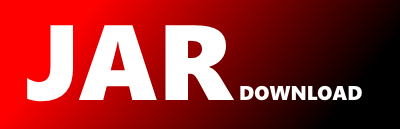
net.sf.javaprinciples.test.JsonObjectLoad Maven / Gradle / Ivy
The newest version!
package net.sf.javaprinciples.test;
import java.io.IOException;
import java.util.Iterator;
import org.springframework.core.io.Resource;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import net.sf.javaprinciples.core.UnexpectedException;
import net.sf.javaprinciples.persistence.BusinessObjectPersistence;
/**
* Test the reading of personal communique records.
* This test is only functional with a loaded JVI database.
*
* @author Warwick Slade
*/
public class JsonObjectLoad implements ObjectLoad
{
private BusinessObjectPersistence businessObjectPersistence;
private ObjectMapper mapper;
public void load(Resource resource)
{
JsonNode node = null;
try
{
node = mapper.readTree(resource.getURL());
}
catch (IOException e)
{
throw new UnexpectedException("Could not load resource", e);
}
int size = node.size();
Iterator stringIterator = node.fieldNames();
while (stringIterator.hasNext())
{
String name = stringIterator.next();
JsonNode child = node.get(name);
Class target;
try
{
target = Class.forName(name);
}
catch (ClassNotFoundException e)
{
throw new UnexpectedException("Class not found", e);
}
if (child.isArray())
{
for (int i = 0; i < child.size(); i++)
{
JsonNode element = child.get(i);
try
{
Object targetInstance = mapper.treeToValue(element, target);
businessObjectPersistence.storeObject(targetInstance);
}
catch (JsonProcessingException e)
{
throw new UnexpectedException("Problem processing json", e);
}
}
}
else
{
try
{
Object targetInstance = mapper.treeToValue(child, target);
businessObjectPersistence.storeObject(targetInstance);
}
catch (JsonProcessingException e)
{
throw new UnexpectedException("Problem processing json", e);
}
}
}
}
public void setBusinessObjectPersistence(BusinessObjectPersistence businessObjectPersistence)
{
this.businessObjectPersistence = businessObjectPersistence;
}
public void setMapper(ObjectMapper mapper)
{
this.mapper = mapper;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy