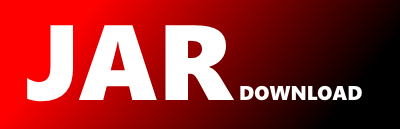
net.sf.javaprinciples.resource.Resource Maven / Gradle / Ivy
package net.sf.javaprinciples.resource;
import java.io.IOException;
import java.io.InputStream;
/**
* The Resource object encapsulates the content.
* It is flexible on whether the content is stored in memory or on disk.
* The resource support a window of time for when the resource is valid.
*
* @author wslade
* @param - resource type
*
*/
public interface Resource
{
/**
* A resource may be valid from a point in time.
* This typically will be the time the resource was created. It may not be the
* time the resource was first served.
* @return A time in milliseconds since 1970 relative to UTC.
*/
public long getValidFrom();
/**
* A resource may be valid only up to a point in time.
* This typically will be the time the resource may be updated.
* @return A time in milliseconds since 1970 relative to UTC.
*/
public long getValidUntil();
/**
* Each resource holds content of a specific type.
* @return The mime type of the content.
*/
public String getMimeType();
/**
* The resource may have a specific encoding.
* @return A standard encoding, may be null
*/
public String getEncoding();
/**
* The length of the resource in bytes
*/
public long getResourceLength();
/**
* Apply content to the resource.
* @param content May be the content itself or a reference such as a file url.
*/
public void setContent(T content);
/**
* Get content of the resource.
* @return content of resource.
*/
public T getContent();
/**
*
* @return A stream to the content. May be null if the content is no longer present.
* @throws IOException If a problem occurs opening the stream. Retrying wont help.
*/
InputStream getInputStream() throws IOException;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy