
* the {@link Action} to apply for the new {@link JButton}
* @param index
* the index where to add the new {@link JButton} within the
* current {@link JPanel}
* @return the {@link ButtonBuilder} for the added {@link JButton}
* @see JPanel#add(java.awt.Component, int)
* @see JButton#JButton(Action)
*/
public ButtonBuilder> button(final Action action, final int index)
{
final JButton button = new JButton(action);
get().add(button, index);
return new ButtonBuilder>(this, button);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy