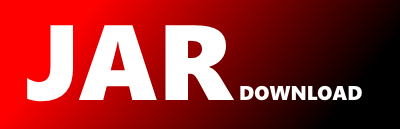
net.sf.javaprinciples.resource.servlet.ResourceModel Maven / Gradle / Ivy
package net.sf.javaprinciples.resource.servlet;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
import net.sf.javaprinciples.resource.Resource;
public class ResourceModel
{
private int statusCode;
private Map properties = new HashMap();
private Resource resource;
/**
* A code that will be set on the response.
* @param statusCode An Http status code or zero for dont care.
*/
public void setStatusCode(int statusCode)
{
this.statusCode = statusCode;
}
/**
* A status code that may have been optional set.
* @return Zero indicates no code set.
*/
public int getStatusCode()
{
return statusCode;
}
/**
* Add a property that would be returned in the header.
* @param name A header name
* @param value Any string value
*/
public void addProperty(String name, String value)
{
properties.put(name, value);
}
/**
* A method to iterator the properties.
* @return The key set that backs the properties. Never null.
*/
public Set getPropertyKeys()
{
return properties.keySet();
}
/**
* The property value for a key.
* @param key Hopefully a key that was returned in addProperty.
* @return Null if an invalid key is used.
*/
public String getProperty(String key)
{
return properties.get(key);
}
/**
* The resource that contains the data of interest.
* @param resource Must not be null.
*/
public void setResource(Resource resource)
{
this.resource = resource;
}
/**
* The resource previously set.
* @return May be null.
*/
public Resource getResource()
{
return resource;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy