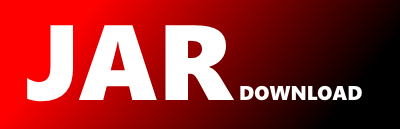
net.sf.javagimmicks.util.ComparableWrapper Maven / Gradle / Ivy
Go to download
[Jenkins](http://jenkins-ci.org/) extendable open source continuous integration server using OpenShift Jenkins image
Please wait ...