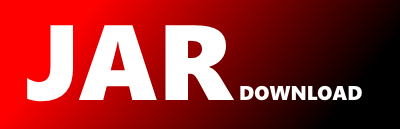
org.omg.uml.Package Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.2.5-b10
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2013.09.13 at 12:06:48 PM EST
//
package org.omg.uml;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for Package complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Package">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="ownedElement" type="{}Element" maxOccurs="unbounded" minOccurs="0"/>
* <element name="ownedComment" type="{}Comment" maxOccurs="unbounded" minOccurs="0"/>
* <element name="name" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="qualifiedName" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="visibility" type="{}VisibilityKind" minOccurs="0"/>
* <element name="packageImport" type="{}PackageImport" maxOccurs="unbounded" minOccurs="0"/>
* <element name="ownedRule" type="{}Constraint" maxOccurs="unbounded" minOccurs="0"/>
* <element name="ownedMember" type="{}NamedElement" maxOccurs="unbounded" minOccurs="0"/>
* <element name="member" type="{}NamedElement" maxOccurs="unbounded" minOccurs="0"/>
* <element name="importedMember" type="{}PackageableElement" maxOccurs="unbounded" minOccurs="0"/>
* <element name="elementImport" type="{}ElementImport" maxOccurs="unbounded" minOccurs="0"/>
* <element name="URI" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="packagedElement" type="{}PackageableElement" maxOccurs="unbounded" minOccurs="0"/>
* <element name="packageMerge" type="{}PackageMerge" maxOccurs="unbounded" minOccurs="0"/>
* <element name="ownedType" type="{}Type" maxOccurs="unbounded" minOccurs="0"/>
* <element name="nestedPackage" type="{}Package" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Package", propOrder = {
"ownedElement",
"ownedComment",
"name",
"qualifiedName",
"visibility",
"packageImport",
"ownedRule",
"ownedMember",
"member",
"importedMember",
"elementImport",
"uri",
"packagedElement",
"packageMerge",
"ownedType",
"nestedPackage"
})
public class Package {
protected List ownedElement;
protected List ownedComment;
protected String name;
protected String qualifiedName;
protected VisibilityKind visibility;
protected List packageImport;
protected List ownedRule;
protected List ownedMember;
protected List member;
protected List importedMember;
protected List elementImport;
@XmlElement(name = "URI")
protected String uri;
protected List packagedElement;
protected List packageMerge;
protected List ownedType;
protected List nestedPackage;
/**
* Gets the value of the ownedElement property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the ownedElement property.
*
*
* For example, to add a new item, do as follows:
*
* getOwnedElement().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Element }
*
*
*/
public List getOwnedElement() {
if (ownedElement == null) {
ownedElement = new ArrayList();
}
return this.ownedElement;
}
/**
* Gets the value of the ownedComment property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the ownedComment property.
*
*
* For example, to add a new item, do as follows:
*
* getOwnedComment().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Comment }
*
*
*/
public List getOwnedComment() {
if (ownedComment == null) {
ownedComment = new ArrayList();
}
return this.ownedComment;
}
/**
* Gets the value of the name property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getName() {
return name;
}
/**
* Sets the value of the name property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setName(String value) {
this.name = value;
}
/**
* Gets the value of the qualifiedName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getQualifiedName() {
return qualifiedName;
}
/**
* Sets the value of the qualifiedName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setQualifiedName(String value) {
this.qualifiedName = value;
}
/**
* Gets the value of the visibility property.
*
* @return
* possible object is
* {@link VisibilityKind }
*
*/
public VisibilityKind getVisibility() {
return visibility;
}
/**
* Sets the value of the visibility property.
*
* @param value
* allowed object is
* {@link VisibilityKind }
*
*/
public void setVisibility(VisibilityKind value) {
this.visibility = value;
}
/**
* Gets the value of the packageImport property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the packageImport property.
*
*
* For example, to add a new item, do as follows:
*
* getPackageImport().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PackageImport }
*
*
*/
public List getPackageImport() {
if (packageImport == null) {
packageImport = new ArrayList();
}
return this.packageImport;
}
/**
* Gets the value of the ownedRule property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the ownedRule property.
*
*
* For example, to add a new item, do as follows:
*
* getOwnedRule().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Constraint }
*
*
*/
public List getOwnedRule() {
if (ownedRule == null) {
ownedRule = new ArrayList();
}
return this.ownedRule;
}
/**
* Gets the value of the ownedMember property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the ownedMember property.
*
*
* For example, to add a new item, do as follows:
*
* getOwnedMember().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NamedElement }
*
*
*/
public List getOwnedMember() {
if (ownedMember == null) {
ownedMember = new ArrayList();
}
return this.ownedMember;
}
/**
* Gets the value of the member property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the member property.
*
*
* For example, to add a new item, do as follows:
*
* getMember().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link NamedElement }
*
*
*/
public List getMember() {
if (member == null) {
member = new ArrayList();
}
return this.member;
}
/**
* Gets the value of the importedMember property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the importedMember property.
*
*
* For example, to add a new item, do as follows:
*
* getImportedMember().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PackageableElement }
*
*
*/
public List getImportedMember() {
if (importedMember == null) {
importedMember = new ArrayList();
}
return this.importedMember;
}
/**
* Gets the value of the elementImport property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the elementImport property.
*
*
* For example, to add a new item, do as follows:
*
* getElementImport().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ElementImport }
*
*
*/
public List getElementImport() {
if (elementImport == null) {
elementImport = new ArrayList();
}
return this.elementImport;
}
/**
* Gets the value of the uri property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getURI() {
return uri;
}
/**
* Sets the value of the uri property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setURI(String value) {
this.uri = value;
}
/**
* Gets the value of the packagedElement property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the packagedElement property.
*
*
* For example, to add a new item, do as follows:
*
* getPackagedElement().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PackageableElement }
*
*
*/
public List getPackagedElement() {
if (packagedElement == null) {
packagedElement = new ArrayList();
}
return this.packagedElement;
}
/**
* Gets the value of the packageMerge property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the packageMerge property.
*
*
* For example, to add a new item, do as follows:
*
* getPackageMerge().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PackageMerge }
*
*
*/
public List getPackageMerge() {
if (packageMerge == null) {
packageMerge = new ArrayList();
}
return this.packageMerge;
}
/**
* Gets the value of the ownedType property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the ownedType property.
*
*
* For example, to add a new item, do as follows:
*
* getOwnedType().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Type }
*
*
*/
public List getOwnedType() {
if (ownedType == null) {
ownedType = new ArrayList();
}
return this.ownedType;
}
/**
* Gets the value of the nestedPackage property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the nestedPackage property.
*
*
* For example, to add a new item, do as follows:
*
* getNestedPackage().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Package }
*
*
*/
public List getNestedPackage() {
if (nestedPackage == null) {
nestedPackage = new ArrayList();
}
return this.nestedPackage;
}
}