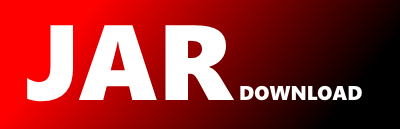
net.sf.javagimmicks.swing.model.DependencyComboBoxModelBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gimmicks Show documentation
Show all versions of gimmicks Show documentation
Utility classes, APIs and tools for Java
package net.sf.javagimmicks.swing.model;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Stack;
import java.util.Vector;
import javax.swing.ComboBoxModel;
import javax.swing.DefaultComboBoxModel;
import net.sf.javagimmicks.swing.model.DependencyComboBoxModel.CompositeKey;
/**
* A fluent API builder for creating a hierarchy of inter-depending
* {@link DependencyComboBoxModel}s.
*
* The fluent API is very simple: {@link #add(Object)} adds an element to the
* currently built {@link ComboBoxModel}, {@link #children()} internally
* switches down one level and allows to specify elements for a depending
* {@link ComboBoxModel} (and so on) until a call to {@link #parent()} which
* comes back to the original level and allows to specify more elements there.
* The resulting {@link List} of {@link ComboBoxModel}s can finally be retrieved
* via {@link #buildModels()}.
*
* For a better understanding, please have a look at this example from the test
* code of this class:
*
*
* // @formatter:off
* new DependencyComboBoxModelBuilder()
* .add("A").children()
* .add("A1").children()
* .add("A1x")
* .add("A1y")
* .add("A1z").parent()
* .add("A2").children()
* .add("A2x")
* .add("A2y")
* .add("A2z").parent()
* .add("A3").children()
* .add("A3x")
* .add("A3y")
* .add("A3z").parent()
* .add("A4").children()
* .add("A4x")
* .add("A4y")
* .add("A4z").parent().parent()
*
* .add("B").children()
* .add("B1").children()
* .add("B1x")
* .add("B1y")
* .add("B1z").parent()
* .add("B2").children()
* .add("B2x")
* .add("B2y")
* .add("B2z").parent().parent()
*
* .add("C").children()
* .add("C1").children()
* .add("C1x")
* .add("C1y")
* .add("C1z").parent()
* .add("C2").children()
* .add("C2x")
* .add("C2y")
* .add("C2z").parent()
* .add("C3").children()
* .add("C3x")
* .add("C3y")
* .add("C3z").parent().parent()
*
* .buildModels();
* // @formatter:on
*
*/
public class DependencyComboBoxModelBuilder
{
private Map> _modelData = new HashMap>();
private Stack
© 2015 - 2025 Weber Informatics LLC | Privacy Policy