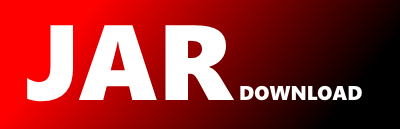
net.sf.jcc.model.parser.uml2.ModelElement Maven / Gradle / Ivy
package net.sf.jcc.model.parser.uml2;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import javax.xml.namespace.QName;
import au.com.sparxsystems.Association;
public class ModelElement
{
private String guid;
private Object element;
private Object extension;
private Map> references;
private List associations;
private String parentGuid;
public ModelElement(String guid, Object element)
{
this.guid = guid;
this.element = element;
references = new HashMap>();
}
public String getGuid()
{
return guid;
}
public Object getElement()
{
return element;
}
public void addReference(QName name, String ref)
{
List refs = references.get(name);
if (refs == null)
{
refs = new ArrayList();
references.put(name, refs);
}
refs.add(ref);
}
public Iterator references()
{
return references.keySet().iterator();
}
public String getReference(QName qname)
{
List refs = references.get(qname);
if (refs == null || refs.isEmpty())
{
return null;
}
return refs.get(0);
}
public List getReferences(QName qname)
{
return references.get(qname);
}
public Object getExtension()
{
return extension;
}
public void setExtension(Object extension)
{
this.extension = extension;
}
public List getAssociations()
{
return associations;
}
public void setAssociations(List associations)
{
this.associations = associations;
}
public void addAssocation(Association association)
{
if (associations == null)
{
associations = new ArrayList();
}
associations.add(association);
}
public String getParentGuid()
{
return parentGuid;
}
public void setParentGuid(String parentGuid)
{
this.parentGuid = parentGuid;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy