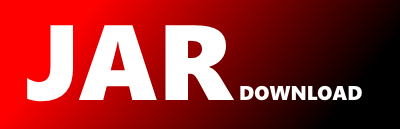
au.com.sparxsystems.Attribute Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.5-b10
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2013.09.13 at 12:06:48 PM EST
//
package au.com.sparxsystems;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.namespace.QName;
/**
* Java class for Attribute complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Attribute">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <choice>
* <element name="properties" type="{http://www.sparxsystems.com.au/ext}AttributeProperties"/>
* <element name="tags" type="{http://www.sparxsystems.com.au/ext}Tag" maxOccurs="unbounded" minOccurs="0"/>
* <element name="style" type="{http://www.sparxsystems.com.au/ext}AttributeStyle"/>
* <element name="documentation" type="{http://www.sparxsystems.com.au/ext}Documentation"/>
* <element name="model" type="{http://www.sparxsystems.com.au/ext}Model"/>
* <element name="stereotype" type="{http://www.sparxsystems.com.au/ext}Stereotype"/>
* </choice>
* <attGroup ref="{http://www.sparxsystems.com.au/ext}ObjectAttribs"/>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Attribute", propOrder = {
"properties",
"tags",
"style",
"documentation",
"model",
"stereotype"
})
public class Attribute {
protected AttributeProperties properties;
protected List tags;
protected AttributeStyle style;
protected Documentation documentation;
protected Model model;
protected Stereotype stereotype;
@XmlAttribute(name = "idref", namespace = "http://www.sparxsystems.com.au/ext")
protected QName idref;
@XmlAttribute(name = "type", namespace = "http://www.sparxsystems.com.au/ext")
protected QName type;
@XmlAttribute(name = "name")
@XmlSchemaType(name = "anySimpleType")
protected String name;
@XmlAttribute(name = "scope")
@XmlSchemaType(name = "anySimpleType")
protected String scope;
/**
* Gets the value of the properties property.
*
* @return
* possible object is
* {@link AttributeProperties }
*
*/
public AttributeProperties getProperties() {
return properties;
}
/**
* Sets the value of the properties property.
*
* @param value
* allowed object is
* {@link AttributeProperties }
*
*/
public void setProperties(AttributeProperties value) {
this.properties = value;
}
/**
* Gets the value of the tags property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the tags property.
*
*
* For example, to add a new item, do as follows:
*
* getTags().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Tag }
*
*
*/
public List getTags() {
if (tags == null) {
tags = new ArrayList();
}
return this.tags;
}
/**
* Gets the value of the style property.
*
* @return
* possible object is
* {@link AttributeStyle }
*
*/
public AttributeStyle getStyle() {
return style;
}
/**
* Sets the value of the style property.
*
* @param value
* allowed object is
* {@link AttributeStyle }
*
*/
public void setStyle(AttributeStyle value) {
this.style = value;
}
/**
* Gets the value of the documentation property.
*
* @return
* possible object is
* {@link Documentation }
*
*/
public Documentation getDocumentation() {
return documentation;
}
/**
* Sets the value of the documentation property.
*
* @param value
* allowed object is
* {@link Documentation }
*
*/
public void setDocumentation(Documentation value) {
this.documentation = value;
}
/**
* Gets the value of the model property.
*
* @return
* possible object is
* {@link Model }
*
*/
public Model getModel() {
return model;
}
/**
* Sets the value of the model property.
*
* @param value
* allowed object is
* {@link Model }
*
*/
public void setModel(Model value) {
this.model = value;
}
/**
* Gets the value of the stereotype property.
*
* @return
* possible object is
* {@link Stereotype }
*
*/
public Stereotype getStereotype() {
return stereotype;
}
/**
* Sets the value of the stereotype property.
*
* @param value
* allowed object is
* {@link Stereotype }
*
*/
public void setStereotype(Stereotype value) {
this.stereotype = value;
}
/**
* Gets the value of the idref property.
*
* @return
* possible object is
* {@link QName }
*
*/
public QName getIdref() {
return idref;
}
/**
* Sets the value of the idref property.
*
* @param value
* allowed object is
* {@link QName }
*
*/
public void setIdref(QName value) {
this.idref = value;
}
/**
* Gets the value of the type property.
*
* @return
* possible object is
* {@link QName }
*
*/
public QName getType() {
return type;
}
/**
* Sets the value of the type property.
*
* @param value
* allowed object is
* {@link QName }
*
*/
public void setType(QName value) {
this.type = value;
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
this.name = value;
}
/**
* Gets the value of the scope property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getScope() {
return scope;
}
/**
* Sets the value of the scope property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setScope(String value) {
this.scope = value;
}
}