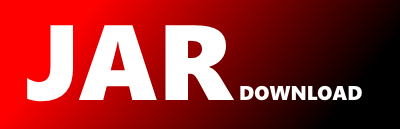
net.sf.jcmdlineparser.example.Example Maven / Gradle / Ivy
/**********************************************************************
Copyright (c) 2009-2010 Alexander Kerner. All rights reserved.
Licensed under the Apache License, Version 2.0 (the "License");
you may not use this file except in compliance with the License.
You may obtain a copy of the License at
http://www.apache.org/licenses/LICENSE-2.0
Unless required by applicable law or agreed to in writing, software
distributed under the License is distributed on an "AS IS" BASIS,
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
See the License for the specific language governing permissions and
limitations under the License.
***********************************************************************/
package net.sf.jcmdlineparser.example;
import java.util.Arrays;
import net.sf.jcmdlineparser.options.BooleanOption;
import net.sf.jcmdlineparser.options.NoValueGivenException;
import net.sf.jcmdlineparser.options.OptionBuilder;
import net.sf.jcmdlineparser.options.OptionClashException;
import net.sf.jcmdlineparser.options.StringMultiOption;
import net.sf.jcmdlineparser.options.StringSingleOption;
import net.sf.jcmdlineparser.parser.CmdLineParser;
import net.sf.jcmdlineparser.parser.CmdLineParserImpl;
/**
*
* Usage examples.
*
*
* @author Alexander Kerner
* @version 2010-08-10
*
*/
//START SNIPPET: example2
public class Example {
// your command line parser
private static final CmdLineParser parser = new CmdLineParserImpl();
public static void main(String[] args) {
try {
// Build all options you want to parse for
// Build a StringSingleOption to parse for a option, that provides
// one string as a value
final StringSingleOption h = new OptionBuilder()
.setShortIdentifier('h').setLongIdentifier("hans")
.setDescription("hans option").newStringSingleOption();
// Build a StringMultiOption to parse for a option, that provides a
// list of string values
final StringMultiOption m = new OptionBuilder().setRequired(true)
.setShortIdentifier('m').newStringMultiOption();
// Build a BooleanOption to parse for a boolean value
final BooleanOption b0 = new OptionBuilder()
.setDescription("the new pope is german!")
.setLongIdentifier("german-pope").newBooleanOption();
// Build another Boolean option
final BooleanOption b1 = new OptionBuilder()
.setDescription("the new pope is swedish!")
.setLongIdentifier("swedish-pope").newBooleanOption();
// since there can be only one pope, and this one can either be from
// germany or from sweden, we must exclude the two boolean options
// from each other
b0.addClashOption(b1);
// make option m require option h
m.addRequiredOption(h);
// register your options
parser.registerOption(h);
parser.registerOption(m);
parser.registerOption(b0);
parser.registerOption(b1);
// finally start parsing
args = parser.parse(args);
// print a help, just to see how it looks
// c.printHelp("usage:");
// print out all options from command line that could not be
// recognized
System.out.println("unknown options: \"" + Arrays.asList(args)
+ "\"");
// finally lets see what we got:
// start the program with following command line:
//
// --german-pope=true -m=rambo,john -h=john-rambo
//
// play around a bit and see what happens!
System.out.println("parsed options:");
if (h.isSet())
System.out.println(h + ": \"" + h.getValue() + "\"");
if (m.isSet())
System.out.println(m + ": \"" + m.getValues() + "\"");
if (b0.isSet())
System.out.println(b0 + ": \"" + b0.getValue() + "\"");
if (b1.isSet())
System.out.println(b1 + ": \"" + b1.getValue() + "\"");
} catch (OptionClashException e) {
exitWithKnownError(
"the new pope cannot be both from germany and sweden!", e,
parser);
} catch (NoValueGivenException e) {
exitWithKnownError("missing value for option!", e, parser);
} catch (Exception e) {
exitWithUnknownError(e.getLocalizedMessage(), e, parser);
}
}
private static void exitWithKnownError(String message, Throwable t,
CmdLineParser parser) {
System.err.println(message);
if (t != null)
// t.printStackTrace();
if (parser != null)
parser.printHelp("Program usage:");
System.exit(1);
}
private static void exitWithUnknownError(String message, Throwable t,
CmdLineParser parser) {
System.err.println(message);
if (t != null)
t.printStackTrace();
if (parser != null)
parser.printHelp("Program usage:");
System.exit(2);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy